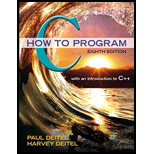
Concept explainers
(Date Class) Create a class called ate that includes three pieces of information as data members—a month (type int), a day (type int) and a year (type int). Your class should have a constructor with three parameters that uses the parameters to initialize the three data members. For the purpose of this exercise, assume that the values provided for the year and day are correct, but ensure that the month value is in the range 1–12; if it isn’t, set the month to 1. Provide a set and a get function for each data member. Provide a member function displayData that displays the month, day and year separated by forward slashes (/). Write a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
C How To Program Plus Mylab Programming With Pearson Etext -- Access Card Package (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Starting Out with C++: Early Objects
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with Python (4th Edition)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- Problem (Online Address Book): Using classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType with following members and methods: firstname, lastname, and accessors and mutators, print to display, and constructors. Define a class dateType for month, day and year as members with its accessors, mutators, and constructors) Design a class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information.…arrow_forwardRewrite the calculator program using a class called calculator. Your program will keep asking the user if they want to perform more calculations or quit and will have a function displayMenu to display the different functions e.g .(1 - addition, 2- subtraction, 3- multiplication, 4- division) Your program must have appropriate constructors and member functions to initialize, access, and manipulate the data members as well as : A member function to perform the addition and return the result A member function to perform the subtraction and return the result A member function to perform the multiplication and return the result A member function to perform the division and return the resultarrow_forwardPythonarrow_forward
- computer programming (C++ language)arrow_forward(Java) The Sculpture Subclass Write class as follows: The class is named Sculpture, and it inherits from the Painting class. It has a private boolean member variable named humanForm It has a default constructor that assigns the values "No name" to name, "No artist" to artist, -1 to the year, "No medium" to the medium, and false to the humanForm variable. This default constructor calls the five argument constructor. It has a five-argument constructor to assign values to the name, artist, year, medium, and humanForm variables. It has a getter and setter for the humanForm variable. It has a toString() method. This class contains no other methods Make sure to include your name, the name of this class, our course number, and the Activity number in a Javadoc comment at the top. Make sure to write a Javadoc comment for each of these methods.arrow_forward(Account class)Create an Account class that a bank might use to represent customers’ bank accounts. Include a data member of type int to represent the account balance. Provide a constructor that receives an initial balance and uses it to initialize the data member. The constructor should validate the initial balance to ensure that it’s greater than or equal to 0. If not, set the balance to 0 and display an error message indicating that the initial balance was invalid. Provide three member functions. Member function credit should add an amount to the current balance. Member function debit should withdraw money from the Account and ensure that the debit amount does not exceed the Account’s balance. If it does, the balance should be left unchanged, and the function should print a message indicating "Debit amount exceeded account balance." Member function get_Balance should return the current balance. Create a program that creates two Account objects and tests the member functions of class…arrow_forward
- (java) The Painting Subclass Write class as follows: The class is named Painting, and it inherits from the Art class. It has a private String member variable named medium It has a default constructor that assigns the values "No name" to name, "No artist" to artist, -1 to year, and "No medium" to the medium variable. This default constructor calls the four-argument constructor. It has a four-argument constructor to assign values to the name, artist, year, and medium variables. It has a getter and settersfor the medium variable. It has a toString() method This class contains no other methods Make sure to include your name, the name of this class, our course number, and the Activity number in a Javadoc comment at the top. Make sure to write a Javadoc comment for each of these methods.arrow_forward(Person Class) Design a class named Person that contains: o name, gender, and personCase as a private attribute o Non-default constructor that specifies name and gender o toString method that returns person data o personCase () method with no implementation (Student Class) Design a class named Student which is a child of Person that contains: o studentID as a private attribute o Nondefault constructor that specifies the name, gender, and studentID o toString method that returns student data o Implement personCase () that assigns "Not Studying" to personCase if studentID equals 0, “Studying" in case studentID greater than 0, and "Not a student" in case studentID less than 0. (Employee Class) Design a class named Employee which is a child of Person that contains: o employeelD as a private attribute o Non-default constructor that specifies the name, gender, and employeelD o toString method that returns employee data o Implement personCase () that assign “Technical" to personCase if…arrow_forward(Java) The Abstract Art Class Write an abstract class as follows: The class is named Art It inherits from the Comparable interface It has a private String member variable named name It has a private String member variable named artist It has a private int member variable called year It has a default constructor that assigns the values "No name" to name, "No artist" to artist and -1 to the year. This default constructor calls the three argument constructor. It has a three-argument constructor to assign values to the name, artist and year variables. It has a copy constructor that makes a copy of another non-null Art object It has getters and setters for the name, artist and year variables It has a toString() method that creates a string of artist, with name and year tabbed once on subsequent lines (see sample output) It has an equals method that compares this Art to another Object It has a compareTo method that compares in this order: 1) artist, 2) name, 3) year This class contains no…arrow_forward
- Nonearrow_forwardReadme.md: Stars(C++) This lab exercise will practice creating objects with constructors and destructors and demonstrate when constructors and destructors are called. Star Class Create a class, Star. A Star object has two member variables: its name, and a solar radius. This class should have a constructor which takes a std::string, the name of the star, and a double, the solar radius of the star. In the constructor, the Star class should print to the terminal that the star was born. For example, if you create a Star as follows: Star my_star("Saiph", 22.2); Then the constructor should print: The star Saiph was born. In the destructor, the Star class should print to the terminal that the star was destroyed, along with the number of times the volume of the sun that that star was, formatted to two decimal places. Hint: use the following line to set the precision to 2 decimal places: std::cout << std::fixed << std::setprecision(2); For example, when my_star above has its…arrow_forward5 (The Time class) Design a class named Time. The class contains: ■ Data fields hour, minute, and second that represent a time. ■ A no-arg constructor that creates a Time object for the current time. ■ A constructor that constructs a Time object with a specified elapse time since the middle of night, Jan 1, 1970, in seconds. ■ A constructor that constructs a Time object with the specified hour, minute, and second. ■ Three get functions for the data fields hour, minute, and second. ■ A function named setTime(int elapseTime) that sets a new time for the object using the elapsed time. Draw the UML diagram for the class. Implement the class. Write a test program that creates two Time objects, one using a no-arg constructor and the other using Time (555550), and display their hour, minute, and second. (Hint: The first two constructors will extract hour, minute, and second from the elapse time. For example, if the elapse time is 555550 seconds, the hour is 10, the minute is 19, and the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
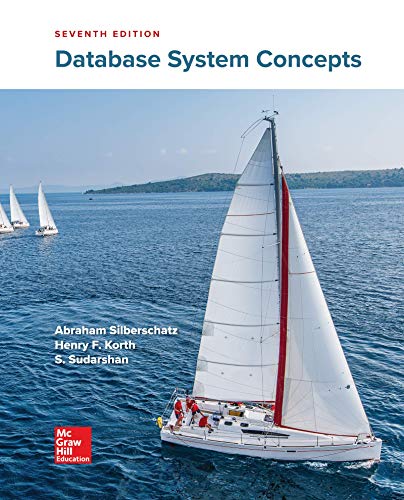
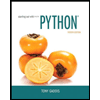
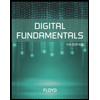
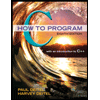
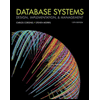
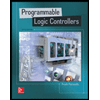