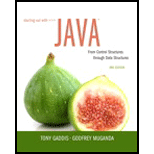
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 17.1, Problem 17.2CP
Explanation of Solution
Insertion sort:
- This is sorting
algorithm in which the first two elements of the array are taken and these elements are compared. If the swap is required, then it swaps the elements and places the elements in proper order. - Now, this becomes a sorted portion of an array. Then it starts with the third element of the array and it is compared with the already sorted portion.
- This is done to insert the elements relatively in correct position according to first two elements.
- If the swap is required on comparison, then it is need to shift the elements until the third elements is placed in it correct position.
- Now, the first three elements will be in sorted manner.
- This process is continued for the fourth and subsequent elements until all of the elements are inserted into proper positions.
Example:
Consider the elements to sort (3, 6, 5, 2, 1, 4).
- The number 3 is less than 6; so no changes are made; next number 6 is greater than 5. So, number 5 is inserted in between 3 and 6.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Double Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even.
Improved Bubble Sort: One possible improvement for Bubble Sort would be to add a flag variable and a test that…
Double Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even.
Implement the Double Insertion sort algorithm by using JAVA on a randomly generated list of N integer Your program…
Double Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even.
By using java, Implement the Double Insertion sort algorithm on a randomly generated list of N integer numbers. Your…
Chapter 17 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Prob. 17.7CPCh. 17.2 - If a sequential search is performed on an array,...Ch. 17.3 - Prob. 17.9CPCh. 17.3 - Prob. 17.10CP
Ch. 17.3 - Prob. 17.11CPCh. 17.3 - Prob. 17.12CPCh. 17.3 - Prob. 17.13CPCh. 17.3 - Prob. 17.14CPCh. 17.3 - Let a[ ] and b[ ] be two integer arrays of size n....Ch. 17.3 - Prob. 17.16CPCh. 17.3 - Prob. 17.17CPCh. 17.3 - Prob. 17.18CPCh. 17 - Prob. 1MCCh. 17 - Prob. 2MCCh. 17 - Prob. 3MCCh. 17 - Prob. 4MCCh. 17 - Prob. 5MCCh. 17 - Prob. 6MCCh. 17 - Prob. 7MCCh. 17 - Prob. 8MCCh. 17 - Prob. 9MCCh. 17 - Prob. 10MCCh. 17 - True or False: If data is sorted in ascending...Ch. 17 - True or False: If data is sorted in descending...Ch. 17 - Prob. 13TFCh. 17 - Prob. 14TFCh. 17 - Assume this code is using the IntBinarySearcher...Ch. 17 - Prob. 1AWCh. 17 - Prob. 1SACh. 17 - Prob. 2SACh. 17 - Prob. 3SACh. 17 - Prob. 4SACh. 17 - Prob. 5SACh. 17 - Prob. 6SACh. 17 - Prob. 7SACh. 17 - Prob. 8SACh. 17 - Prob. 1PCCh. 17 - Sorting Objects with the Quicksort Algorithm The...Ch. 17 - Prob. 3PCCh. 17 - Charge Account Validation Create a class with a...Ch. 17 - Charge Account Validation Modification Modify the...Ch. 17 - Search Benchmarks Write an application that has an...Ch. 17 - Prob. 8PCCh. 17 - Efficient Computation of Fibonacci Numbers Modify...
Knowledge Booster
Similar questions
- A sort algorithm that finds the smallest element of the array and interchanges it with the element in the first position of the array. Then it finds the second smallest element from the remaining elements in the array and places it in the second position of the array and so onarrow_forward1. Write a program that compares all four advanced sorting algorithms . To perform the tests, create a randomly generated array of 1,000 elements. What is the ranking of the algorithms? What happens when you increase the array size to 10,000 elements and then 100,000 elements?arrow_forwardWrite a bubble sorting algorithm that sorts a 10-element array called sortarray from smallest to largest. The array starts at address 0x400. The bubble sorting algorithm starts at element 0 and continues to the end of the array and swaps adjacent elements if one is greater than the other. For example, if sortarray[0] = 10 and sortarray[1] = 7, then it would swap the elements resulting in: sortarray[0] = 7 and sortarray[1] = 10. The algorithm then continues to compare the next two elements of the array: sortarray[1] and sortarray[2]. And so on, until it compares the last two elements of the array: sortarray[8] and sortarray[9]. The algorithm repeats this process until it processes the entire array without performing a swap. Sketch high-level code (C code) that uses a for loop to perform this algorithm. Name that program bubblesort.c. You need not test your C code, and you may use any text editor to write it. Then convert the high-level code to RISC-V assembly. Have your program hold any…arrow_forward
- An input array consists of multiple integers. Write a sorting program to sort the content of thearray in place.Sample:Input: {4,2,0,3,4,0,4,1,2,1,3}Output: [0 0 1 1 2 2 3 3 4 4 4]arrow_forwardMake a programme that compares the four advanced sorting algorithms covered in this chapter. Create a 1,000-element randomly generated array for the tests. What is the algorithm's ranking? What happens when the array size is increased to 10,000, then 100,000 elements?arrow_forwardSubject: Data structurearrow_forward
- An input array consists of multiple integers. Write a sorting program to sort the content of thearray in place.Sample:Input: {4,2,0,3,4,0,4,1,2,1,3}Output: [0 0 1 1 2 2 3 3 4 4 4]arrow_forward1. Write a program that compares all four advanced sorting algorithms discussed in this chapter. To perform the tests, create a randomly generatedarray of 1,000 elements. What is the ranking of the algorithms? What happens when you increase the array size to 10,000 elements and then 100,000elements?arrow_forwardWrite a modified version of the selection sort algorithm that selects the largest element each time and moves it to the end of the array, rather than selecting the smallest element and moving it to the beginning. Will this algorithm be faster than the standard selection sort? What will its complexity class (big-Oh) be?arrow_forward
- Sort the array (D, G, J, F, A, C) using selection sort (show the array after each step).arrow_forwardComputer Science Design a logic for a program that prompt a user for 10 items and store them within an array. Using bubble sort algorithm, sort out your array and print out the median of the sorted list within the array. The list should be sorted in an ascending order.arrow_forwardThe binary search algorithm that follows may be used to search an array when the elements are in order. This algorithm is analogous to the following approach to finding a name in a telephone book. a. Open the book in the middle and look at the middle name on the page. b. b. If the middle name isn't the one, you're looking for, decide whether it comes before or after the name you want. c. Take the appropriate half of the section of the book you were looking in and repeat these steps until you land on the name. 1. Let the bottom be the subscript of the initial array element. 2. Let the top be the subscript of the last array element. 3. Let found be false. 4. Repeat as long as the bottom isn't greater than the top and the target has not been found. 5. Let middle be the subscript of the element halfway between bottom and top. 6. If the element in the middle is the target 7. Set found to true and index to middle. else if the element in the middle is larger than the target 8. Let the top be…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
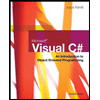
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
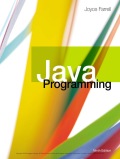
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage