Explanation of Solution
The corrected program is given below with errors and corrections explained in the in-lined comments:
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
//declare double type pointer variables
double *length;
double *width;
//set the output format
cout << fixed << showpoint << setprecision(2);
//allocate memory to be referred to by length
length = new double;
//the statement is incorrect as length is pointer
//variable and not a double variable
///length = 6.5;
//so the correct code is
*length = 6.5;
//statement is incorrect as & is an address of operator
/// &width = 3.0;
//so the correct set of statements are shown below
//where first memory is allocated and then the value
//is assigned
width = new double;
*width = 3...

Trending nowThis is a popular solution!

Chapter 12 Solutions
C++ PROGRAMMING LMS MINDTAP
- Broken Cabins Problem Statement: There is an Office consisting of m cabins enumerated from 1 to m. Each cabin is 1 meter long. Sadly, some cabins are broken and need to be repaired. You have an infinitely long repair tape. You want to cut some pieces from the tape and use them to cover all of the broken cabins. To be precise, a piece of tape of integer length t placed at some positions will cover segments 5,5+1-sit-1. You are allowed to cover non-broken cabins, it is also possible that some pieces of tape will overlap. Time is money, so you want to cut at most k continuous pieces of tape to cover all the broken cabins. What is the minimum total length of these pieces? Input Format The first line contains three integers n,m and k(1sns10°, namsloº, Isksn) - the number of broken cabins, the length of the stick and the maximum number of pieces you can use The second line contains n integers bl,b2,bn (Isbism) - the positions of the broken cabins. These integers are given in increasing…arrow_forwardAnalyze the following code. int x = 13B while (0 < x) && (x < 100) System.out .printin(x++); The numbers 2 to 100 are displayed. The code does not compile because the loop body is not in the braces. O The loop runs forever. The code does not compile because (0 < x) && (x < 100) is not enclosed in a pair of parentheses. The numbers 1 to 99 are displayed.arrow_forwarda. Create a 1D array of integers to store 50 integers. b. Store values from 0 to 49 into the array you just created. c. Create a new String Object using no-arg constructor. d. Using for loop append the array elements one by one to the String (one per loop iteration) e. Record and display a run-time it took to append all integers to the String (record run-time of 1.d.)). E.g. "It took nanoseconds to append 50 integers to the Stringarrow_forward
- Section Test of Application... Note: You need to press "Final Submit" for your pr Given a string in which the same character occurs in many.consecutive character elements. Your task is to find the characters that have even frequency and are consecutive. Display the sum of every frequency count (For even frequency only). Example1: Input aaabbaccccdd Input String Output: Explanation: Input string "aabbaccccdd" contains 'a' 3 times, b' 2 times, again 'a' 1 time, 'c' 4 times, and 'd 2 times Here, the frequency of b.c and d is even and the frequency count is 2,4 and 2 respectively. Hence the output32-4-2%3D8 Example2: input: vdkkmmmnn Output Buns andur-arrow_forward"Scan Line Conversion". All questions require you to extend one of the line drawing algorithm to handle difference combination of the two end points. For the programming question, the final result function should be assigned to a variable named myAnswer. The variable myAnswer need to be accessed by the system, it should NOT preceded by let nor var.The output need to plot such a line. 2.[JavaScript] Extend the Octantal DDA algorithm so that it can handle any combination of x1, y1, x2, y2. Your function must based on the original Octantal DDA algorithm and the resulting function should be assigned to the myAnswer variable.arrow_forwardIn python, Old-fashioned photographs from the nineteenth century are not quite black and white and not quite color, but seem to have shades of gray, brown, and blue. This effect is known as sepia, as shown in the figures below. Original Sepia Original Image & Sepia Image Write and test a function named sepia that converts a color image to sepia. This function should first call grayscale to convert the color image to grayscale. A code segment for transforming the grayscale values to achieve a sepia effect follows.Note that the value for green does not change. (red, green, blue) = image.getPixel(x, y)if red < 63: red = int(red * 1.1) blue = int(blue * 0.9)elif red < 192: red = int(red * 1.15) blue = int(blue * 0.85)else: red = min(int(red * 1.08), 255) blue = int(blue * 0. 1. GUI TEST 2. CUSTOM TEST 3. IMAGE TESTarrow_forward
- 8. Which among the following show a valid use of the Color enumeration as a parameter to the setPixel function? Select all that apply. Take note that this is a right minus wrong question. enum Color { case red, green, blue}func setPixel(x: Int, y: Int, color: Color) {// code here} Which among the following show a valid use of the Color enumeration as a parameter to the setPixel function? Select all that apply. Take note that this is a right minus wrong question. enum Color { case red, green, blue}func setPixel(x: Int, y: Int, color: Color) {// code here} 1. setPixel(x: 0, y: 0, color: .blue) 2. setPixel(x: 0, y: 0, color: .orange) 3. setPixel(x: 0, y: 0, color: Color.green) 4. setPixel(x: 0, y: 0, color: Color.yellow)arrow_forwardComplete the convert() function that casts the parameter from a float to an integer and returns the result.Note that the returned value of the convert() function is printed. Ex: If the float value is 19.9, then the output is: 19 Ex: If the float value is 3.1, then the output is: 3 this is what i have def largest_number(num1, num2, num3): if(num1 > num2 and num1 > num3): return num1 elif(num2 > num3): return num2 else: return num3 def smallest_number(num1, num2, num3): if(num1 < num2 and num1 < num3): return num1 elif(num2 < num3): return num2 else: return num3 if __name__ == '__main__': n1 = float(input()) n2 = float(input()) n3 = float(input()) print("largest:",largest_number(n1,n2,n3)) print("smallest:",smallest_number(n1,n2,n3)) it says Test convert() with random float value test_passed function missing Traceback (most recent call last): File "main.py", line 18, in <module> n1 = float(input()) EOFError: EOF when reading a line Your output Your…arrow_forwardcode in c++arrow_forward
- (Python matplotlib or seaborn) CPU Usage We have the hourly average CPU usage for a worker's computer over the course of a week. Each row of data represents a day of the week starting with Monday. Each column of data is an hour in the day starting with 0 being midnight. Create a chart that shows the CPU usage over the week. You should be able to answer the following questions using the chart: When does the worker typically take lunch? Did the worker do work on the weekend? On which weekday did the worker start working on their computer at the latest hour? cpu_usage = [ [2, 2, 4, 2, 4, 1, 1, 4, 4, 12, 22, 23, 45, 9, 33, 56, 23, 40, 21, 6, 6, 2, 2, 3], # Monday [1, 2, 3, 2, 3, 2, 3, 2, 7, 22, 45, 44, 33, 9, 23, 19, 33, 56, 12, 2, 3, 1, 2, 2], # Tuesday [2, 3, 1, 2, 4, 4, 2, 2, 1, 2, 5, 31, 54, 7, 6, 34, 68, 34, 49, 6, 6, 2, 2, 3], # Wednesday [1, 2, 3, 2, 4, 1, 2, 4, 1, 17, 24, 18, 41, 3, 44, 42, 12, 36, 41, 2, 2, 4, 2, 4], # Thursday [4, 1, 2, 2, 3, 2, 5, 1, 2, 12, 33, 27, 43, 8,…arrow_forwardProgramming Assignment 6: SwapPoints Objective: To write two missing functions that manipulate objects, to complete a given program. Please complete this SwapPoints program. so it produces the sample output shown at the end of the program, without changing the main method. Make sure to follow the instructions in the comments of the program. So your job is to write the two functions below, which are missing from the program: inputNewPlayerLocation: to input (x,y) coordinates from user (using Scanner), then return a Point object with those coordinates. swapPoints: to swap the coordinates of two Point objects. (Hint: To keep the main function's reference variables relevant, you can't swap Point objects entirely. You need to change the x and y coordinates inside the Point objects.) /* Here is the Sample Output your completed program should produce: Welcome, new player. Please enter your coordinates:x: 5y: 7Welcome, new player. Please enter your coordinates:x: 10y: 15Player1…arrow_forwardWhat do you call a function that assigns a unique real number to each element in the sample space? a. Event b. Random Variables c. Sample Spacearrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
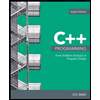
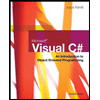