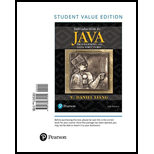
Concept explainers
Pick Four cards
Program Plan:
- Import the required packages.
- Create a class Myclass
- Declare the necessary variables
- Using start initialize the required
- Create a new array list
- Loop that iterates to all the 52 values into the list.
- Create and initialize a random shuffle method.
- Create “hbox” and add cards randomly.
- Align the position of the cards.
- Create a new button.
- Add an action even to the button.
- Shuffle randomly to place four cards.
- Initialize the new pane.
- Add the created items into the pane.
- Create a scene based on the defined positions
- Set title for the preview
- Display the cards after refresh button is pressed.
- Define the main method.
- Initialize the call.

The below program is used to pick four cards randomly by pressing refresh button:
Explanation of Solution
Program:
//import the required headers
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.image.ImageView;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
import java.util.ArrayList;
//define the class Myclass
public class Myclass extends Application
{
@Override
//start method gets overidden in the application class
public void start(Stage primeview) {
// create a new list
ArrayList<Integer> mylist = new ArrayList<>();
// iterate for all cards
for (int iter = 1; iter <= 52; iter++)
{
// add the values to the list
mylist.add(iter);
}
// shuffle method call
java.util.Collections.shuffle(mylist);
// new Hbox declaration
HBox my_hBox = new HBox(5);
// set the alignment to be center
my_hBox.setAlignment(Pos.CENTER);
// add card 1 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(0) + ".png"));
// add card 2 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(1) + ".png"));
// add card 3 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(2) + ".png"));
// add card 4 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(3) + ".png"));
// new button gets created
Button btRefresh = new Button("Refresh");
// action event for the button gets created
btRefresh.setOnAction(e -> {
// shuffling the card
java.util.Collections.shuffle(mylist);
// clear the contents of the hbox
my_hBox.getChildren().clear();
// add card 1 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(0) + ".png"));
// add card 2 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(1) + ".png"));
// add card 3 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(2) + ".png"));
// add card 4 to the hbox
my_hBox.getChildren().add(new ImageView("exer1/image/card/" + mylist.get(3) + ".png"));
});
// new border pane is created
BorderPane new_pane = new BorderPane();
// align the position
new_pane.setCenter(my_hBox);
// align the button position
new_pane.setBottom(btRefresh);
// add the contents to the pane
BorderPane.setAlignment(btRefresh, Pos.TOP_CENTER);
// new scene gets created
Scene n_scene = new Scene(new_pane, 250, 150);
// title of the stage is set
primeview.setTitle("Pick four cards");
// the stage gets placed in the scene
primeview.setScene(n_scene);
// stage gets displayed
primeview.show();
}
// main method
public static void main(String[] args)
{
// initilaize calls
launch(args);
}
}
The below output will be displayed initially:
Screenshot of initial stage
When the refresh button is clicked the cards gets shuffled and it will be displayed as shown below:
Screenshot after rotation
Want to see more full solutions like this?
Chapter 15 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version, Student Value Edition (11th Edition)
- What are the four types of feasibility? what is the issues addressed by each feasibility component.arrow_forwardI would like to get ab example of a situation where Agile Methods might be preferable versus the traditional SDLC? What are the characteristics of this situation that give Agile Methods an advantage?arrow_forwardWhat is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forward
- What are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forward
- Security in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forwardPlease include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward
- 1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forwardWhen using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forwardFind the error, assume data is a string and all variables have been declared. for ch in data: if ch.isupper: num_upper = num_upper + 1 if ch.islower: num_lower = num_lower + 1 if ch.isdigit: num_digits = num_digits + 1 if ch.isspace: num_space = num_space + 1arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
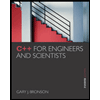
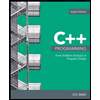
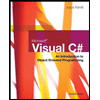
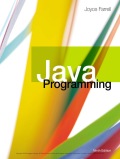