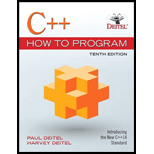
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
This is object oriented programming
Language is C++
Consider the following scenario of a class Person. A Person class has a Name, ID, Address and has functions of change the address and profile display. (Hint: Composition is not applied)
Two classes are derived from this class person. 1) Student 2) Employee.
The student class has a course number, classes attended, year (freshman=1, sophomore=2 and so on), and functions to change course and profile display.
The employee class has a Date of joining and date of promotion.
The employee class further has two child classes 1) Faculty 2) Admin.
Faculty class has a course number, classes taught, Rank (AP, lecturer etc) and a function to display profile.
Admin class has number of projects worked on and a display profile function.
Answer the questions based on this scenario.
Make the classes as mentioned above. Make appropriate constructors and functions. Choose access type wisely.
c++
1- Use UML notations to describe 4 or 5 related classes and their relationships. For each class, define the private data (variables), and the public methods (operations), and IS-A (inheritance), Has-A (Composition / Aggregation) relationships between classes. Classes related to a school and Classes related to a hospital.
with explanantion please
Chapter 11 Solutions
C++ How to Program (10th Edition)
Ch. 11 - Exercises11.3 (Composition as an Alternative to...Ch. 11 - (Inheritance Advantage) Discuss the ways in which...Ch. 11 - (Protected vs. Private Base Classes) Some...Ch. 11 - Prob. 11.6ECh. 11 - Prob. 11.7ECh. 11 - (Quadrilateral Inheritance Hierarchy) Draw an...Ch. 11 - Package Inheritance Hierarchy} Package-delivery...Ch. 11 - (Account Inheritance Hierarchy) Create an...
Knowledge Booster
Similar questions
- Consider the definition of the class product Type as given in Exercise 8. Which function members are accessors and which are mutators? (4)arrow_forwardprogramming language : C++ subject : object oriented programming question: We know that an Organization consists of Managers and Employees. You are required to create a base class Person with attributes name, CNIC and gender. Derive Manager and Employee class from Person class.arrow_forwardQuestion 2 (Student Inheritance Hierarchy) Draw a UML class diagram for an inheritance hierarchy for students at a university similar to the hierarchy shown in Fig. 11.2. Use Student as the base class of the hierarchy, then extend Student with classes UndergraduateStudent and GraduateStudent. Continue to extend the hierarchy as deeply (i.e., as many levels) as possible. For example, Freshman, Sophomore, Junior and Senior might extend UndergraduateStudent, and DoctoralStudent and MastersStudent might be derived classes of GraduateStudent. After drawing the hierarchy, discuss the relationships that exist between the classes.arrow_forward
- (Richer Shape Hierarchy) The world of shapes is much richer than the shapes included inthe inheritance hierarchy of Fig. 19.3. Write down all the shapes you can think of—both two-dimensional and three-dimensional—and form them into a more complete Shape hierarchy with asmany levels as possible. Your hierarchy should have the base class Shape from which class TwoDimensionalShape and class ThreeDimensionalShape are derived. [Note: You do not need to write any codefor this exercise.] We’ll use this hierarchy in the exercises of Chapter 20 to process a set of distinctshapes as objects of base-class Shape. (This technique, called polymorphism, is the subject ofChapter 20.)arrow_forwardusing c++arrow_forward(b) How does the static data member of a class differ from a non-static data member? Give a real-world scenario in which a static data member might be useful.arrow_forward
- *Initial Class Diagram is attached below Refine the UML class diagram so that it demonstrates the following: Classes (using the complex class symbols), Associations (with multiplicities AND association names), Generalizations (if any), and Compositions (if any) Focus ONLY on the following activities within the system: Start a Trip, Reserve a Vehicle, Make a Parking Reservation, Make a Charging Session for a Personal Vehicle, End a Trip or Charging Session, and Submit a Support Ticket for a Vehicle or Station Add the primary key necessary to support any relationships that have a multiplicity of "1" as an attribute in the corresponding classarrow_forward): Draw use case diagram for an online library system. (Eg. Saudi Digital Library SDL . **e-3: Draw Class Diagram for Toyota Company. Company deals with two types of ithat are Cars and Jeeps. Vehicles have Spare Parts (0 Breaks and Lights. Technicians (fix the spare parts whereas Engineer Inspect () Vehicles (; Alike Engine, the Vehicles. Fua-- a. Cauman Dis ADD.arrow_forwardLANGUAGE : C++ QUESTION : make a class of Student with attributes StudentName, Enrollment, semester, section, course MarksObtained and Grade. Write appropriate constructors, get and set functions for data members. The data member grade is automatically calculated based on marks obtained out of 100 for each course. also print out the outputsarrow_forward
- Game of Chess in C++ The objective of this assignment is to practice concepts related to inheritance, overriding, polymorphism, and abstract classes, to program the basics of a chess playing program. About: Chess is played on an 8 X 8 board where the initial placement of pieces. The white king is on e1, and the black king is on e8. Each chess piece can move in a specific way. Follow a simplified version. Most importantly, ignore an important rule in chess: Moving any piece in a way that puts your own king in check is illegal. Since we don't know what check means, for us a move is legal if the piece we are moving has an empty square to move to or can capture (replace) an opponent's piece (including their king). The king does not move at all. Nor can it castle the queen and knight can't move either. A pawn in the initial position may move one or two squares vertically forward to an empty square but cannot leap over any piece. Subsequently it can move only one square vertically forward…arrow_forwardSUBJECT: OOPPROGRAMMING LANGUAGE: C++ ALSO ADD SCREENSHOTS OF OUTPUT. Write a class Distance to measure distance in meters and kilometers. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class Time to measure time in hours and minutes. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class which has appropriate functions for taking objects of the Distance class and Time class to store time and distance in a file. Make the data members and functions in your program const where applicablearrow_forwardNutritional information (classes/constructors) PYTHON ONLY Complete the FoodItem class by adding a constructor to initialize a food item. The constructor should initialize the name (a string) to "None" and all other instance attributes to 0.0 by default. If the constructor is called with a food name, grams of fat, grams of carbohydrates, and grams of protein, the constructor should assign each instance attribute with the appropriate parameter value. The given program accepts as input a food item name, fat, carbs, and protein and the number of servings. The program creates a food item using the constructor parameters' default values and a food item using the input values. The program outputs the nutritional information and calories per serving for both food items. Ex: If the input is: M&M's10.034.02.01.0 where M&M's is the food name, 10.0 is the grams of fat, 34.0 is the grams of carbohydrates, 2.0 is the grams of protein, and 1.0 is the number of servings, the output is:…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
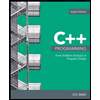
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning