STARTING OUT WITH C++ MPL
9th Edition
ISBN: 9780136673989
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 11PC
Program Plan Intro
Generation of Subsets
Program Plan:
- Include the required header files
- Declare function prototypes.
- Define the “main()” function.
- Declare the required variables.
- Get the input from the user.
- If the user input is not in the range the condition exits the program.
- Create the list and call the function “get_Subsets ()”.
- Display the output.
- Define the overloaded stream insertion operator for
vector of int.- Display the open square bracket.
- If the number is in the range, display the number inside the bracket.
- Display the close square bracket.
- Return the output to the main function.
- Define the overloaded stream insertion operator for a list of generic type.
- Display the open square bracket.
- Create a list and declare the variable name “itr” for the list.
- While condition used to check if the “itr” is not equal to last number in the same list.
- If so, display the number.
- Increment the “itr”.
- Print the numbers separated by commas.
- Display the close square bracket.
- Return the output to the main function.
- Define the “get_subsets” vector function.
- Declare the list of subsets
- Start with a list of subsets of 1 to n that contains on the empty set.
- The “if” condition is used to check if the “n” is greater than “k” value.
- Temporarily used to extend the subset list by declaring the vector variables.
- While condition used to check if the “itr” is not equal to last number in the same list.
- Declare the vector variables.
- Push the value to the list.
- Increment the “itr” value.
- Set the “sub_setList” variable with the “big_List” value.
- Return the value to the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Introduction
For this assignment, you are to write a program which implements a Sorted List data structure using a circular
array-based implementation and a driver program that will test this implementation.
The Sorted List ADT is a linear collection of data in which all elements are stored in sorted order. Your
implementation has to store a single int value as each element of the list and support the following operations:
1. add(x) – adds the integer x to the list. The resulting list should remain sorted in increasing order. The time
complexity of this operation should be 0(N), where N is the size of the list.
2. removefirst() - deletes the first integer from the list and returns its value. The remaining list should
remain sorted. Time complexity of this operation should be 0(1).
3. removelast() – deletes the last integer from the list and returns its value. The remaining list should
remain sorted. Time complexity of this operation should be 0(1).
4. exists(x) – returns true if the…
Suppose you have two objects of Doubly Linked List D1 and D2. Each object is representing a different Doubly Linked List. Write a function to concatenate both doubly linked lists. The concatenation function must return the address of head node of concatenated Doubly Linked List, and it must take two Node* parameters. You need to keep in mind all exceptional cases for example what if either one of the doubly linked lists is empty? Perform this task on paper, take a clear picture of solution and paste it in answer section.
must answwer attach output screenshot
Chapter 17 Solutions
STARTING OUT WITH C++ MPL
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Similar questions
- Solve in C++ using the given code and avoid using vectorsarrow_forwardThe following question requires to be done in Python. Thanks in advancearrow_forward1. a function that takes in a list (L), and creates a copy of L. note: The function should return a pointer to the first element in the new L. [iteration and recursion]. 2. a function that takes in 2 sorted linked lists, and merges them into a single sorted list. note: This must be done in-place, and it must run in O(n+m).arrow_forward
- QUESTION: NOTE: This assignment is needed to be done in OOP(c++/java), the assignment is a part of course named data structures and algorithm. A singly linked circular list is a linked list where the last node in the list points to the first node in the list. A circular list does not contain NULL pointers. A good example of an application where circular linked list should be used is a items in the shopping cart In online shopping cart, the system must maintain a list of items and must calculate total bill by adding amount of all the items in the cart, Implement the above scenario using Circular Link List. Do Following: First create a class Item having id, name, price and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in list Display all items. Traverse…arrow_forwardlinear search array c++arrow_forward[] [] partite_sets In the cell below, you are to write a function "partite_sets (graph)" that takes in a BIPARTITE graph as its input, and then returns a single list whose two entries are the partite sets of the graph as lists (the order of the sets outputed does not matter). After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (partite_sets({"A" : ["B", "C"], "B" : partite_sets({"A" : ["B", "C"], "B" : ["A"], "C" : ["A"]}), ["A", "D"], "C" : ["A", "D"], "D" : ["B", "C"]})) This should return [["B","C"], ["A"]] [["A","D"], ["B","C"]] (the order in which the entries appear does not matter) Python Pythonarrow_forward
- Exercise B: "Matrix Addition" For this exercise you will design and implement a function that takes two matrix arguments and computes their sum (if and only if a sum can actually be computed, returning an empty list otherwise). Two matrices can only be added together if they have exactly the same dimensions. If the dimensions are acceptable, then the matrix sum will have the same dimensions as either of the operands, and the value of each element in the matrix sum is itself the sum of the corresponding elements in the operand matrices. And although it does makes sense to test rectangularity before attempting addition, you need not perform this step (since you did it above). In order to complete this task, you will need to: ensure you know how matrix addition can be performed² ● Your submission for this exercise:arrow_forward2 in python pleasearrow_forward6. Write a function DOT-PRODUCT that takes two lists, each list has the same number of elements, and produces the dot product of the vectors that they represent. For example, (DOT-PRODUCT '(1.2 2.0 -0.2) '(0.0 2.3 5.0)) -> 3.6 Hint: The key for designing a lisp function is using recursion.arrow_forward
- Python data structures: write a function that takes in a list (L) as input, and returns the number of inversions. An inversion in L is a pair of elements x and y such that x appears before y in L, but x > y. Your function must run in -place and implement the best possible algoritm of time complexity. def inversions(L: List) -> int:'''finds and returns number of inversions'''arrow_forwardExercise G -- Implement a function halves that takes a list of integers and divides each element of the list by two (using the integer division operator //) NOTE use the map function combined with a lambda expression to do the division with a neat solution halves : List Int -> List Int halves xs = [ ] --remove this line and implement your halves function herearrow_forwardLanguage: C++ Solve the following problem using a Singly Linked List.Given a singly linked list of characters, write a function to make a word out of given letters in the list Example:Input:C->S->A->R->B->B->E->LNULLOutput:S->C->R->A->B->B->L->E->NULLarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
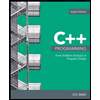
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning