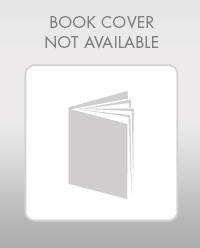
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 8PC
Program Plan Intro
Modification of “SearchableVector” class
Program plan:
SimpleVector.h:
- Include the required header files in the program.
- Define the template class “SimpleVector”:
- Declare the required class data members and exception function under “private” access specifier.
- Declare the required class member functions and class constructor under “public” access specifier.
- Define the “SimpleVector” function:
- Get the array size and allocate the memory for corresponding array size
- If allocation gets fail, then call the “memError()” function.
- Else initialize the array elements.
- Define the copy constructor of the “SimpleVector” function:
- Copy the array size and allocate the memory for corresponding array size
- If allocation gets fail, then call the “memError()” function.
- Else copy the array elements.
- Define the “~SimpleVector” class destructor:
- Delete the allocated memory for the array.
- Define the “getElement” function:
- Get the array subscript and check it is in the array range.
- If not then call the exception function.
- Return the subscript.
- Define the operator overload for “[]” operator
- Get the array subscript and check it is in the array range.
- If not then call the exception function.
- Return the subscript.
- Define the “push_back()” function:
- Get the element which is going to insert.
- Create a new array and allocate the memory for the array which is greater than 1 from an old array.
- Copy the elements from old array to new array.
- Insert the new element at the last of the array.
- Delete the old array and make the pointer points the new array
- Finally adjust the array size.
- Define the “pop_back()” function:
- Save the last element which is going to delete.
- Create a new array and allocate the memory for the array which is lesser than 1 from an old array.
- Copy the elements from old array to new array.
- Delete the old array and make the pointer points the new array
- Finally adjust the array size.
- Return the deleted value.
SearchableVector.h:
- Include the required header files in the program.
- Define the template class “SearchableVector”, which is derived from the above class template “SimpleVector”:
- Declare the required class member functions and class constructor under “public” access specifier.
- Define the copy constructor of the “SearchableVector” function:
- Call the base class copy constructor for copying the array size and allocate the memory for corresponding array size.
- Copy the array elements.
- Define the function “findItem”
- Declare the required variables in the function.
- Get the element from the function call.
- Using while loop
- Find the middle element of the array.
- Check the middle element is the searching element.
- If so, then return the array subscript.
- Else, check the middle element is greater than the searching element.
- If so, decrease the last index value.
- Else, increase the starting index value.
- If the element is not found, then return -1 as the array subscript.
Main.cpp:
- Include the required header files in the program.
- Create an object for the class “SearchableVector” for integer type.
- Create an object for the class “SearchableVector” for double type.
- Use for loop to iterate array elements
- Insert the integer value on integer array.
- Insert the double value on double array.
- Using for loop, display an integer array on the output screen.
- Using for loop, display the double array on the output screen.
- Call the function “findItem” using the searching element which is integer.
- Compare the result of the function and display the message according to the condition.
- Do the same again using double value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need help to solve the following case, thank you
hi I would like to get help to resolve the following case
Could you help me to know features of the following concepts:
- defragmenting.
- dynamic disk.
- hardware RAID
Chapter 16 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - The function int minPosition(int arr[ ], int size)...Ch. 16.2 - What must you be sure of when passing a class...Ch. 16.2 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - In the following Rectangle class declaration, the...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 6RQECh. 16 - A(n)______ container organizes data in a...Ch. 16 - Prob. 8RQECh. 16 - Prob. 9RQECh. 16 - Prob. 10RQECh. 16 - Write a function template that takes a generic...Ch. 16 - Write a function template that is capable of...Ch. 16 - Describe what will happen if you call the function...Ch. 16 - Prob. 14RQECh. 16 - Each of the following declarations or code...Ch. 16 - Prob. 16RQECh. 16 - String Bound Exceptions Write a class BCheckString...Ch. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Sequence Accumulation Write n function T...Ch. 16 - Rotate Left The two sets of output below show the...Ch. 16 - Template Reversal Write a template function that...Ch. 16 - SimpleVector Modification Modify the SimpleVector...Ch. 16 - Prob. 8PCCh. 16 - Sortabl eVector Class Template Write a class...Ch. 16 - Prob. 10PCCh. 16 - Word Transformers Modification Modify Program...Ch. 16 - Prob. 12PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
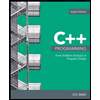
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
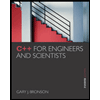
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
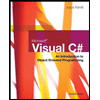
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
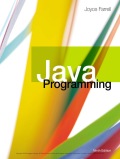
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
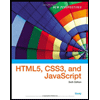
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning