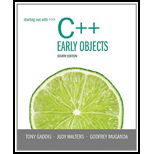
Suppose a
class CheckPoint
{
private:
int a;
protected:
int b;
int c;
void setA(int x ) {a= x;}
public:
void setB(int y) { b = y;}
void setC(int z ) { c = z ;}
};
Answer the following questions.
A) Suppose another class, Quiz, is derived from the CheckPoint class. Here is
the first line of its declaration:
class Quiz : private CheckPoint
Indicate whether each member of the CheckPoint class is private, protected, public, or inaccessible:
a
b
c
setA
setB
setC
B) Suppose the Quiz class, derived from the CheckPoint class, is declared as
class Quiz : protected Checkpoint
Indicate whether each member of the CheckPoint class is private, protected, public, or inaccessible:
a
b
C
setA
setB
setC
C) Suppose the Quiz class, derived from the CheckPoint class, is declared as
class Quiz : public Checkpoint
Indicate whether each member of the CheckPoi nt class is private, protected, public, or inaccessible:
a
b
C
setA
setB
setC
D) Suppose the Quiz class, derived from the CheckPoint class, is declared as
class Quiz: Checkpoint
Is the CheckPoint class a private, public, or protected base class?

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Starting Out with C++: Early Objects
Additional Engineering Textbook Solutions
Modern Database Management (12th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out With Visual Basic (7th Edition)
Starting Out with Java: Early Objects (6th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
- Programming Language = Python 1. Employee and ProductionWorker Classes Write an Employee class that keeps data attributes for the following pieces of information: • Employee name • Employee number Next, write a class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information: • Shift number (an integer, such as 1, 2, or 3) • Hourly pay rate The workday is divided into two shifts: day and night. The shift attribute will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write the appropriate accessor and mutator methods for each class. Once you have written the classes, write a program that creates an object of the ProductionWorker class and prompts the user to enter data for each of the object’s data attributes. Store the data in the object and then use the object’s accessor methods to retrieve it and display it on the…arrow_forward1. How many members does class test 1 have?arrow_forwardConsider the following class named Shirt: public class Shirt { private int size; private double price; public void setSize(int a) { size = a; } public void setPrice(double p) { price= p; public int getSize() {return size; } public double getPrice() { return price: } } a) Write a statement to declare an object named MyShirt b) Write statements to set the following values to the data fields of MyShirt object created above. size: 15, price: 34.99 c) Write a statement to display the value of the field size of the MyShirt object. d) Write a statement to display the value of the field priceof the MyShirt object.arrow_forward
- Consider the following class declaration: class Thing {private: int x; int y;static int z; public:Thing(){ x = y = z; }static void putThing(int a) { z = a; }};int Thing:: z = 0:Assume a program containing the class declaration defines three Thing objects with the following statement:Thing one, two, three;A) How many separate instances of the x member exist?B) How many separate instances of the y member exist?C) How many separate instances of the z member exist?D) What value will be stored in the x and y members of each object?E) Write a statement that will call the putThing member function before the Thing objects are defined.arrow_forward3. Person and Customer Classes The Person and Customer Classes Write a class named Person with data attributes for a person’s name, address, and telephone number. Next, write a class named Customer that is a subclass of the Person class. The Customer class should have a data attribute for a customer number, and a Boolean data attribute indicating whether the customer wishes to be on a mailing list. Demonstrate an instance of the Customer class in a simple program.arrow_forwardNutritional information (classes/constructors) PYTHON ONLY Complete the FoodItem class by adding a constructor to initialize a food item. The constructor should initialize the name (a string) to "None" and all other instance attributes to 0.0 by default. If the constructor is called with a food name, grams of fat, grams of carbohydrates, and grams of protein, the constructor should assign each instance attribute with the appropriate parameter value. The given program accepts as input a food item name, fat, carbs, and protein and the number of servings. The program creates a food item using the constructor parameters' default values and a food item using the input values. The program outputs the nutritional information and calories per serving for both food items. Ex: If the input is: M&M's10.034.02.01.0 where M&M's is the food name, 10.0 is the grams of fat, 34.0 is the grams of carbohydrates, 2.0 is the grams of protein, and 1.0 is the number of servings, the output is:…arrow_forward
- // Is my code correct? //please make it more efficient as you can. Thank you //please correct it if there is some mistakes public class PartTimeEmployee extends Person { //private variables private double payRate; private double hoursWorked; public PartTimeEmployee() { super(); /*invokes the default constructor of class Person */ payRate = 0; hoursWorked = 0; } public PartTimeEmployee(String idPerson, String nameFirst, String nameLast, String date, String addressPerson, double pay, double hours) { super(idPerson, nameFirst, nameLast, date, addressPerson); /*invokes the non-default constructor in other words, override the parameters from Person() non-defaukt with another set of parameters, example 'idPerson' variable overrides 'id' in Person() */ this.payRate = pay; this.hoursWorked = hours; } public String toString(String idPerson,…arrow_forward5) Consider the following code. public class Test { public static void main(String[] args){ NClass nc = new NClass(); nc.t += 1; } } class NClass { public imt t; private NClass() {//Constructor www. } } a) The program has a compilation error because the NClass class has a private constructor which is not accessible within the Test class. b) The program does not compile because the parameter list of the main method is wrong. c) The program compiles, but has a run time error because t has no initial value. d) The program compiles and runs fine.arrow_forwardclass GradedActivity { private: double score; public: GradedActivity() { score = 0.0; } GradedActivity(double s) { score = s; } void setScore(double s) { score = s; } double getScore() { return score; } char getLetterGrade() const; }; Implement the GradedActivity class above. In this C++ assignment, create a new class Assignment which is derived from GradedActivity. It should have three private member ints for 3 different parts of an assignment score: functionality (max 50 points), efficiency (max 25 points), and style (max 25 points). Create member function set() in Assignment which takes three parameter ints and sets the member variables. It should also set its score member, which is inherited from GradedActivity, using the setScore() function, to functionality + efficiency + style. Signature: void Assignment::set(int, int, int) Create a main program which instantiates an Assignment, asks the user…arrow_forward
- Number 25arrow_forwardJava:arrow_forwardConsider the following class definition: (8) class base { public: void setXYZ(int a, int b, int c) ; voidsetX ( int a ) ; int getX ()const{returnx; } void setY ( int b ); intgetY()const{returny; } int mystryNum () {return ( x &*#x00A0;y - z &*#x00A0;z) ;} void print () const; base () } base(int a, int b, int c); protected: void setz ( int c) { z= c; } void secret(); int z= 0; private: int x= 0; int y = 0; }; a. Which member functions of the class base are protected? b. Which member functions of the class base are inline? c. Write the statements that derive the class myclass from class base as a public inheritance. d. Determine which members of class base are private, protected, and public in class myclass.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
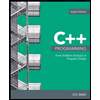
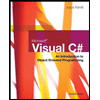
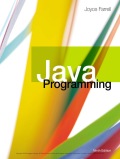
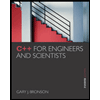
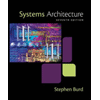