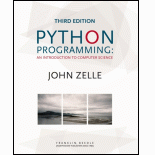
Tracking conference attendees
Program plan:
- In a file “atmmanager.py”,
- Create a class “ATMAp” that calls account information from JSON file and modifies it according to the commands given through an interface class.
- Define the function “_init_()” to create a list of users and manage the account information.
- Define the “run()” function to execute the transaction according to user input.
- Define the function “screen_1()” to return the user input.
- Define the function “screen_2()” to process the transaction according to the user input.
- Define the function “get_User()” to return the user with the given user id.
- Define the function “send_Money()” to move the amount from one user to another. There are two types of accounts are used; “Checking” and “Savings”.
- Define the function “_updateAccounts()” to update the amount in output file whenever the transaction has occurred.
- Define the function “verify()” to check whether the userid and pin given by the user is matched with the saved userid and pin.
- Create a class “User”,
- Define the function “_init_()” method to assign the initial values.
- Define the function “check_Balances()” to return the amount of balance in checking and savings account.
- Define the function “exchange_Cash()” to deposit or withdraw amount fro checking or savings account.
- Define the function “transfer_Money()” to transfer the amount between checking and savings account.
- Define the function “getID()” to return the user id.
- Define the function “getPIN()” to return the pin.
- Create a class “ATMAp” that calls account information from JSON file and modifies it according to the commands given through an interface class.
- In the file “textatm.py”,
- Create a class “TextInterface”,
- Define “_init_()” method to assign initial values.
- Define the function “get_UserInput()” to return the user inputs.
- Define the function “choose_Transaction()” to select the type of transaction.
- Define the function “display_Balanace()” to print the amount in savings and checking accounts.
- Define the function “withdraw_Cash()” to return the withdraw amount and account type.
- Define the function “getTransferInfo()” to return the information of amount transfer between checking and savings account.
- Define the function “getSendInfo()” to return the information of receiver amount transfer.
- Define the function “close()” to exit the transaction.
- Create a class “TextInterface”,
- In a file “atm.py”,
- Import necessary packages.
- Define the “main()” function,
- All the interface class “TextInterface”.
- Call the “ATMAp” class functions with the argument of file and interface.
- Call the “main()” function.

This Python program is to keep track of conference attendees.
Explanation of Solution
Program:
File name: “atmmanager.py”
#Import package
import json
#Create a class
class ATMAp:
#Define the function
def __init__(self, account_file, interface):
#Open the file
with open(account_file) as fi:
#Serialize the object as a json stream
data = json.load(fi)
#Create a list of users
self.users = []
'''Create for loop to iterate key, value pairs till the list of tuples'''
for key,value in data['users'].items():
#Assign the value
usr = User(value["userid"], value["pin"],value["checkin"],value["saving"], value["name"])
#Append to the list
self.users.append(usr)
#Assign the value
self.interface = interface
#Call the run()
self.run()
#Define the run() function
def run(self):
#Execute while loop
while not self.interface.close():
#Call the functions
self.screen_1()
self.screen_2()
#Define the function
def screen_1(self):
#Assign the values
user_id = None
pin = None
#Execute while loop
while not self.verify(user_id, pin):
#Make simultaneous assignment
user_id, pin = self.interface.get_UserInput()
#Assign the value return from get_USer()
self.user = self.get_User(user_id)
#Define the function
def screen_2(self):
#Assign the value
terminate = self.interface.close()
#Execute while loop
while not terminate:
#Make simultaneous assignment
checkin, saving = self.user.check_Balances()
#Assign the value
tran_type = self.interface.choose_Transaction()
#Call the function
self.__updateAccounts()
#Execute the condition
if tran_type[0] == "C":
#Call the function
self.interface.display_Balance(checkin, saving)
#Execute the condition
elif tran_type[0] == "W":
#Make simultaneous assignment
value, account_type = self.interface.withdraw_Cash()
#Call the function
self.user.exchange_Cash(value, account_type)
#Execute the condition
elif tran_type[0] == "T":
#Make simultaneous assignment
inaccount, outaccount, value = self.interface.getTransferInfo()
#Call the function
self.user.transfer_Money(inaccount, outaccount, value)
#Execute the condition
elif tran_type[0] == "S":
#Make the assignment
recipient, type_sender, type_recipient, value = self.interface.getSendInfo()
#Assign the value return from get_User()
recipient = self.get_User(recipient)
#Call the function
self.send_Money(self.user, recipient, value, type_sender, type_recipient)
#Execute the condition
elif tran_type[0] == "Q":
#Call the function
self.user.exchangeCash(-100, "Checking")
#Execute the condition
elif tran_type[0] == "E":
#Call the unction
self.__updateAccounts()
#Assign the boolean value
terminate = True
#Define the function
def get_User(self, userid):
#Create for loop
for user in self.users:
#Check the condition
if userid == user.getID():
#Return the user object for the given userid
return user
#Define the function
def send_Money(self, sender, recipient, value, type_sender, type_recipient):
#Assign the values return from check_Balances()
checking, savings = sender.check_Balances()
checking, savings = recipient.check_Balances()
#Call the functions
sender.exchange_Cash(-value, type_sender)
recipient.exchange_Cash(value, type_recipient)
#Define the function
def __updateAccounts(self):
#Create dictionary
data = {}
#Assign empty dictionary
data['users'] = {}
