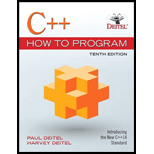
Concept explainers
Exercises11.3 (Composition as an Alternative to Inheritance) Many

Program Plan:
We will implement the BasePlusCommissionEmp class using composition instead of inheritance and invoke different functions in the test program subsequently.
Explanation of Solution
Explanation:
Program Description:
The program demonstrates composition as an alternate way of implementing functionality in Object oriented programming. Some of the pros and cons are self evident in the program, yet we’ll discuss the merits and demerits of using composition over inheritance herewith. As obvious, composition increases duplicacy of code as seen in BasePlusCommissionEmp class where a large part of attributes and functions of the Employee class have to be repeated in the BasePlusCommissionEmp class. Also, the test code or the actual application using these objects becomes more complicated because the use of Data structures like Vectors to store all similar objects together and invoke common functionality in a single loop gets limited. If there are a large number of objects with similar functionality and a little variances, the redundant code soon becomes prone to defect and maintenance nightmares. On the same hand, composition provides more control at the compile time by limiting common access modifier errors and methos overriding errors during development time. has-a relationship is suited mostly where there is limited or no commonality in attributes and functionality of the objects being modelled. Its always better to create an is-a classheirarchywhenthe objects being modelled are having a lot of common attributes and method, resulting in a generic common subset (the base class) and other derived class specializing form it. Inheritance makes the code cleaner to write, read and maintain.
Program:
// BasePlusCommissionEmp class definition . #ifndef BP_COMMISSION_H #define BP_COMMISSION_H #include<string>// C++ standard string class usingnamespace std; classBasePlusCommissionEmp { public: BasePlusCommissionEmp(conststring&, conststring&, conststring&, double = 0.0,double = 0.0, double = 0.0 ); //For generic attributes of Employee voidsetFirstName( conststring& ); // set first name stringgetFirstName() const; // return first name voidsetLastName( conststring& ); // set last name stringgetLastName() const; // return last name voidsetSocialSecurityNumber( conststring& ); // set SSN stringgetSocialSecurityNumber() const; // return SSN // additional functions for attributes of CommisionEmployee voidsetGrossSales( double ); // set gross sales amount doublegetGrossSales() const; // return gross sales amount voidsetCommissionRate( double ); // set commission rate doublegetCommissionRate() const; // return commission rate //additional functions for baseSalary voidsetBaseSalary( double ); // set base salary doublegetBaseSalary() const; // return base salary // Generic functions of Employee doubleearnings() const; voidprint() const; private: //Generic attributes of Employee stringfirstName; // composition: member object stringlastName; // composition: member object stringsocialSecurityNumber; //composition: member object //attributes of CommisionEmployee doublegrossSales; // gross weekly sales doublecommissionRate; // commission percentage //attribute for BaseSalary doublebaseSalary; // base salary }; // end class BasePlusCommissionEmp #endif BasePlusCommisionEmp.cpp /* BasePlusCommissionEmp.cpp using composition Created on: 31-Jul-2018 :rajesh@acroknacks.com */ #include<string>// C++ standard string class #include"BasePlusCommissionEmp.h" #include<iostream> usingnamespace std; BasePlusCommissionEmp::BasePlusCommissionEmp(conststring&fname, conststring&lname, conststring&ssn1, doublebaseSalary, doublegrossSales , doublecomRate ) :firstName (fname), lastName ( lname),socialSecurityNumber (ssn1 ) { setBaseSalary(baseSalary ); // validate and store base salary setGrossSales(grossSales);//validate and store gross sales setCommissionRate(comRate);//validate and store commision rate }// end constructor /&Functions Below are specific to This class */ // set gross sales amount voidBasePlusCommissionEmp::setGrossSales( double sales ) { if ( sales **gt;= 0.0 ) grossSales = sales; else throwinvalid_argument( "Gross sales must be >= 0.0" ); } // end function setGrossSales // return gross sales amount doubleBasePlusCommissionEmp::getGrossSales() const { returngrossSales; } // end function getGrossSales // set commission rate voidBasePlusCommissionEmp::setCommissionRate( double rate ) { if ( rate > 0.0 && rate < 1.0 ) commissionRate = rate; else throwinvalid_argument( "Commission rate must be > 0.0 and < 1.0" ); } // end function setCommissionRate doubleBasePlusCommissionEmp::getCommissionRate() const { returncommissionRate; } // end function getCommissionRate voidBasePlusCommissionEmp::setBaseSalary( double salary ) { if ( salary >= 0.0 ) baseSalary = salary; else throwinvalid_argument( "Salary must be >= 0.0" ); } // end function setBaseSalary // return base salary doubleBasePlusCommissionEmp::getBaseSalary() const { returnbaseSalary; } // end function getBaseSalary //compute earnings doubleBasePlusCommissionEmp::earnings() const { return ( (getCommissionRate() * getGrossSales()) + getBaseSalary()) ; } // end function earnings // print CommissionEmployee object voidBasePlusCommissionEmp::print() const { cout<<"\nBasePlusCommission employee: "; cout<<lastName<<", "<<firstName<<endl; cout<<"SSN : "<<socialSecurityNumber<<endl; cout<<"\n gross sales: $ "<<getGrossSales() <<"\n Base Salary: $ "<<getBaseSalary() <<"\n commission rate: "<<getCommissionRate() ; } // end function print /&Generic Employee functions **/ // set first name voidBasePlusCommissionEmp::setFirstName( conststring**first ) { firstName = first; // should validate } // end function setFirstName // return first name stringBasePlusCommissionEmp::getFirstName() const { returnfirstName; } // end function getFirstName // set last name voidBasePlusCommissionEmp::setLastName( conststring&last ) { lastName = last; // should validate } // end function setLastName // return last name stringBasePlusCommissionEmp::getLastName() const { returnlastName; } // end function getLastName // set social security number voidBasePlusCommissionEmp::setSocialSecurityNumber( conststring&ssn ) { socialSecurityNumber = ssn; // should validate } // end function setSocialSecurityNumber // return social security number stringBasePlusCommissionEmp::getSocialSecurityNumber() const { returnsocialSecurityNumber; } // end function getSocialSecurityNumber Test Program // Testing class BasePlusCommissionEmp. #include<iostream> #include<iomanip> #include"BasePlusCommissionEmp.h"// BasePlusCommissionEmp class definition usingnamespace std; intmain() { // instantiate a BasePlusCommissionEmp object BasePlusCommissionEmpemployee("Sue", "Jones", "222-22-2222",1500,10000,0.16 ); // get commission employee data cout<<"Employee information obtained by get functions: \n" <<"\nFirst name is "<<employee.getFirstName() <<"\nLast name is "<<employee.getLastName() <<"\nSocial security number is " <<employee.getSocialSecurityNumber() <<"\nBase Salary is $"<<employee.getBaseSalary() <<"\nGross sales is $"<<employee.getGrossSales() <<"\nCommission rate is $"<<employee.getCommissionRate() <<endl; cout<<"Earnings based on current Data : $"<<employee.earnings(); //Modify Sales data employee.setGrossSales( 8000 ); // set gross sales employee.setCommissionRate( .1 ); // set commission rate cout<<"\nUpdated employee information output by print function: \n" <<endl; employee.print(); // display the new employee information // display the employee's earnings cout<<"\n\n Updated Employee's earnings: $"<<employee.earnings() <<endl; } // end main
Employee information obtained by get functions:
First name is Sue
Last name is Jones
Social security number is 222-22-2222
Base Salary is $1500
Gross sales is $10000
Commission rate is $0.16
Earnings based on current Data : $3100
Updated employee information output by print function:
BasePlusCommission employee: Jones, Sue
SSN : 222-22-2222
gross sales: $ 8000
Base Salary: $ 1500
commission rate: 0.1
Updated Employee's earnings: $2300
Want to see more full solutions like this?
Chapter 11 Solutions
C++ How to Program (10th Edition)
- Tape for a Turing Machine (Doubly-linked List)”.(I USE ECLIPSE & RUN CHECK ONLINE CONSOLE)A class named Tape to represent Turing machine tapes. The class should have an instance variable of type Cell that points to the current cell with the method: public Cell getCurrentCell() -- returns the pointer that points to the current cell. public char getContent() -- returns the char from the current cell. public void setContent(char ch) -- changes the char in the current cell to the specified value. Don't forget the Javadoc comments. IT WOULD HAVE A PUBLIC CLASS public void moveLeft() -- moves the current cell one position to the left along the tape. Note that if the current cell is the leftmost cell that exists, then a new cell must be created and added to the tape at the left of the current cell, and then the current cell pointer can be moved to point to the new cell. The content of the new cell should be a blank space. (Remember that the Turing machine's tape is conceptually…arrow_forwardComputer Science Exercise 1 (java)– Horses .Create a class Horse containing the attributes name, color and year of birth. Implement getters and setters for the member variables Implement a constructor Create the classes (a) Racehorse and (b) Workhorse. In the case (a), you must add field a field holding the number of races won. In the case (b), add a field that contains the number of years the horse has been working. Write the appropriate getters and setters. Test your classesarrow_forwardLocalResource(String date, String sector)- Actions of the constructor include accepting a date in the format “dd/mm/yyyy”, initializing the base class, storing the sector and storing an id as a consecutively increasing integer. Note that the constructor must ensure that the date of birth information is recorded. b. getId():Integer - returns the ID of the current instance of LocalResource c. getSector():String – returns the sector associated with the current instance of localResource d. getTRN():String – returns the trn number of the current instance of LocalResource. The process used to determine the TRN is to add the id to the number 100000000 and then returning the string equivalent. e. Update the NineToFiver class to ensure it properly extends the LocalResource class f. In method getContact() of NineToFiver, remove the comments so that the method returns "Local Employee #"+and the id of the contact. Write a concrete class LocalConsultant that extends LocalResource, and implements…arrow_forward
- Computer Science There are clearly some similarities in the implementations of Taxi and Shuttle that suggest use of inheritance to represent them. Introducing inheritance is the primary task of this assessment. -The Ezcab class, The Ezcab class maintains separate lists of taxis and shuttles and destinations with destination fares (you can hardcode destination fares). It has a lookup method that searches for a taxi/shuttle with a given ID. -The Taxi and Shuttle classes share some common attributes – location and destination. They also have some common methods – getLocation, getDestination, getStatus and setDestination. -Vehicle is the superclass of both Taxi and Shuttle. This class involves placing the common fields and methods into Vehicle and removing them from Taxi and Shuttle. -Modify Taxi and Shuttle class to indicate that it is a subclass of Vehicle. You can keep the id field in Vehicle class and getId,SetId methods. -Arrange for Taxi's constructor to call the constructor of…arrow_forwardInheritance, Polymorphism, ArrayLists, Throwing Exceptions The UML diagram below shows a set of classes designed to represent a music collection from 1995. The constructors and methods all function in the standard way, except: The equipmentRequired method should return “Record Player” or “CD Player” as appropriate.The getAlbum method of the NinetiesMusicCollection class accepts an index and returns the corresponding Album object. This method throws an IllegalArgumentException if the index is out of range. (This is the only exception you have to throw anywhere in your code.)In the NinetiesMusicCollection constructor, you can assume the ArrayList<Album> object passed as an argument is not null . Don’t worry about privacy leaks.Implement this set of classes in Java. Note the italics on the Class name “Album” and the method name “equipmentRequired” in the Album class.arrow_forwardPython OOP (Check pictures for instructions)Code Template to be used in this link:https://pastebin.com/aikCrFyjarrow_forward
- Java (derived classes) - Pet Informationarrow_forward1.Use inheritance and classes to represent a deck playing cards. Create a Card class that stores the suit (e.g., Clubs, Diamonds, Hearts, Spades) and name (e.g., Ace, 2, 10, Jack) of each card along with appropriate accessors, constructors, and mutators.2.Next, create a Deck class that stores an ArrayList of Card objects. The default constructor should create objects that represent the standard 52 cards and store them in the ArrayList. The Deck class should have methods to do the following: Print every card in the deck. Shuffle the cards in the deck. You can implement this by randomly swappingevery card in the deck. Add a new card to the deck. This method should take a Card object as a parameter and add it to the ArrayList. Remove a card from the deck. This removes the first card stored in the ArrayList and returns it. Sort the cards in the deck ordered by name. 3.Next, create a Hand class that represents the cards in a hand. Hand should be derived from Deck. This is…arrow_forwardUse inheritance and classes to represent a deck of playing cards. Create a Card class that stores the suit (Clubs, Diamonds, Hearts, Spades) and name (e.g., Ace, 2, 10, Jack) along with appropriate accessors, constructors, and mutators. Next, create a Deck class that stores a vector of Card objects. The default constructor should create objects that represent the standard 52 cards and store them in the vector. The Deck class should have functions to:■ Print every card in the deck.■ Shuffle the cards in the deck. You can implement this by randomly swapping every card in the deck.■ Add a new card to the deck. This function should take a Card object as aparameter and add it to the vector.■ Remove a card from the deck. This removes the first card stored in the vector and returns it.■ Sort the cards in the deck ordered by name. Next, create a Hand class that represents the cards in a hand. Hand should be derived from Deck. This is because a hand is like a specialized version of a deck; we…arrow_forward
- Briefly answer the following: Consider the Ben Ten character and his transformation into different Aliens, briefly discuss which OOP pillar may implement over this character. Inheritance is used for code reusability, discuss what makes difference between the “has a” relationship and “is a” relationship. List with the real-life examples. Java does not support multiple inheritance using classes due to diamond problem. Briefly explain diamond problem with code example. Differentiate among the static, final, and finally keyword. As C++ uses destructor to release space of unused object, discuss how java reclaims space from unused objects. How would you throw custom exception? How would you re-throw exception? Discuss with code example Differentiate between checked and unchecked exception. Briefly explain with real life scenario.arrow_forwardSolve:arrow_forwardCan a class be a super class and a sub-class at the same time? Give example.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
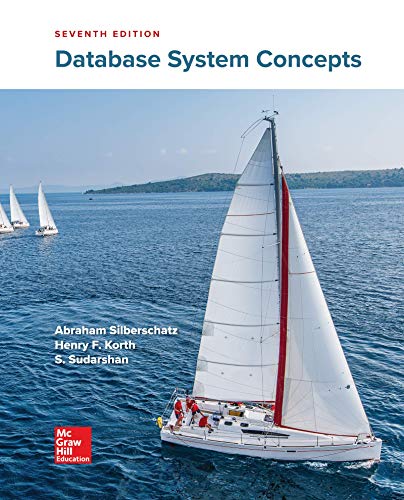
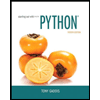
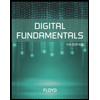
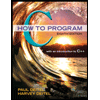
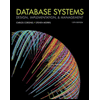
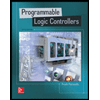