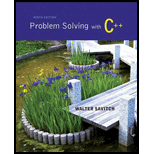
Consider a graphics system that has classes for various figures—rectangles, squares, triangles, circles, and so on. For example, a rectangle might have data members for height, width, and center point, while a square and circle might have only a center point and an edge length orradius, respectively. In a well-designed system, these would be derived from a common class, Figure. You are to implement such a system. The class Figure is the base class. You should add only Rectangle and Triangle classes derived from Figure. Each class has stubs for member functions erase and draw. Each of these member functions outputs amessage telling what function has been called and what the class of the calling object is. Since these are just stubs, they do nothing more than output this message. The member function center calls the erase and draw functions to erase and redraw the figure at the center. Since you have only stubs for erase and draw, center will not do any “cantering” but will call the member functions erase and draw. Also add an output message in the member function center that announces that center is being called. The member functions should take no arguments.
There are three parts to this project:
a. Write the class definitions using no virtual functions. Compile andtest.
b. Make the base class member functions virtual. Compile and test.
c. Explain the difference in results.
For a real example, you would have to replace the definition of each of these member functions with code to do the actual drawing. You will be asked to do this in
Use the following main function for all testing:
//This program tests Programming Project 5. #include <iostream> #include "figure.h" #include "rectangle.h" #include "triangle.h" using std::cout; int main( ) { Triangle tri; tri.draw( ); cout<< "\nDerived class Triangle object calling center( ).\n"; tri.center( ); //Calls draw and center Rectangle rect; rect.draw( ); cout<< "\nDerived class Rectangle object calling center().\n"; rect.center( ); //Calls draw and center return 0; } |

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Concepts Of Programming Languages
Introduction to Programming Using Visual Basic (10th Edition)
Starting Out with Python (4th Edition)
Starting out with Visual C# (4th Edition)
- Using the class diagram in the image below, write a small report (200- 300 words) on how to create customer, car, and parking lot classes for an object-oriented parking system using java.arrow_forwardIn this problem you will implement a simple library system based on object-oriented approach (using inheritance, polymorphism and abstract classes). At the library we have items and people are allowed to borrow them for a while or use them in the library premises. We have 3 types of items at the library. Books, Magazines and Compact Discs (CDs). All items have a unique number called serial number, shelf number that the item put on that shelf and shelf index, the index of the item at the shelf. Items at the library have additional different properties changing to item type. For example books have name, publisher name, and author name. CDs have title property and magazines have name, publisher properties. But people are allowed to borrow only books and CDs, but not magazines. Suppose that two kinds of people using library items. These are students and academic staff. Students can borrow only one item at a time, and academic stuffs can borrow at most 3 items at the same time. Also people…arrow_forwardDraw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a…arrow_forward
- make a program by use of two abstract classes, Position and Maze. A Position is used to identify a unique location within a maze. Any Position can be transformed into another Position by asking it for an adjacent position to the north, south, east, or west.arrow_forwardWrite the classes as shown in the following class diagram. Add a tester that asks the user for a cat, dog and a BigDog then call all of their methods. Note that Cat and Dog inherit from the abstract class Animal and BigDog inherits from Dog.arrow_forwardLet’s consider a superclass Vehicle. Different vehicles have different features and properties however there few of them are common to all. Speed, color, fuel used, size are few which are common to all. Hence we can create a class ‘Vehicle’ with states and actions that are common to all vehicles. The subclass of this superclass can be any type of vehicle. Example: Class Car A has all the features of a vehicle. But it has its own attributes which makes it different from other subclasses. By using inheritance we need not rewrite the code that we’ve already used with the Vehicle. The subclass can also be extended. We can make a class ‘Sports Car’ which extends ‘Car’. It inherits the features of both ‘Vehicle’ and ‘Car’.arrow_forward
