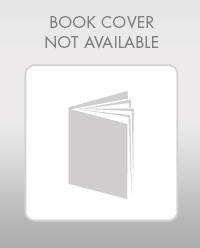
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 2PC
Program Plan Intro
Arithmetic Exception
Program Plan:
- Include the required header files.
- Create SqrtException class for arithmetic exception.
- Define the function mySqrt() that accepts the integer parameter.
- Check whether the number is less than 0. If yes, throw arithmetic exception
- Loop executes until the square of r is less than or equal to number.
- Check whether square of r is equal to number. If yes,
- Return r value.
- Check whether square of r is equal to number. If yes,
- There is no square root, then throw an arithmetic exception.
- Define the “main()” function.
- Read the inputs from the user.
- In the Try block
- Take square root of given number.
- Display the square root of number.
- In the Catch block,
- Display the error message.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
use C++ programing language
Write a program that prompts the user to enter a person's date of birth in numeric form such as 8-27-1980. The program then outputs the date of birth in the form: August 27, 1980. Your program must contain at least two exception classes: invalidDay and invalidMonth. If the user enters an invalid value for day, then the program should throw and catch an invalidDay object. Similar conventions for the invalid values of month and year. (Note that your program must handle a leap year.)
in python
Cpp program
Write a Garage class that has a
Car that is having troubles with its Motor. Use a function-level try block in the Garage class constructor to catch an exception (thrown from the Motor class) when its Car object is initialized. Throw a different exception from the body of the Garage constructor s handler and catch it in main().
Chapter 16 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.2 - Prob. 16.6CPCh. 16.2 - The function int minPosition(int arr[ ], int size)...Ch. 16.2 - What must you be sure of when passing a class...Ch. 16.2 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - In the following Rectangle class declaration, the...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 6RQECh. 16 - A(n)______ container organizes data in a...Ch. 16 - Prob. 8RQECh. 16 - Prob. 9RQECh. 16 - Prob. 10RQECh. 16 - Write a function template that takes a generic...Ch. 16 - Write a function template that is capable of...Ch. 16 - Describe what will happen if you call the function...Ch. 16 - Prob. 14RQECh. 16 - Each of the following declarations or code...Ch. 16 - Prob. 16RQECh. 16 - String Bound Exceptions Write a class BCheckString...Ch. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Sequence Accumulation Write n function T...Ch. 16 - Rotate Left The two sets of output below show the...Ch. 16 - Template Reversal Write a template function that...Ch. 16 - SimpleVector Modification Modify the SimpleVector...Ch. 16 - Prob. 8PCCh. 16 - Sortabl eVector Class Template Write a class...Ch. 16 - Prob. 10PCCh. 16 - Word Transformers Modification Modify Program...Ch. 16 - Prob. 12PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Subject: Object Oriented PrgrammingLanguage: Java ProgramTopic: Exception (SEE ATTACHED PHOTO FOR THE PROBLEM)arrow_forwardneed c++ thanks Write a class named TestScores . The class constructor should accept an array of test scores as its argument. The class should have a member function that returns the average of the test scores. If any test score in the array is negative or greater than 100, the class should throw an exception. Demonstrate the class in a program.arrow_forwardInstructions: Exception handing Add exception handling to the game (try, catch, throw). You are expected to use the standard exception classes to try functions, throw objects, and catch those objects when problems occur. At a minimum, you should have your code throw an invalid_argument object when data from the user is wrong. File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File…arrow_forward
- c++ programming Lab 10-1: Write a test program to accept two numbers from consol. Then check to see if first number is larger than second number . If it is throwing an exception and handle the error properly. Lab 10-2: Write a test program to accept a number from consol. then check to see if the number is positive or negative. If it is negative throw an exception and indicate the number is negative otherwise take the squire root of the number. Use exception handling to control the flow of the code. Lab 10-3: Write a test program to accept a number from consol. This number is radius of circle if the number is positive find area and circumference. Otherwise, throw an exception with proper message. Lab 10-4: Show the output of the following code with input 10, 60, and 120, respectively.arrow_forwardC++ languagearrow_forwardInstructions: In the code editor, you are provided with a treasureChestMagic() function which has the following description: Return type - void Name - treasureChestMagic Parameters - an address of an integer Description - updates the value of a certain integer randomly You do not have to worry about how the treasureChestMagic() function works. All you have to do is ask the user for an integer and then call the treasureChestMagic() function, passing the address of that integer you just asked. Finally, print the updated value of the integer inputted by the user. Please create a main code for my function that works with this. This is my code: #include<stdio.h>#include<math.h> void treasureChestMagic(int*); int main(void) { // TODO: Write your code here return 0;} void treasureChestMagic(int *n) { int temp = *n; int temp2 = temp; *n = *n + 5 - 5 * 5 / 5; temp2 = (int) pow(2, 3); if(temp % 3 == 0) { *n = temp * 10; } else if(temp %…arrow_forward
- Exceptions are caught and handled by usingarrow_forwardC++ Visual Studio 2019 Complete #13. Dependent #1 Employee and ProductionWorker classes showing below. Modify the Employee and ProductionWorker classes so they throw exceptions when the following errors occur: The Employee class should throw an exception named InvalidEmployeeNumber when it receives an employee number that is less than 0 or greater than 9999. The ProductionWorker class should throw an exception named InvalidShift when it receives an invalid shift. The ProductionWorker class should throw an exception named InvalidPayRate when it receives a negative number for the hourly pay rate. Write a driver program that demonstrates how each of these exception conditions works. #1 Employee and ProductionWorker classes #include <string>#include <iostream>#include <iomanip>using namespace std; class Employee{private: string name; // Employee name string number; // Employee number string hireDate; // Hire date public: // Default…arrow_forwardin c++ Define a new exception class named “BadNameException” that must inherit from the C++ runtime_error class. It simply manages the specific reason of the error (string). It must be able to describe the reason for the error. Please note that if runtime_error class is not available, you may inherit from the exception class.arrow_forward
- CUSTOM EXCEPTION 1 Donor Eligibility Write a C++ program to handle the exception. Read all the details and check for the eligibilty of the donors. Conditions: Age must be greater than 17. Minimum weight should be greater than 44 kg. Maximum amount of blood can be drawn is 350ml. if any of the conditions are not met then throw an exceptibn Refer the sample input/output below. Input and Output Format: Refer sample input and output for formatting specifications. All text in bold corresponds to input and the rest corresponds to output. [All text in bold corresponds to input and P Type here to searcharrow_forwardTrue or False An output parameter works like a by value parameter.arrow_forwardC++ Each Fraction contains a numerator, denominator, a whole number portion, and has access to several functions you have developed, including overloaded operators. Complete these tasks: a. Create a FractionException class. The class contains a Fraction object and a string message explaining the reason for the exception. Include a constructor that requires values for the Fraction and the message. Also include a function that displays the message. b. Modify each Fraction class constructor and data entry function so that it throws a FractionException whenever a client attempts to instantiate a Fraction with a zero denominator. c. Write a main()function that asks the user to enter values for four Fractions. If the user attempts to create a Fraction with a zero denominator, catch the exception, display a message, and force the Fraction to 0 / 1. Display the four Fraction objects. Save the file as FractionException1.cpp.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
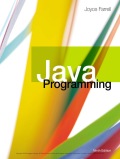
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
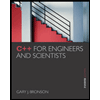
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
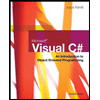
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
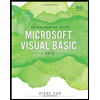
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning