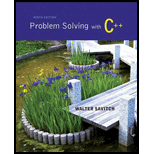
Flesh out

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Java How To Program (Early Objects)
Computer Systems: A Programmer's Perspective (3rd Edition)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- IN C++ In your previous class, move the member variables (student name and grades) as private. Create the proper functions to fill data in them. In main, create an instance of that array and fill that instance with data. Call the DisplayInfo() function from main so it prints the content of the object. A screenshot showing the code in Visual Studio and the output (on the console screen). previous activity class code:- #include <iostream>using namespace std;class student{ public: string Name; string grade[3]; void DisplayInfo() { cout<<"Student Name:"<<Name; cout<<"\nGrade:"<<grade[1]; }};int main(){ cout << "Welcome to my world..." << endl; system("pause"); student obj; obj.Name="Rahul"; obj.grade[1]="A++"; obj.DisplayInfo(); return 0;}arrow_forwardWhat would be the possible situations that a copy constructor is activated? a. It can be called to construct a new object, just like any other constructor. b. It is also called when a value parameter is an object. c. It is call when a function returns an object. d. It is call when an object is destroyed. Group of answer choices a, b, and c a, b, c, ad d b and c a only a and barrow_forward1. Create a Student class that implements the Person interface. As well as storing the students name and email, also store their course grade (e.g A, B, C) in a member variable. The grade should be accessible via a getGrade method. For the implementation of getDescription return a message along the lines of “A C grade student”, substituting the students actual grade.2. Create a Lecturer class that implements the Person interface. This class should also store the subject that the lecturer teaches. Add a getSubject method, and implement getDescription so that it returns a suitable message, e.g. “Teaches Biology”.3. Create a third class, Employee that implements the interface. This should also store the name of the department the Employee works in (available via getDepartment). Again, getDescription should return a suitable message.arrow_forward
- Pythonarrow_forwardCreate a class MyTime which has the datamembers as follows: 1. hour: integer (1 to 12)2. minute: integer (0 to 59)3. second: integer (0 to 59)4. pm: bool variable. True means PM time and false means AM time. Implement a default constructor, a parameterized constructor and a copy constructor. Write set and get functions for all the four members of the class. Overload these operators: 1. Extraction operator >> : Prompt the user for hours, minutes, seconds and for am/pm and initialize the structure. Left operand istream object and right operand MyTime2. Assignment = : Assign the right object to the left object. Both operands are of type MyTime.3. Insertion << : Print the time in the format HH:MM SS PM. Left operand of type ostream and right operand of type MyTime. this in c++.arrow_forwardRedo Programming Exercise 3 by overloading the operators as nonmembers of the class boxType. Write a test program that tests various operations on the class boxType.arrow_forward
- A class Student can have three member variables: name, age, and an array of grades of two exams marks [5]. The grades can be integers (0-100), doubles (0.0-100.00) and characters (A-F, where F denotes grades less than 50). Write a class template to accommodate all five types of grades. The class template must have two constructors (no-arg constructor, and aconstructor that takes 7 parameters: name, age and grades of 5 exams). Write a c++ function to read an object from console, and another function to print the object to the console. Implement the class template by creating three objects with three different types (int, double and char) of grades, and printing the objects on the console.arrow_forwardCan you call a constructor from another constructor? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first. Problem Statement# A class can have multiple parameterized constructors which can call each other. You are given a partially completed code of a Car class in the editor. Modify the parametrized constructor which assigns parameters to the required fields. Input# carName, carModel, carCapacity Output# carName, carModel, carCapacity Sample Input# "Ferrari", "F8", "100" Sample Output# "Ferrari", "F8", "100" Part of solution // Car class class Car { // Private Fields private String carName; private String carModel; private String carCapacity; // Default Constructor public Car() { this.carName = ""; this.carModel = ""; this.carCapacity = ""; } // Parameterized Constructor 1 public Car(String name, String model) { this.carName = name; this.carModel = model; } // Parameterized Constructor 2 public…arrow_forwardPythonarrow_forward
- objective of the project: Implement a class address. An address has a house number street optional apartment number city state postal code. All member variables should be private and the member functions should be public. Implement two constructors: one with an apartment number one without an appartment number. Implement a print function that prints the address with the street on one line and the city, state, and postal code on the next line. Implement a member function comesBefore that tests whether one address comes before another when the addresses are compared by postal code. Returns false if both zipcodes are equal. Use the provided main.cpp to start with. The code creates three instances of the Address class (three objects) to test your class. Each object will utilize a different constructor. You will need to add the class definition and implementation. The comesBefore function assumes one address comes before another based on zip code alone. The test will also return…arrow_forwardImplement a nested class composition relationship between any two class types from the following list: Advisor Вook Classroom Department Friend Grade School Student Teacher Tutor Write all necessary code for both classes to demonstrate a nested composition relationship including the following: a. one encapsulated data member for each class b. inline default constructor using constructor delegation for each class c. inline one-parameter constructor for each class d. inline accessors for all data members e. inline mutators for all data membersarrow_forwardlanguage is c++ sample output included with user input in boldarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
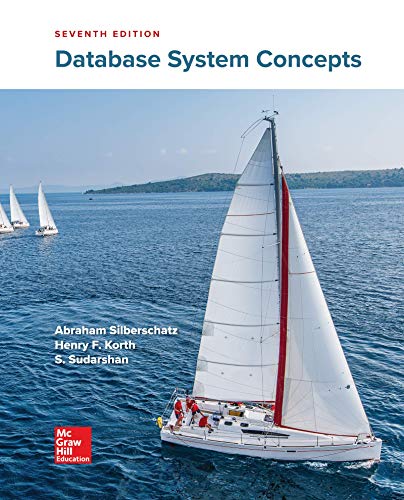
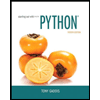
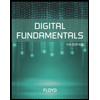
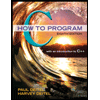
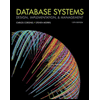
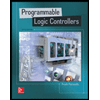