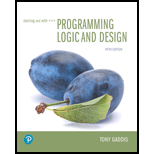
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 12, Problem 4SA
Program Plan Intro
Character testing library functions:
- Library functions other than the string library functions are provided by
programming language, which are intended to work with single characters. - The support library functions are used for testing the value of the character.
- The function will return the Boolean result on testing; the result will be in “True” or “False” format.
isUpper(character):
- This function is used to validate whether the character given is upper case letter.
- The function will return “True” if the character is in upper case.
- The function will return “False” if the character is in lower case.
isLower(character):
- This function is used to validate whether the character given is lower case letter.
- The function will return “True” if the character is in lower case.
- The function will return “False” if the character is in upper case.
Example:
The below
//determine if the first character is in upper case
If isUpperCase (str[0]) Then
//replace the values present at the first position
Set str [0] = "0"
//end if
End If
Explanation:
- If condition validates the first position of the “str” is in upper case or not.
- If the condition becomes true, the character at positon “0” gets replaced with the character “0”.
Accessing string using subscript notation:
- The string that are accessed using the subscript notation uses subscript “0” to hold the first character and similarly till the last character.
- The first value the index value will be “0” and to find the last character use “length(variable)-1”.
- When a string is tried to access using an invalid subscript, it will throw an error.
Analysis of the given statement:
//declare and define a string
Declare string str= “Might”
//set the value located at the subscript 5 as defined
Set str[5] = “y”
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a C++ (visual studio) program that examines a list of numbers to find the smallest value. The list should include 10,000 random whole numbers that range from -1,000,000 to 1,000,000. The program should display the lowest value in the list and the number of the element that contains that value.And create a pseudocode explaining the steps. (if you would give to someone to create the program)
The program must have:Declare an array in the main() function that will hold 10,000 integers.
Pass the array to the other functions as necessary.
Use a reliable algorithm to produce random integers within a given range.
Note: stran()/rand() is NOT a reliable random number algorithm.
The function should accept the minimum and maximum values that will be generated and return an integer.Examine the array to find the smallest value.
Return the smallest value and the number of the element that contains the smallest value.
char lastChar(const char *str){ char last; //loop over the string and go uptill end of the string \0 while(*str !='\0'){ str++; // increment pointer last = *str; //store the current character to the last variable } return last; //last character is returned}
C++
Chapter 12 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 12.2 - Prob. 12.1CPCh. 12.2 - Prob. 12.2CPCh. 12.2 - Design an algorithm that determines whether the...Ch. 12.2 - Design an algorithm that determines whether the...Ch. 12.2 - Prob. 12.5CPCh. 12.2 - Prob. 12.6CPCh. 12 - Prob. 1MCCh. 12 - Prob. 2MCCh. 12 - If the str variable contains the string "berry",...Ch. 12 - If the str variable contains the string "Redmond",...
Ch. 12 - Prob. 5MCCh. 12 - Prob. 1TFCh. 12 - Prob. 2TFCh. 12 - If the String variable str contains the string...Ch. 12 - The insert library module automatically expands...Ch. 12 - Prob. 5TFCh. 12 - Prob. 6TFCh. 12 - Prob. 7TFCh. 12 - Prob. 1SACh. 12 - If the following pseudocode were an actual...Ch. 12 - Prob. 3SACh. 12 - Prob. 4SACh. 12 - Prob. 5SACh. 12 - Design an algorithm that counts the number of...Ch. 12 - Prob. 2AWCh. 12 - Design an algorithm that counts the number of...Ch. 12 - Design an algorithm that deletes the first and...Ch. 12 - Design an algorithm that converts each occurrence...Ch. 12 - Design an algorithm that replaces each occurrence...Ch. 12 - Assume the following declaration exists in a...Ch. 12 - Prob. 1DECh. 12 - Prob. 2DECh. 12 - Prob. 3DECh. 12 - Prob. 1PECh. 12 - Sentence Capitalizer Design a program that prompts...Ch. 12 - Prob. 3PECh. 12 - Sum of Digits in a String Design a program that...Ch. 12 - Prob. 5PECh. 12 - Alphabetic Telephone Number Translator Many...Ch. 12 - Word Separator Design a program that accepts as...Ch. 12 - Pig Latin Design a program that reads a sentence...Ch. 12 - Prob. 9PECh. 12 - File Encryption File encryption is the science of...Ch. 12 - File Decryption Filter Design a program that...Ch. 12 - Password Weakness Detector Design a program that...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- c code Screenshot and output is mustarrow_forwardchar[ ] letters=new char[5];int x = 0;while(x < 10){ar[x]='a';x++;}arrow_forwardfunction main() { # ist: input numbers #w: outer for loop index # X: inner for loop index # y: number of parsmeters # min: index for max value at the time of iteration # buf: used for swapping ____________________a _______ #declare local vars let=___________b___ #intialize aary with the parametrs y= _______c___ # find the lenght of lstfor((________________)); do min=________e_____ # intialize main index for ((___________f_______)); do # find index for main value in one line. use a short tets. _________g_____done # swap- two values using two indices, min and outerloop # use buf to hold value when swapping ________________h________ # move min lst [.] to buf ________________i_______ # move lst[.] to lst[.] _________________j_____ # move buf to lst[.]done}main "@" # pass the input parameters to the function main# end of bash script show me the ss when u run chatgpt doesnt give right codearrow_forward
- C++ Write a program that first asks the user to enter a string and then asks the user to enter a character. The program should display the number of times the character appears in the string. Use the function Count_char(). A function Count_char() that has two arguments. The first argument is an array and the second argument is a character. The function returns an integer.arrow_forwardAssume that int a[ 2 ][ 2 ] = { { 1, 2 }, { 3, 4 } }; the value of a[ 1, 1 ] = ______;arrow_forwardC++ PLS HELP allow the user to enter the size of the randomly generated array of integers, then display the resultsarrow_forward
- All arrays in C# have a that is set to the array's number of items.arrow_forwardProgramming Language: C++ Create a structure Student and ask the user how many students. Create an array which will save the number of students in the input. Ask for the data of the first and last student and display them.arrow_forwardC Programming "Arrays"arrow_forward
- You will write a program that allows the user to enter 10 songs and their artists into a playlist and will sort the entries by artist order. The program should use a 10 element array of structure objects that holds two string variables: artist and title.arrow_forwardPalindrome Checkercreate a JavaScript program that checks if phrasesentered by the user are palindromes. A palindrome is a word, number, phrase, or other sequence of characters which reads the same backward as forward, such as “SIR”,“raceBIKE”, “Never odd or even”, “20/1/20”. The program should prompt the user to typein a phrase that is at least three characters long, and then tell the user whether the phrasewas a palindrome. Note, the decision should be based solely on letters and numericaldigits, and so it should not consider other characters, such as spaces or exclamationmarks, even though these might be entered by the user. when checking palindromes.A. Select your test data with care. Both palindromes and non-palindromes would be included in this. Provide examples that are text, numeric, and mixed; ignore any additional characters and spaces; andB. Ask the user for the word and then read their input. To verify that their input string has been read correctly, test this by displaying…arrow_forwardC programming language questionarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
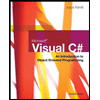
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
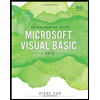
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning