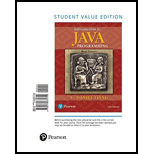
(BitOutputStream) Implement a class named BitOutputStream, as shown in Figure 17.22, for writing bits to an output stream. The writeBit (char bit) method stores the bit in a byte variable. When you create a BitOutputStream, the byte is empty. After invoking writeBit(‘1’), the byte becomes 00000001. After invoking writeBit (“0101”), the byte becomes 00010101. The first three bits are not filled yet. When a byte is full, it is sent to the output stream. Now the byte is reset to empty. You must close the stream by invoking the close() method. If the byte is neither empty nor full, the close() method first fills the zeros to make a full 8 bits in the byte and then outputs the byte and closes the stream. For a hint, see
FIGURE 17.22 BitOutputStream outputs a stream of bits to a file.

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
SURVEY OF OPERATING SYSTEMS
Database Concepts (8th Edition)
Concepts Of Programming Languages
- <<Write in Java>> - Challenge 7 File encryption is the science of writing the contents of a file in a secret code. Your encryption program should work like a filter, reading the contents of one file, modifying the data into a code, and then writing the coded contents out to a second file. The second file will be a version of the first file, but written in a secret code. Although there are complex encryption techniques, you should come up with a simple one of your own. For example, you could read the first file one character at a time, and add 10 to the character code of each character before it is written to the second file. - Challenge 8 Write a program that decrypts the file produced by the program in Programming Challenge 7. The decryption program should read the contents of the coded file, restore the data to its original state, and write it to another filearrow_forwardOperating system The free space bitmap looks like this:1010 0000 0000 0000:The system always searches for free blocks starting at the lowest numbered block. Block size is 4 KiB. (Blocks that belong to the same le do not need to be contiguous.) Write down the bitmap after each of the following actions:(a) File A of size 22 KiB is written.(b) File B of size 10 KiB is written.(c) File A is deleted.(d) File C of size 29 KiB is written.(e) File B is deleted.arrow_forwardComputer Science Write a program that processes a data sequence according to the following specifications: The sequence has three kinds of positive numbers: red, black and white. Each red number is preceded by the letter r, each black number is preceded by the letter b and each white number is preceded by the letter w. The number 0 indicates the end of data. If a number is preceded by any character other than r, b or w, the number is ignored. For instance, if the input to the program is: r 23 b 15 b 11 w 17 c 13 r 19 b 0, then we have two red numbers, 23 and 19, two black numbers 15 and 11, and one white number, 17. The number 13 is ignored. (The terminating 0 is also a black number but it is not processed.) The program produces the following output: The sum of red numbers is 42The sum of black numbers is 26The sum of white numbers is 17 Assume that input is from the keyboard and is in the prescribed format. (a). In 2-3 sentences, describe your strategy for solving this…arrow_forward
- Did I correctly do this function? Did I correctly do the precondition and postcondition? //Precondition: flag_position is a non-negative integer and hBit_flags is a handle to a valid Bit_flags object.//Postcondition: returns the value of the flag at index flag_position if it is in bounds or -1 otherwise.int bit_flags_check_flag(BIT_FLAGS hBit_flags, int flag_position){Bit_flags* pBit_flags = (Bit_flags*)hBit_flags;int i = flag_position / 32;flag_position %= 32;return ((1 << flag_position) & (pBit_flags->data[i])) != 0;if (flag_position <= pBit_flags->capacity) { return *pBit_flags->data;}else{ return -1;}}arrow_forwardLanguage: JAVA Problem 1: Decimal to Binary Conversion Write a program that takes an integer value as an input and converts that value to its binary representation; for instance, if the user inputs 17, then the output will be 10001. Do not forget to check for valid input, which means if the user inputs a type of data other than an integer re-prompt the user to enter a valid value. The output binary number can be a string. Sample input and output: Enter an integer > a You entered an invalid type. Try again. Enter an integer > 17 10001arrow_forward9. to read data from data reader (dr1) just one time,, we write the following statement:- dr1.read(1) dr1.read() dr1.executereader nonearrow_forward
- java pleasearrow_forwardWrite a Java programarrow_forwardLab Assignment 1: Vigenere Cipher Overview: You are required to implement the Vigenere Cipher using any programming language of your choice. Your program should be able to encrypt any given message written in text. Your program should allow the user to submit a message for encryption (the message must use text only). You should use the following message to demonstrate the encryption: “Party on the Quad." Your program should allow the user to input the key for performing the encryption. Please use the following key to demonstrate the encryption process: "Homecoming."arrow_forward
- Write a Program in C Language.arrow_forwardAssignment for Computer Architecture You are to write a program in MIPS that computes N! using a loop. Remember N! is the product of all the numbers from 1 to N inclusive, that is 1 x 2 x 3 x (N – 1) x N. It is defined as 1 for N = 0 and is undefined for values less than 0. The program first requests the user to input the value of N (display a prompt first so the user knows what to do). If the input value is less than 0, the program is to display “N! undefined for values less than 0” and request input again. If the value input is non-negative, it is to compute N! using a loop. You are to have your name, the assignment number, and a brief description of the program in comments at the top of your program. Since this is an assembly language program, I expect to see comments on almost every line of code in the program. Also make the code neat (line up the commands and comments in nice columns)arrow_forwardNetworking, TCP/IP requires one computer to function as the server and another to function as the client. The two computers must communicate using the same “language”. You will be building the server and the client. Write the program in C The Server Must accept commands in the form: PUSH “filename” fileSize <<<bytes>>> PUSH means that the client will be sending you a file. You need to store it with the filename sent. You must expect and require fileSize bytes. Respond to the client with “OK”. PULL “filename” PULL means that the client wants this file back. Respond with the number of bytes (use stat()), and then send all of the bytes to the client. Then respond with “OK” (a different send()). The server should take two parameters – the directory to work in and the port. The server should not shutdown. The server must have an accept() loop to allow multiple clients to connect (one at a time).arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
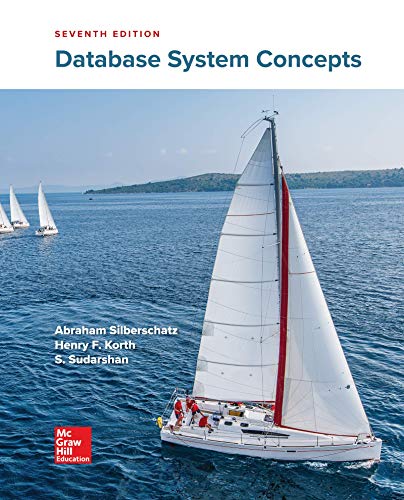
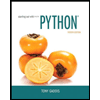
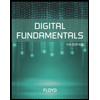
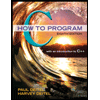
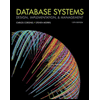
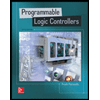