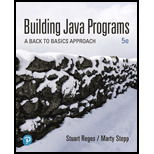
Explanation of Solution
Adding the “lastIndexOf” methods in “ArrayIntList” class:
//definition of "ArrayIntList" class
public class ArrayIntList
{
//declare the required variables
private int[] elementData;
private int size;
//refer the remaining methods in the textbook
//definition of "lastIndexOf" method
public int lastIndexOf(int value)
{
/*iterate "i" until it reaches the size of an array*/
for (int i = size - 1; i >= 0; i--)
{
//check the condition
if (elementData[i] == value)
{
//return the "i" value
return i;
}
}
//return the value
return -1;
}
}
Explanation:
In the above program, the “ArrayIntList” is the class name,
- Inside the “ArrayIntList” class, declare the “elementData”, and “size” variables as a private integer datatype.
- The “lastIndexOf” method is defined inside the “ArrayIntList” class with the integer value as the parameter.
- The “for” loop is used to iterate the value until it reaches size of an array.
- The “if” loop is used to check whether the array of element value is equal to passed value.
- The “ith” position of an array value is returned.
- The “if” loop is used to check whether the array of element value is equal to passed value.
- If the value is not in an array return the -1 value.
- The “for” loop is used to iterate the value until it reaches size of an array.
- The “lastIndexOf” method is defined inside the “ArrayIntList” class with the integer value as the parameter.
Want to see more full solutions like this?
Chapter 15 Solutions
Building Java Programs: A Back To Basics Approach, Loose Leaf Edition (5th Edition)
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
- I need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forwardNeed help completing this algorithm here in coding! 2arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
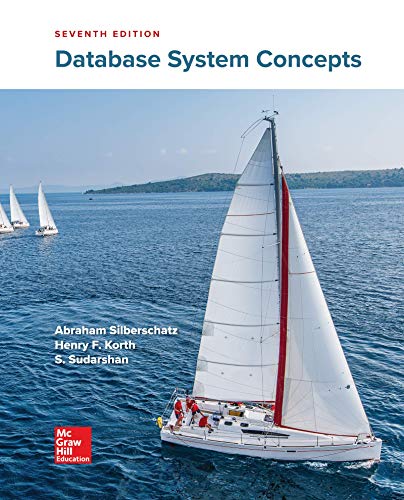
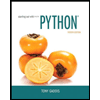
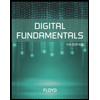
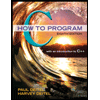
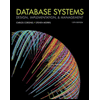
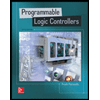