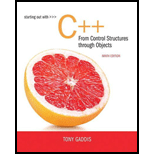
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 17.3, Problem 17.15CP
What happens when you use an invalid index with the
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
in C++ mathematical functions language
Consider the function
void modify(int & x)
{
x = 10;
}Show how to call the modify function so that it sets the integer int i;to 10.
in C++
Write a function that allows you to modify a Planet in the SolarSystem class. You can choose to pass a Planet into this function, or have the function prompt for planet information. Either way, you need to be able to specify the Planet at a specific index to be modified.
This is an example of how it could potentially look in the main:
// code that declared a SolarSystem the_system// and assigned planetsPlanet p("X", 2000, 4000);int index = 2;the_system.changePlanet(p, index);
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 17.2 - Prob. 17.1CPCh. 17.2 - Prob. 17.2CPCh. 17.2 - Prob. 17.3CPCh. 17.2 - Suppose you are writing a program that uses the...Ch. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - What does a containers begin() and end() member...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17.3 - Write a statement that defines an empty vector...Ch. 17.3 - Prob. 17.12CPCh. 17.3 - Prob. 17.13CPCh. 17.3 - Write a statement that defines a vector object...Ch. 17.3 - What happens when you use an invalid index with...Ch. 17.3 - Prob. 17.16CPCh. 17.3 - If your program will be added a lot of objects to...Ch. 17.3 - Prob. 17.18CPCh. 17.3 - Prob. 17.19CPCh. 17.4 - Prob. 17.20CPCh. 17.4 - Write a statement that defines a nap named myMap....Ch. 17.4 - Prob. 17.22CPCh. 17.4 - Prob. 17.23CPCh. 17.4 - Prob. 17.24CPCh. 17.4 - Prob. 17.25CPCh. 17.4 - Prob. 17.26CPCh. 17.4 - Prob. 17.27CPCh. 17.5 - What are two differences between a set and a...Ch. 17.5 - Write a statement that defines an empty set object...Ch. 17.5 - Prob. 17.30CPCh. 17.5 - Prob. 17.31CPCh. 17.5 - Prob. 17.32CPCh. 17.5 - If you store objects of a class that you have...Ch. 17.5 - Prob. 17.34CPCh. 17.5 - Prob. 17.35CPCh. 17.6 - Prob. 17.36CPCh. 17.6 - What value will be stored in v[0] after the...Ch. 17.6 - Prob. 17.38CPCh. 17.6 - Prob. 17.39CPCh. 17.6 - Prob. 17.40CPCh. 17.6 - Prob. 17.41CPCh. 17.6 - Prob. 17.42CPCh. 17.7 - Prob. 17.43CPCh. 17.7 - Which operator must be overloaded in a class...Ch. 17.7 - Prob. 17.45CPCh. 17.7 - What is a predicate?Ch. 17.7 - Prob. 17.47CPCh. 17.7 - Prob. 17.48CPCh. 17.7 - Prob. 17.49CPCh. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - How does the behavior of the equal_range() member...Ch. 17 - Prob. 12RQECh. 17 - When using one of the STL algorithm function...Ch. 17 - You have written a class, and you plan to store...Ch. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - A(n) ___________ container stores its data in a...Ch. 17 - _____________ are pointer-like objects used to...Ch. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - The _____ class is an associative container that...Ch. 17 - Prob. 27RQECh. 17 - Prob. 28RQECh. 17 - A _______ object is an object that can be called,...Ch. 17 - A _________ is a function or function object that...Ch. 17 - A ____________ is a predicate that takes one...Ch. 17 - A __________ is a predicate that takes two...Ch. 17 - A __________ is a compact way of creating a...Ch. 17 - T F The array class is a fixed-size container.Ch. 17 - T F The vector class is a fixed-size container.Ch. 17 - T F You use the operator to dereference an...Ch. 17 - T F You can use the ++ operator to increment an...Ch. 17 - Prob. 38RQECh. 17 - Prob. 39RQECh. 17 - T F You do not have to declare the size of a...Ch. 17 - T F A vector uses an array internally to store its...Ch. 17 - Prob. 42RQECh. 17 - T F You can store duplicate keys in a map...Ch. 17 - T F The multimap classs erase() member function...Ch. 17 - Prob. 45RQECh. 17 - Prob. 46RQECh. 17 - Prob. 47RQECh. 17 - Prob. 48RQECh. 17 - T F If two iterators denote a range of elements...Ch. 17 - T F You must sort a range of elements before...Ch. 17 - T F Any class that will be used to create function...Ch. 17 - T F Writing a lambda expression usually requires...Ch. 17 - T F You can assign a lambda expression to a...Ch. 17 - Prob. 54RQECh. 17 - Write a statement that defines an iterator that...Ch. 17 - Prob. 56RQECh. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 58RQECh. 17 - Prob. 59RQECh. 17 - The following code defines a vector and an...Ch. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 62RQECh. 17 - Prob. 63RQECh. 17 - Prob. 64RQECh. 17 - Look at the following vector definition: vectorint...Ch. 17 - Write a declaration for a class named Display. The...Ch. 17 - Write code that docs the following: Uses a lambda...Ch. 17 - // This code has an error. arrayint, 5 a; a[5] =...Ch. 17 - // This code has an error. vectorstring strv =...Ch. 17 - // This code has an error. vectorint numbers(10);...Ch. 17 - // This code has an error. vectorint numbers ={1,...Ch. 17 - Prob. 72RQECh. 17 - Prob. 73RQECh. 17 - // This code has an error. vectorint v = {6, 5, 4,...Ch. 17 - // This code has an error. auto sum = ()[int a,...Ch. 17 - Unique Words Write a program that opens a...Ch. 17 - Course Information Write a program that creates a...Ch. 17 - Prob. 3PCCh. 17 - File Encryption and Decryption Write a program...Ch. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
How are relationships between tables expressed in a relational database?