#Create for loop
for usr in self.users:
#Assign the values
json_str = (vars(usr))
user_id = usr.getID()
#Assign the value
data['users'][user_id] = json_str
#Open the output file in write mode
with open('new_atmusers.json', 'w') as f:
#Serialize the objecct as a json stream
json.dump(data, f, indent=4)
#Define the function
def verify(self, user_id, pin):
#Create for loop
for usr in self.users:
#Execute the condition
if (usr.getID() == user_id) and (usr.getPIN() == pin):
#Return boolean value
return True
#Otherwise
else:
#Return boolean value
return False
#Create a class
class User:
#Define _init_() method
def __init__(self, userid, pin, checkin, saving, name):
#Assign initial values
self.userid = userid
self.pin = pin
self.checkin = float(checkin)
self.saving = float(saving)
self.name = name
#Define the function
def check_Balances(self):
#Return two values
return self.checkin, self.saving
#Define the function
def exchange_Cash(self, value, inaccount):
#Check the condition
if inaccount[0] == "C":
#Assign the value
self.checkin = round(self.checkin + value, 2)
else:
#Assign the value
self.saving = round(self.saving + value, 2)
#Define the function
def transfer_Money(self, inaccount, outaccount, value):
#Check the condition
if inaccount[0] == "C":
#Assign the values
self.checkin = self.checkin + value
self.saving = self.saving - value
#Otherwise
else:
#Assign the values
self.checkin = self.checkin - value
self.saving = self.saving + value
#Define the function
def getID(self):
"""Returns userid"""
#Return the value
return self.userid
#Define the function
def getPIN(self):
#Return the value
return self.pin
File name: “textatm.py”
#Create a class
class TextInterface:
#Define the function
def __init__(self):
#Assign boolean value
self.active = True
#Print the string
print("Welcome to the ATM")
#Define the function
def get_UserInput(self):
#Get user inputs
a = input("Username >> ")
b = input("Pin >> ")
#Return the values
return a, b
#Define the function
def choose_Transaction(self):
#Display user balance
#Assign the value
tran = "xxx"
#Execute the loop
while tran[0] != ("C" or "W" or "T" or "S" or "E" or "Q"):
#Get the choice
tran = input('Please select a transaction from the approved list. "Check Balance", \n "Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> ')
#Check the condition
if tran[0] != "E":
#Return the choice
return tran
else:
#Assign boolean value
self.active = False
#Return the choice
return tran
#Define the function
def display_Balance(self, checking, savings):
#Print savings and checking
print("Checking: {0} Savings: {1}".format(checking, savings))
#Define the function
def withdraw_Cash(self):
#Print the string
print("This function will withdraw or deposit cash.")
#Get the values
value = eval(input("Enter the amount to deposit/withdraw? Enter negative value to withdraw. >> "))
account = input("Checking or Savings account? >> ")
#Print the string
print("Transaction is complete.\n")
#Retuen the values
return value, account
#Define the function
def getTransferInfo(self):
#Get the inputs
outaccount = input("Select account to withdraw money from: >> ")
inaccount = input("Select account to deposit money in: >> ")
value = eval(input("How much would you like to move?"))
#Print the string
print("Transaction is complete.\n")
#Return the values
return inaccount, outaccount, value
#Define the function
def getSendInfo(self):
#Get the inputs
recipient = input("Recipient userid")
type_sender = input("Sender: Checking or Savings? >> ")
type_recipient = input("Recipient: Checking or Savings? >> ")
value = eval(input("How much to send? >> "))
#Print the string
print("Transaction is complete.\n")
#Return the values
return recipient, type_sender, type_recipient, value
#Define the function
def close(self):
#Execute the condition
if self.active == False:
#Print the string
print("\nPlease come again.")
#Return boolean value
return True
#Otherwise
else:
#Return boolean value
return False
File name: “atm.py”
#Import packages
from atmmanager import ATMAp, User
from textatm import TextInterface
#Define the function
def main():
#Assign the value return from TextInterface()
interface = TextInterface()
#Assign the value return from ATMApp class
app = ATMAp("new_atmusers.json", interface)
#Call the function
main()
Content of “new_atmusers.json”:
{
"users": {
"xxxx": {
"userid": "xxxx",
"pin": "1111",
"checkin": 2000.0,
"saving": 11000.0,
"name": "Christina"
},
"ricanontherun": {
"userid": "ricanontherun",
"pin": "2112",
"checkin": 8755.0,
"saving": 7030.0,
"name": "Christian"
},
"theintimidator": {
"userid": "theintimidator",
"pin": "3113",
"checkin": 1845.0,
"saving": 112.0,
"name": "Sean"
},
"jazzplusjazzequalsjazz": {
"userid": "jazzplusjazzequalsjazz",
"pin": "4114",
"checkin": 4489.0,
"saving": 24000.0,
"name": "Dan"
}
}
}
Output:
Welcome to the ATM
Username >> xxxx
Pin >> 1111
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Check Balance
Checking: 2000.0 Savings: 11000.0
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Exit
Please come again.
>>>
Additional output:
Welcome to the ATM
Username >> xxxx
Pin >> 1111
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Withdraw/Deposit Cash
This function will withdraw or deposit cash.
Enter the amount to deposit/withdraw? Enter negative value to withdraw. >> 100
Checking or Savings account? >> Checking
Transaction is complete.
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Exit
Please come again.
>>>
Want to see more full solutions like this?
Chapter 12 Solutions
Python Programming: An Introduction to Computer Science
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- You will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forwardConstruct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
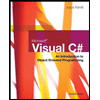
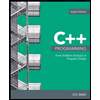
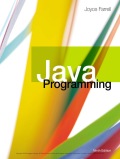
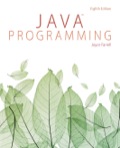
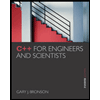