- Now we are going to use the design pattern for collecting objects. We are going to create two classes, a class AmazonOrder that models Amazon orders and a class Item that models items in Amazon orders. An item has a name and a price, and the name is unique. The Item class has a constructor that takes name and price, in that order. The class also has getters and setters for the instance variables. This is the design pattern for managing properties of objects. The setName() method should do nothing if the parameter is the empty string, and the setPrice() method should do nothing if the parameter is not positive. The class also has a toString() method that returns a string representation for the item in the format “Item[Name:iPad,Price:399.99]”. For simplicity, we assume an Amazon order can have at most 5 items, and class AmazonOrder has two instance variables, an array of Item with a length of 5 and an integer numOfItems to keep track of the number of items in the…arrow_forwardDraw an inheritance hierarchy representing parts of a -computer system (processor, memory, disk drive, DVD drive, printer, scanner, keyboard, mouse, etc.).arrow_forwardCreate a Dog and a Cat class, each of which inherit from Animal. Each class should have a constructor which initialses their name and a hello method. The cat's hello method should return the string "Meow" and the dog's hello method should return the string "Woof". The Animal class is public abstract class Animal { public Animal(String n) { name = n; } abstract String hello(); public String greeting() { return hello() + ", I am + name; } // private private String name; } Your classes will be used in the following Main class. public class Main { public static void main(String [] args) { Animal cat = new Cat("Angel"); Animal dog = new Dog("Fido"); System.out.println(cat.greeting()); System.out.println(dog.greeting()); } } Note that your Cat and Dog classes must not supply a greeting method. They should use the greeting method from the Animal class.arrow_forward
- Develop a Library Management system for borrowing and returning activities The basic classes could be the main Library, Subscribers, borrowing records, Contents, articles, e.t.c. NB: Contents may include books, journal articles or digital media. Also a subscriber may borrow books for one month, after which a fee accrue until the borrowed item is returned. On the other hand, golden subscribers may borrow for 2 months without fee before fees begin. Provides an interface for the user to: A. Adding/editing/deleting instances belonging to each class, B. Subscriber browsing library contents and select items to borrow. C. Subscriber returning borrowed item and check his balance and pay any late fee if any. D. Admin can print reports of overdue borrowed items.arrow_forwardUsing JAVA Language Consider a Billing class that implements an interface Payable having a method getTotalPaymentAmount(). Besides this, you have a Doctor class with private instance variables (docID, docName, and docFee) and a public getDoc() method, Patient class with private instance variables (pName, pID, pDisease), Medicine class with private instance variables (medID, medName, medQty, medPrice), and MedicalTest class with private instance variables (testID, testName, testPrice). Each of these classes has the toString() method to display the information of its object. The Billing class is having "Has A" relationship with the other four classes (Doctor, Patient, Medicine, and MedicalTest) mentioned above. The getPaymentAmount() method of Billing class returns the total billing amount that includes doc fee, medicine cost, and medical test fee that a patient has to pay. After implementing these classes, you are required to do the following in the driver class: Create an ArrayList of…arrow_forwardAn arithmetic progression is a sequence of numbers such that the difference between the consecutive terms is constant. For instance, the sequence 5, 7, 9, 11, 13, 15 is an arithmetic progression with a common difference of 2.Given the class IntNumber:1 public class IntNumber2 {3 public final int value;45 public IntNumber(int number) {6 this.value = number;7 }8 }where value is an immutable field, that can be directly accessed (public), implement a class called ArithmeticProgressionthat implements the following methods:•1 public static IntNumber getArithmeticProgressionDifference(ArrayList<IntNumber> list)which returns the difference between terms of the arithmetic progression (as an object of type IntNumber) if thevalues of the IntNumber objects are an arithmetic progression. The method returns null in any other case.•1 public static void printIsArithmeticProgression(ArrayList<IntNumber> list)which prints on the console:The list is an arithmetic progression with difference =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
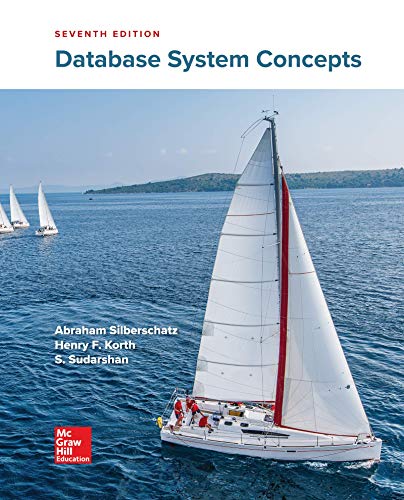
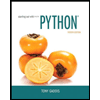
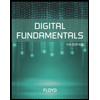
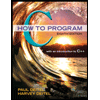
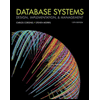
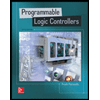