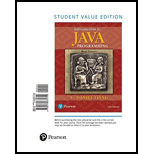
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17.4, Problem 17.4.7CP
Program Plan Intro
“writeDouble” method:
The “writeDouble” method is used to write double value to the output stream.
Example:
output.writeDouble(132.5);
“output” is an object created for “DataOutputStream()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a program that reads a stream of integers from a file and writes only the positive numbers to a second file. The user should be prompted to enter the names of both the input file and output file in main(), and then main() should attempt to open both files (providing an error if there is an error during this process). The main() method should then call the process() method to read all the integers from the input file and write only the positive integers to the output file. The process() method takes as arguments a Scanner to read from the input and a PrintWriter to write to the output. You can assume that if you are able to successfully open the input file, then there will only be integers in it.
Write program that reads a list of names from a data file and displays the names to the console. The program should prompt the user to enter a filename. Create such a file and check your code. Solve with python.
Example of code format:
#Prompt the user for file name
file_name = input("Enter file name : ")
#Open file
with open(file_name,"r") as file:
#Read file content
f = file.readline()
#split the contents into numbers
numbers = f.split(' ')
test_list = [int(i) for i in numbers]
res = 1
#Find the product
for i in test_list:
res*=i
#Print result
print("Product of numbers in the list :",res)
Write a java program that replace each line of a file with its reverse. For example, we have in file this data:
Hello
I am world!
Hello world), Smart.
-if you run the output is:
olleH
!dlrow ma I
.tramS,) dlrow olleH
-Of course, if you run reverse twice on the same file, you get back the original file.
Chapter 17 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 17.2 - What is a text file and what is a binary file? Can...Ch. 17.2 - How do you read or write text data in Java? What...Ch. 17.3 - Prob. 17.3.1CPCh. 17.3 - How is a Java character represented in the memory,...Ch. 17.3 - If you write the string ABC to an ASCII text file,...Ch. 17.3 - If you write the string 100 to an ASCII text file,...Ch. 17.3 - What is the encoding scheme for representing a...Ch. 17.4 - Prob. 17.4.1CPCh. 17.4 - Why should you always close streams? How do you...Ch. 17.4 - Prob. 17.4.3CP
Ch. 17.4 - Does FileInputStream/Fi1eOutputStream introduce...Ch. 17.4 - What will happen if you attempt to create an input...Ch. 17.4 - How do you append data to an existing text file...Ch. 17.4 - Prob. 17.4.7CPCh. 17.4 - What is written to a file using writeByte(91) on a...Ch. 17.4 - How do you check the end of a file in an input...Ch. 17.4 - What is wrong in the following code? Import...Ch. 17.4 - Suppose you run the following program on Windows...Ch. 17.4 - After the following program is finished, how many...Ch. 17.4 - For each of the following statements on a...Ch. 17.4 - What are the advantages of using buffered streams?...Ch. 17.5 - How does the program check if a file already...Ch. 17.5 - How does the program detect the end of the file...Ch. 17.5 - How does the program count the number of bytes...Ch. 17.6 - Prob. 17.6.1CPCh. 17.6 - Prob. 17.6.2CPCh. 17.6 - Is it true that any instance of...Ch. 17.6 - Prob. 17.6.4CPCh. 17.6 - Prob. 17.6.5CPCh. 17.6 - What will happen when you attempt to run the...Ch. 17.7 - Can RandomAccessFi1e streams read and write a data...Ch. 17.7 - Create a RandomAccessFi1e stream for the file...Ch. 17.7 - What happens if the file test.dat does not exist...Ch. 17 - (Create a text file) Write a program to create a...Ch. 17 - (Create a binary data file) Write a program to...Ch. 17 - (Sum all the integers in a binary data file)...Ch. 17 - (Convert a text file into UTF) Write a program...Ch. 17 - Prob. 17.5PECh. 17 - Prob. 17.6PECh. 17 - Prob. 17.7PECh. 17 - (Update count) Suppose that you wish to track how...Ch. 17 - (Address book) Write a program that stores,...Ch. 17 - (Split files) Suppose you want to back up a huge...Ch. 17 - (Split files GUI) Rewrite Exercise 17.10 with a...Ch. 17 - Prob. 17.12PECh. 17 - (Combine files GUI) Rewrite Exercise 17.12 with a...Ch. 17 - (Encrypt files) Encode the file by adding 5 to...Ch. 17 - (Decrypt files) Suppose a file is encrypted using...Ch. 17 - (Frequency of characters) Write a program that...Ch. 17 - (BitOutputStream) Implement a class named...Ch. 17 - (View bits) Write the following method that...Ch. 17 - (View hex) Write a program that prompts the user...Ch. 17 - (Hex editor) Write a GUI application that lets the...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a program that reads the strings from file SortedStrings. txt and report whether the strings in the files are stored in ascending order. If the strings are not sorted in the file, display the first two strings that are out of order.arrow_forwardHow to write on a file more than one data at a time using loops in java?arrow_forwardWrite a program in python IDLE that allows the user to navigate the lines of text in a file. The program should prompt the user for a filename and input the lines of text into a list. The program then enters a loop in which it prints the number of lines in the file and prompts the user for a line number. Actual line numbers range from 1 to the number of lines in the file. If the input is 0, the program quits. Otherwise, the program prints the line associated with that number.arrow_forward
