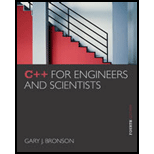
C++ for Engineers and Scientists
4th Edition
ISBN: 9781133187844
Author: Bronson, Gary J.
Publisher: Course Technology Ptr
expand_more
expand_more
format_list_bulleted
Question
Chapter 11, Problem 1PP
a)
Program Plan Intro
To construct the class Pol_coord along with the member functions convToPolar() and showdata().
a)
Expert Solution

Explanation of Solution
//class definition classPol_coord { //two floating point data members dist and mathematical private: floatdist; float theta; //constructor and function prototypes public: Pol_coord(); voidshowdata(); voidconvToPolar(float,float); }; //constructor function Pol_coord::Pol_coord() { //setting the values to 0 dist=0; theta=0; } //function for finding the polar coordinates voidPol_coord::convToPolar(float x,float y) { dist=sqrt(pow(x,2)+pow(y,2)); theta=(atan(y/x)*(180/3.1416)); } //function for showing the data voidPol_coord::showdata() { cout<<"The polar coordinates are "; cout<<dist<<", "<<theta<<endl; return; }
Explanation:
Create the class Pol_coordwith two floating data members for distance and angle. The constructor is used for assigning these two values.The function convToPolar() is used for converting them into polar coordinates using the following formula:
dist=sqrt(pow(x,2)+pow(y,2)); theta=(atan(y/x)*(180/3.1416));
b)
Program Plan Intro
- Include the class declaration and implementation in the program.
- Create the objects of the class Pol_coordin the main function.
- Calculate the polar coordinates using the method convToPolar.
- Display the data member values using the function showValues.
Program Description: The purpose of the program is to find the polar coordinate values.
b)
Expert Solution

Explanation of Solution
Program:
#include<iostream> //including the header file for mathematical functions #include<cmath> usingnamespacestd; //class definition classPol_coord { //two floating point data members dist and mathematical private: floatdist; float theta; //constructor and function prototypes public: Pol_coord(); voidshowdata(); voidconvToPolar(float,float); }; //constructor function Pol_coord::Pol_coord() { //setting the values to 0 dist=0; theta=0; } //function for finding the polar coordinates voidPol_coord::convToPolar(float x,float y) { dist=sqrt(pow(x,2)+pow(y,2)); theta=(atan(y/x)*(180/3.1416)); } //function for showing the data voidPol_coord::showdata() { cout<<"The polar coordinates are "; cout<<dist<<", "<<theta<<endl; return; } intmain() { Pol_coord a; a.convToPolar(9.09326,5.25001); a.showdata(); return0; }
Explanation:
The object of the class Pol_coord is created and the function convToPolar ( float, float ) is called with float values and then function showdata() is called for displaying the polar coordinates in the output screen.
Output Screenshot:
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
Given the following request to an AI chatbot, which response is more accurate? (You should use Google to verify that the factual claims in each response are accurate.)
Prompt
Is this true? The first recorded use of the phrase "when pigs fly" in print is in a 1616 play by Ben Jonson called "The Devil is an Ass," in which a character says, "When pigs fly, good-night!!"
Response
Response A
Response B
Yes, that's true! The phrase "when pigs fly" indeed appears in Ben Jonson's 1616 play The Devil is an Ass and was said during the second act in a conversation between Fitzdottrel and Pug. The phrase was first used to express skepticism or disbelief, suggesting that something is as unlikely to happen as the fanciful idea of pigs flying. This usage is often cited as one of the earliest instances of the phrase in print.
The claim that the phrase "when pigs fly" was first recorded in Ben Jonson's 1616 play "The Devil is an Ass" is not accurate. There is no evidence from the…
This is for my Computer Organization & Assembly Language Class
Please answer the homework scenario below and make a JAVA OOP code.
You have been hired by GMU to create and manage their course registration portal. Your first task is to develop a program that will create and track different courses in the portal. Each course has the following properties: • a course number ex. IT 106, IT 206, • A course description, ex. Intro to Programming • Total credit hour ex. 3.0, and • current enrollment ex. 30 Each course must have at least a course number and credit hours. The maximum enrollment for each course is 40 students. The current enrollment should be no greater than the maximum enrollment. A course can have a maximum of 4 credit hour. The DDC should calculate the number of seats remaining for the course. Design an object-oriented solution to create a data definition class for the course object. The course class must define all the constructors, mutators with proper validation, accessors, and special purpose methods. The DDC should calculate the…
Chapter 11 Solutions
C++ for Engineers and Scientists
Knowledge Booster
Similar questions
- For this case study, students will analyze the ethical considerations surrounding artificial intelligence and big data in healthcare, as explored in the case study found in the textbook (pages 34-36) and in the extended version available here There will also be additional articles in this weeks learning module to show both sides of the coin. https://www.delftdesignforvalues.nl/wp-content/uploads/2018/03/Saving-the-life-of-medical-ethics-in-the-age-of-AI-and-Big-Data.pdf Students should refer to the syllabus for specific guidelines regarding length, format, and content requirements. Reflection Questions to Consider: What are the key ethical dilemmas presented in the case? How does AI challenge traditional medical ethics principles such as autonomy, beneficence, and confidentiality? In what ways can responsible innovation help address moral overload in healthcare decision-making? What are the potential risks and benefits of integrating AI-driven decision-making into patient care?…arrow_forwardCan you please solve this. Thanksarrow_forwardcan you solve this pleasearrow_forward
- In the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forwardSend me the lexer and parserarrow_forward
- Here is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private…arrow_forwardIf integer x is divisible by 3, can you prove that ceil(x/2) + floor(x/6) = floor(x/2) + ceil(x/6)arrow_forwardDraw the NFA for thisarrow_forward
- What are three examples each of closed-ended, open-ended, and range-of-response questions? thank youarrow_forwardCreate 2 charts using this data. One without using wind speed and one including max speed in mph. Write a Report and a short report explaining your visualizations and design decisions. Include the following: Lead Story: Identify the key story or insight based on your visualizations. Shaffer’s 4C Framework: Describe how you applied Shaffer’s 4C principles in the design of your charts. External Data Integration: Explain the second data and how you integrated it with the Halloween dataset. Compare the two datasets. Attach screenshots of the two charts (Bar graph or Line graph) The Shaffer 4 C’s of Data Visualization Clear - easily seen; sharply defined• who's the audience? what's the message? clarity more important than aestheticsClean - thorough; complete; unadulterated, labels, axis, gridlines, formatting, right chart type, colorchoice, etc.Concise - brief but comprehensive. not minimalist but not verboseCaptivating - to attract and hold by beauty or excellence does it capture…arrow_forwardHow can I resolve the following issue?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
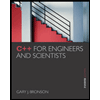
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
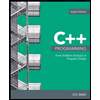
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning