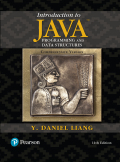
Concept explainers
a.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
}
//Finally block
finally
{
statement4;
}
statement5;
b.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
}
//Finally block
finally
{
statement4;
}
statement5;
c.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
throw ex2;
}
//Finally block
finally
{
statement4;
}
statement5;
d.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
throw ex2;
}
//Finally block
finally
{
statement4;
}
statement5;

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
- Could you help me to know features of the following concepts: - defragmenting. - dynamic disk. - hardware RAIDarrow_forwardwhat is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forward
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
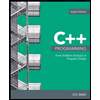
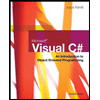
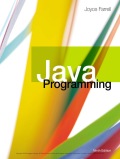
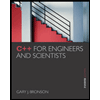
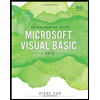