- Write a program in MIPS that does the following: Request the name of a file from the user and open it. If it will not open (for example, the file does not exist) print an error message and exit. Read a block of text from a file Break the line into words. The lines in the file may contain spaces, commas, or periods, colons, and semicolons that separate words. Look up the word in your list. If it is not in the list, add the word to the list and add a count of 1. If it is in the list, increment the count for that word. Once all the words in the block of text have been counted, return for another line. When you reach the end of the file, print the list of all the words and their counts, one word per line, then exit.arrow_forwardwrite a program with python that asks the user to enter two filenames. One filecontains a list of words that you want to count. For the following explanation, let’s call it words.txt.(When your program runs, the user could type a different name. Do not hardcode any filenames inyour code.) The second file contains the text that you want to search for each word. For theexplanation, let’s call it story.txt.Ask the user to enter the name of each file. Assume that the files are located in the same directoryas your code file.For each word in words.txt, search through story.txt and count how many times the word occurs.Print a summary similar to the sample output below.Note 1: You should consider a “word” to be anything bounded by whitespace. You do not need todo any processing to account for punctuation or other special characters. For example, in thesample below, “time.” (with a period) is not considered the same as the word “time” (without aperiod). Capitalization will also matter. “The”…arrow_forwardI have managed to get my random number generator working. The range of the random number and how many random numbers are read in from a file and I can read them with no problem The generated random numbers are written to a file with the sum of those numbers. My issue is that everytime only the first line is correct, the following lines have the correct random numbers but the sum is totally wrongarrow_forward
- Suppose you are given a text file that contains the names of people. Every name in the file consists of a first name and last name. Unfortunately, the programmer that created the file of names did not guarantee that each name was on a single line of the file. Read this file of names and write them to a new text file sorted according to first name, one name per line. For example, if the input file contains Ed Marston Bob Jones Jeff Williams Fred Charles The output file should be Bob Jones Ed Marston Fred Charles Jeff Williams Use arrays to solve the problem.arrow_forwardWrite a python program that has the following functionality:1. read input from a file containing a list of words (each word is written on its own line) and store the data (strings) in a listHint: try using the readline() function or a for loop2. randomly select a string from the list3. output / write the selected string to a new filearrow_forwardThe history teacher at your school needs help in grading a True/False test. The students’ IDs and test answers are stored in a file. The first entry in the file contains answers to the test in the form:TFFTFFTTTTFFTFTFTFTTEvery other entry in the file is the student ID, followed by a blank, followed by the student’s responses. For example, the entry:ABC54301 TFTFTFTT TFTFTFFTTFTindicates that the student ID is ABC54301 and the answer to question 1 is True, the answer to question 2 is False, and so on. This student did not answer question 9. The exam has 20 questions, and the class has more than 150 students. Each correct answer is awarded two points, each wrong answer gets one point deducted, and no answer gets zero points. Write a program that processes the test data. The output should be the student’s ID, followed by the answers, followed by the test score, followed by the test grade. Assume the following grade scale:90%–100%, A; 80%–89.99%, B; 70%–79.99%, C; 60%–69.99%, D; and…arrow_forward
- Write a program that reads two input files whose lines are ordered by a key data field. Your program should merge these two files, writing an output file that contains all lines from both files ordered by the same key field. As an example, if two input files contain student names and grades for a particular class ordered by name (the key field), merge the information as shown below. File 1 and file 2 are supplied. Here is an algorithm to merge the data from two files: Read a line from each data file While the end of both files has not been reached While the end of both files has not been reached Write the line from file 1 to the output file and read a new line from file 1. Else Write the line from file 2 to the output file and read a new line from file 2. Write the remaining lines (if any) from file 1 to the output file. Write the remaining lines (if any) from file 2 to the output file. See the Merging Filesslides attachedto the project in Canvasfor a visual look at this algorithm.arrow_forwardconsider a program that reads data from a text file called: "klingon-english.txt" (shown below). How would one construct such a program with the following conditions: 1. Ask the user to choose a Klingon consonant they want to practice with. Ask again if the user’s answer is not a valid Klingon consonant, until the user enters a valid consonant. 2. Find a Klingon word that starts with the chosen consonant (the text file contains only one word that starts with any given consonant, so you don’t need to use the random library) 3. Ask the user to translate the chosen word into Klingon 4. Print "Correct" if the user’s answer is correct 5. Print "Sorry, you’re wrong!" if the user’s answer is wrong 6. Print The correct answer is ... if all three user’s answers are wrong You will also factor in this version: 7. If the answer is incorrect, show the first hint: the first and last characters of the correct Klingon word. When showing a hint, replace all other characters with a star (*) 8. If the…arrow_forwardSuppose that you have two text files that contain sequences of integers separated by white space (blank space, tabs, and line breaks). The integers in both files appear in sorted order, with smaller values near the beginning of the file and large values closer to the end. Write a pseudocode algorithm that merges the two sequences into a single sorted sequence that is written to a third file.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
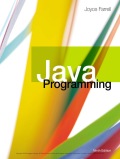
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT