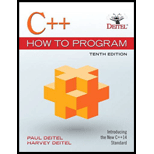
C++ How to Program (Early Objects Version)
10th Edition
ISBN: 9780134448824
Author: Paul Deitel; Harvey M. Deitel
Publisher: Pearson Education (US)
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Protected vs. Private Base Classes) Some programmers prefer not to use protected accessbecause they believe it breaks the encapsulation of the base class. Discuss the relative merits of usingprotected access vs. using private access in base classes.
(java)
The Painting Subclass
Write class as follows:
The class is named Painting, and it inherits from the Art class.
It has a private String member variable named medium
It has a default constructor that assigns the values "No name" to name, "No artist" to artist, -1 to year, and "No medium" to the medium variable. This default constructor calls the four-argument constructor.
It has a four-argument constructor to assign values to the name, artist, year, and medium variables.
It has a getter and settersfor the medium variable.
It has a toString() method
This class contains no other methods
Make sure to include your name, the name of this class, our course number, and the Activity number in a Javadoc comment at the top.
Make sure to write a Javadoc comment for each of these methods.
(Java)
The Sculpture Subclass
Write class as follows:
The class is named Sculpture, and it inherits from the Painting class.
It has a private boolean member variable named humanForm
It has a default constructor that assigns the values "No name" to name, "No artist" to artist, -1 to the year, "No medium" to the medium, and false to the humanForm variable. This default constructor calls the five argument constructor.
It has a five-argument constructor to assign values to the name, artist, year, medium, and humanForm variables.
It has a getter and setter for the humanForm variable.
It has a toString() method.
This class contains no other methods
Make sure to include your name, the name of this class, our course number, and the Activity number in a Javadoc comment at the top.
Make sure to write a Javadoc comment for each of these methods.
Chapter 11 Solutions
C++ How to Program (Early Objects Version)
Ch. 11 - Exercises11.3 (Composition as an Alternative to...Ch. 11 - (Inheritance Advantage) Discuss the ways in which...Ch. 11 - (Protected vs. Private Base Classes) Some...Ch. 11 - Prob. 11.6ECh. 11 - Prob. 11.7ECh. 11 - (Quadrilateral Inheritance Hierarchy) Draw an...Ch. 11 - Package Inheritance Hierarchy} Package-delivery...Ch. 11 - (Account Inheritance Hierarchy) Create an...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (Java) The Abstract Art Class Write an abstract class as follows: The class is named Art It inherits from the Comparable interface It has a private String member variable named name It has a private String member variable named artist It has a private int member variable called year It has a default constructor that assigns the values "No name" to name, "No artist" to artist and -1 to the year. This default constructor calls the three argument constructor. It has a three-argument constructor to assign values to the name, artist and year variables. It has a copy constructor that makes a copy of another non-null Art object It has getters and setters for the name, artist and year variables It has a toString() method that creates a string of artist, with name and year tabbed once on subsequent lines (see sample output) It has an equals method that compares this Art to another Object It has a compareTo method that compares in this order: 1) artist, 2) name, 3) year This class contains no…arrow_forward(The Time class) Design a class named Time. The class contains: - The data fields hour, minute, and second that represent a time. - A no-arg constructor that creates a Time object for the current time. (The values of the data fields will represent the current time.) -A constructor that constructs a Time object with a specified elapsed time since midnight, January 1, 1970, in milliseconds. (The values of the data fields will represent this time.) -A constructor that constructs a Time object with the specified hour, minute, and second. - Three getter methods for the data fields hour, minute, and second, respectively. -A method named setTime (long elapseTime) that sets a new time for the object using the elapsed time. For example, if the elapsed time is 555550000 milliseconds, the hour is 10, the minute is 19, and the second is 10. Draw the UML diagram for the class and then implement the class. Write a test program that creates two Time objects (using new Time (), new Time(555550000),…arrow_forwardProblem (Online Address Book): Using classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType with following members and methods: firstname, lastname, and accessors and mutators, print to display, and constructors. Define a class dateType for month, day and year as members with its accessors, mutators, and constructors) Design a class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information.…arrow_forward
- (JAVA) Create a class hierarchy to represent the different types of employees in an office who have the following job titles: manager, assistant, salesperson. Write an abstract base class called Employee that declares an abstract method: signature double calculateSalary() This class must also define the following attributes: name (String type), enrollment (String type) and base_salary (double type). Use encapsulation and provide a constructor that receives the corresponding values to be stored in the respective attributes. This abstract class must be extended by the other classes representing the types of employees, so the classes Manager, Assistant and Salesperson must be written. In each class you must override the method calculateSalario so that the salary calculation is done like this: The manager receives twice the base_salary, the assistant receives the base_salary and the salesperson receives the base_salary plus a commission defined in the constructor of his class. Create a…arrow_forward(The Person, Student, Employee, Faculty, and Staff classes) Design a class named Person and its two subclasses named Student and Employee. Make Faculty and Staff subclasses of Employee. A person has a name, address, phone number, and e-mail address. A student has a class status (freshman, sophomore, junior, or senior). Define the status as a constant. An employee has an office, salary, and date hired. Use the MyDate class defined in Programming Exercise 10.14 – see the textbook - to create an object for date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString method in each class to display the class name and the person's name. Draw the UML diagram for the classes and implement them. Write a test program that creates a Person, Student, Employee, Faculty, and Staff, and invokes their toString() methods.arrow_forward(Inheriting Interface vs. Inheriting Implementation) A derived class can inherit “interface” or “implementation” from a base class. How do inheritance hierarchies designed for inheriting interface differ from those designed for inheriting implementation?arrow_forward
- (Account class)Create an Account class that a bank might use to represent customers’ bank accounts. Include a data member of type int to represent the account balance. Provide a constructor that receives an initial balance and uses it to initialize the data member. The constructor should validate the initial balance to ensure that it’s greater than or equal to 0. If not, set the balance to 0 and display an error message indicating that the initial balance was invalid. Provide three member functions. Member function credit should add an amount to the current balance. Member function debit should withdraw money from the Account and ensure that the debit amount does not exceed the Account’s balance. If it does, the balance should be left unchanged, and the function should print a message indicating "Debit amount exceeded account balance." Member function get_Balance should return the current balance. Create a program that creates two Account objects and tests the member functions of class…arrow_forward(Default Constructor) What’s a default constructor? How are an object’s data members initialized if a class has only an implicitly defined default constructor?arrow_forward(Person Class) Design a class named Person that contains: o name, gender, and personCase as a private attribute o Non-default constructor that specifies name and gender o toString method that returns person data o personCase () method with no implementation (Student Class) Design a class named Student which is a child of Person that contains: o studentID as a private attribute o Nondefault constructor that specifies the name, gender, and studentID o toString method that returns student data o Implement personCase () that assigns "Not Studying" to personCase if studentID equals 0, “Studying" in case studentID greater than 0, and "Not a student" in case studentID less than 0. (Employee Class) Design a class named Employee which is a child of Person that contains: o employeelD as a private attribute o Non-default constructor that specifies the name, gender, and employeelD o toString method that returns employee data o Implement personCase () that assign “Technical" to personCase if…arrow_forward
- (Composition as an Alternative to Inheritance) Many programs written with inheritancecould be written with composition instead, and vice versa. Rewrite class BasePlusCommissionEmployee of the CommissionEmployee–BasePlusCommissionEmployee hierarchy to use compositionrather than inheritance. After you do this, assess the relative merits of the two approaches for designing classes CommissionEmployee and BasePlusCommissionEmployee, as well as for object-oriented programs in general. Which approach is more natural? Why?arrow_forward(JAVA) The use case description for the View Exam Grades use case is described below. Determineclasses that are needed to implement the use case. Also, define each class's attributes andmethods. Represent class and its attributes and methods using the UML class notation.Use case name: View Exam Grades.Summary: The student looks at his/her exam grade of CS2365 OOP or CS3365 SoftwareEngineering.Actor: StudentPrecondition: None.Main sequence:1. The student enters the student ID and password into the system.2. The system checks if the student ID and password are valid.3. The system displays the course numbers (e.g., CS2365 and CS3365) if the ID andpassword are valid.4. The customer chooses only a course to view the grade.5. The system displays the course grade to the student.Alternative sequence:Step 3: If the student's ID and password are invalid, the system displays an error messageand asks to enter another ID and password into the system. If the ID and password arevalid, the system…arrow_forward(The Account class) Account -id: int -balance: double -annualInterestRate : double -dateCreated : Date +Account( ) +Account(someId : int, someBalance : double) +getId() : int +setId(newId : int) : void +getBalance() : double +setBalance(newBalance : double) : void +getAnnualInterestRate( ) : double +setAnnualInterestRate(newRate : double) : void +getDateCreated( ) : Date +getMonthlyInterestRate( ) : double +getMonthlyInterest( ) : double +withdraw(amt : double) : void +deposit(amt : double) : void Design a class named Account that contains: ■ A private int data field named id for the account (default 0). ■ A private double data field named balance for the account (default 0). ■ A private double data field named annualInterestRate that stores the current interest rate (default 0). Assume all accounts have the same interest rate. Make it static. ■ A private Date data field named dateCreated that stores the date when the account was created. (private Date dateCreated)…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
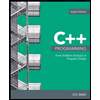
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
What is Abstract Data Types(ADT) in Data Structures ? | with Example; Author: Simple Snippets;https://www.youtube.com/watch?v=n0e27Cpc88E;License: Standard YouTube License, CC-BY