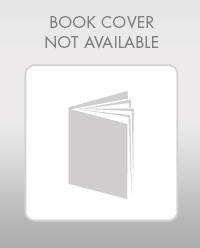
Concept explainers
A)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::printList()//Line 1
{//Line 2
//loop
//Error line3
while(head)//Line 3
{//Line 4
/*Print the data value of the node while traversing through the list*/
cout<<head->value; //Line5
/*the pointer is moved one position ahead till end of list */
head= head->next;//Line6
}//Line 7
}//Line 8
Error in the given code:
- In “line 3”, use of the head pointer to walk down the list destroys the list.
- This should be written as an “auxiliary pointer”. So, correct code is given below:
ListNode *nodePtr = head
- This should be written as an “auxiliary pointer”. So, correct code is given below:
while (nodePtr != null)
;&#x...
B)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::PrintList()//Line 1
{//Line 2
//Declaration of structure pointer variables
//Line3
ListNode *p =head; /*the start or head of the list is stored in p */
//loop
//Error Line4
while (p->next) //Line4
{//Line 5
/*Print the data value of node p while traversing through the linklist */
//Line6
cout<<p->value; /*print the individual data values of each node */
//Line7
p=p->next; //the pointer is moved one position ahead till end of list
}//Line8
}//Line9
Error in the given code:
- In “line 4”, eventually the pointer p becomes “NULL”, at which time the attempt to access p->NULL will result in an error.
- This should be written by replacing the text p->next in the while loop with p.
while (p) //Line4
/*Here the loop will traverse till p exists or till p is not NULL */
- The function fails to declare a return type of void...
C)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::PrintList()//Line 1
{//Line 2
//Declaration of structure pointer variables
//Line3
ListNode *p =head; /*the start or head of the list is stored in p */
//loop
// Line4
while (p) //Line4
{//Line 5
/*Print the data value of node p while traversing through the linklist */
//Line6
cout<<p->value; /*print the individual data values of each node */
//Error Line7
//Line7
p++//the pointer is incremented by one position
}//Line8
}//Line9
Error in the given code:
- In “line 7”, the function uses p++ erroneously in place of p=p->next when attempting to move to the next node in the list. This is not possible as increment operator can work only on variables containing data values but here p is a node of a link list which contains an address value pointer pointing to the next list along with a data value.
- This should be written by replacing the text p++ in the while loop body with p=p->next...
D)
Explanation of Solution
Purpose of the given code:
The given code is trying to destroy the members of a linked list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::~NumberList()//Line 1
{//Line 2
//Declaration of structure pointer variables
ListNode *nodePtr, *nextNode;//Line 3
//Storing "head" pointer into "nodePtr"
nodePtr = head;//Line 4
//loop
while (nodePtr != nullptr)//Line 5
{//Line 6
//Assign address of next into "nextNode"
nextNode = nodePtr->next;//Line 7
//Error
nodePtr->next=nullptr;//Line 8
//Assign nextNode into "nodePtr"
nodePtr = nextNode;//Line 9
}//Line 10
}//Line 11
Error in the given code:
In “line 8”, the address of “next” in “nodePtr” is assigned as “nullptr”.
- This should be written as “delete nodePtr” to delete the value of node from the list, because, “delete” operator is used to free the memory space allocated by the list...

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Starting Out With C++: Early Objects (10th Edition)
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
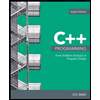
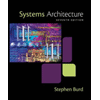
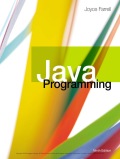
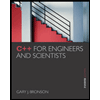
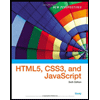