Modern Database Management
Circumference and Area of a Circle) Heres a peek ahead. In this chapter, you learned about integers and the typ...
Java How To Program (Early Objects)
String Search Write a program that asks the user for a file name and a string for which to search. The program ...
Starting Out with C++ from Control Structures to Objects (8th Edition)
A file that contains a Flash animation uses the __________ file extension. a. .class b. .swf c. .mp3 d. .flash
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Answer Problem 13 using SQL. PROBLEM 13 13. Using the commands SELECT, PROJECT, and JOIN, write a sequence of i...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
What is a ToolTip?
Starting Out With Visual Basic (7th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- You may use the vector and iostream libraries. You are allowed to use three built-in vector member functions (and no others) but you may not have to use them all. The member functions you are allowed to use are size(), at(), and push_back() Write a function that outputs a vector of doubles with each number in the vector separated by a space then a newline after the entire vector is output. The whole vector output should be preceded by a single line saying "Current Vector Contents:". Write a function that takes a vector a doubles and reverses the order of all the elements of the vector. Write a function that fills a vector of doubles with positive numbers using the standard input stream cin, terminate the input when the user enters any negative number. A single output prompt should precede the initial input stating directions for user. Write a main function that creates an empty vector, calls functions from 2 & 3 and calls your output function before and after each of your calls…arrow_forwardINSTRUCTIONS: In this assignment, you will make your own smart pointer type. You will write the following class to develop a referenced counted smart pointer. You will also implement a few other member functions to resemble the functionality of an ordinary raw pointer. Basically, this is a problem of designing a single, non-trivial class and overloading a few pointer related operators. You may not use any of the STLs except for stdexcept, to do this, i.e., please dont try and use a shared_ptr to implement the class. You can start with this code, and you may add other member functions, if you want. C++ LANGUAGE Only need smart_ptr.h file.arrow_forwardProcedure: Develop an extension of structure.Vector, called MyVector, that includes a new method, sort. Here are some steps toward implementing this new class: 1. Create a new class, MyVector, which is declared to be an extension of the structure.Vector class. You should write a default constructor for this class that simply calls super();. This will force the structure.Vector constructor to be called. This, in turn, will initialize the protected fields of the Vector class. 2. Construct a new Vector method called sort. It should have the following declaration: public void sort(Comparator c) // pre: c is a valid comparator // post: sorts this vector in order determined by c This method uses a Comparator type object to actually perform a sort of the values in MyVector. You may use any sort that you like. 3. Write an application that reads in a data file with several fields, and, dependingarrow_forward
- In C++, define a “Conflict” function that accepts an array of Course object pointers. It will return two numbers:- the count of courses with conflicting days. If there is more than one course scheduled in the same day, it is considered a conflict. It will return if there is no conflict.- which day of the week has the most conflict. It will return 0 if there is none. Show how this function is being called and returning proper values. you may want to define a local integer array containing the count for each day of the week with the initial value of 0.Whenever you have a Course object with a specific day, you can increment that count for that corresponding index in the schedule array.arrow_forwardgetting error please helparrow_forwardIn C++, as an actual parameter, can an array be passed by value?arrow_forward
- Remove is a function that has been defined.arrow_forwardSmart Pointer: Write a smart pointer class. A smart pointer is a data type, usually implemented with templates, that simulates a pointer while also providing automatic garbage collection. It automatically counts the number of references to a SmartPointer<?> object and frees the object of type T when the reference count hits zero.arrow_forwardI would really appreciate it if you could solve it quickly. Thank you so much C++arrow_forward
- 03 Inheritance / Polymorphism Task 1 Currently, you are using composition by creating an instance of a vector / ArrayList inside your AddressBook. This works fine but if we really think about it, and AddressBook is a collection like a stack or a queue or a vector for that matter. Your task is to: C++ derive AddressBook from vector. In other words, use the vector as a base class and derive AddressBook from it. This means that you will need to remove the Vector from the private section of your class. You are going to have to make some fundamental changes to your AddressBook so that you are using the vector / ArrayList functionality to store and retrieve people. In other words, you should be accessing the ArrayList / vector using this. C++ people, don’t forget you are returning pointers in the accessors of the AddressBook class Test your AddressBook the same way you did for assignment 2. If you have coded everything properly it should work the same. Task 2 Create class called Player that…arrow_forwardC++ Double Pointer: Can you draw picture of what this means : Food **table? struct Food { int expiration_date; string brand; } Food **table; What is this double pointer saying? I know its a 2D matrix but I don't understand what I'm dealing with here. Does the statement mean that we have an array of pointers of type Food and what is the other * mean? I'm confused.arrow_forwardIn C++ Syntax for arrays of objects classGrades allCS[15]; //the default constructor is applied to //all elements of this array //to set the number of students in each class to 25 for (i = 0; i < 15; i++) { allCS[i].setNumStudents(25); } //Assume values in all classes. Write the code to print all class averages?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
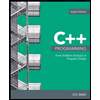
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License