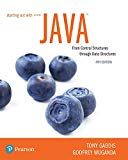
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 15, Problem 7AW
Write an iterative version (using a loop instead of recursion) of the factorial method shown in this chapter.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please do not use any AI tools to solve this question.
I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor.
No AI-generated responses, please.
Obtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).
I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ?
Cisco Packet Tracer
1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.
Chapter 15 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 15.2 - It is said that a recursive algorithm has more...Ch. 15.2 - Prob. 15.2CPCh. 15.2 - What is a recursive case?Ch. 15.2 - What causes a recursive algorithm to stop calling...Ch. 15.2 - What is direct recursion? What is indirect...Ch. 15 - Prob. 1MCCh. 15 - This is the part of a problem that can be solved...Ch. 15 - This is the part of a problem that is solved with...Ch. 15 - This is when a method explicitly calls itself. a....Ch. 15 - Prob. 5MC
Ch. 15 - Prob. 6MCCh. 15 - True or False: An iterative algorithm will usually...Ch. 15 - True or False: Some problems can be solved through...Ch. 15 - True or False: It is not necessary to have a base...Ch. 15 - True or False: In the base case, a recursive...Ch. 15 - Find the error in the following program: public...Ch. 15 - Prob. 1AWCh. 15 - Prob. 2AWCh. 15 - What will the following program display? public...Ch. 15 - Prob. 4AWCh. 15 - What will the following program display? public...Ch. 15 - Convert the following iterative method to one that...Ch. 15 - Write an iterative version (using a loop instead...Ch. 15 - What is the difference between an iterative...Ch. 15 - What is a recursive algorithms base case? What is...Ch. 15 - What is the base case of each of the recursive...Ch. 15 - What type of recursive method do you think would...Ch. 15 - Which repetition approach is less efficient: a...Ch. 15 - When recursion is used to solve a problem, why...Ch. 15 - How is a problem usually reduced with a recursive...Ch. 15 - Prob. 1PCCh. 15 - isMember Method Write a recursive boolean method...Ch. 15 - String Reverser Write a recursive method that...Ch. 15 - maxElement Method Write a method named maxElement,...Ch. 15 - Palindrome Detector A palindrome is any word,...Ch. 15 - Character Counter Write a method that uses...Ch. 15 - Recursive Power Method Write a method that uses...Ch. 15 - Sum of Numbers Write a method that accepts an...Ch. 15 - Ackermarms Function Ackermanns function is a...Ch. 15 - Recursive Population Class In Programming...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Convert the following Whi1e loop to a For loop: Declare Integer count = 0 While count 50 Display "The count is...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Under what circumstances does Java automatically provide a default constructor for a class?
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Car Class Write a class named Car that has the following member variables: yearModelan int that holds the cars...
Starting Out with C++ from Control Structures to Objects (9th Edition)
The job of the _____ is to fetch instructions, carry out the operations commanded by the instructions, and prod...
Starting Out With Visual Basic (8th Edition)
A file exists on the disk named students. txt. The file contains several records, and each record contains two ...
Starting Out with Python (4th Edition)
In Exercises 41 through 46, identify the errors. Dima,b,c,dAsDoublea=2b=3c=d=4Istoutput.Items.Add(5((a+b)/(c+d)
Introduction To Programming Using Visual Basic (11th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- using r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forward
- using r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forward
- Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forward
- Considering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forwardGiven the FSA below, answer the following questions. b 1 3 a a b b с 2 A. Write a RegEx that is equivalent to this FSA as it is; B. Write a RegEx that is equivalent to this FSA removing the states and edges corresponding to the letter c. Note: To both items feel free to use any method you want, including analyzing which words are accepted by the FSA, to generate your RegEx.arrow_forward3) Finite State Automata Given the FSA below, answer the following questions. a b a b 0 1 2 b b 3 A. Give three 4-letter words that can be accepted by this FSA; B. Give three 4-letter words that cannot be accepted by this FSA; C. How could you describe the words accepted by this FSA? D. Is this FSA deterministic or non-deterministic?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
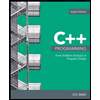
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
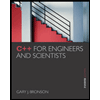
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
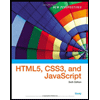
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
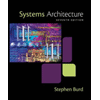
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
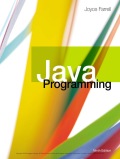
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Computational Software for Intelligent System Design; Author: Cadence Design Systems;https://www.youtube.com/watch?v=dLXZ6bM--j0;License: Standard Youtube License