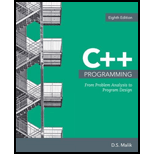
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN: 9781337102087
Author: D. S. Malik
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 7PE
Program Plan Intro
Program Plan:
- Declare a class dayType to store a day and to check the day number is valid or not. If it is valid, then go to the next function. Otherwise print the message "Day number should be in between 0 and 6."
- Implement the main function that tests all constructors and functions in the class dayType.
- Implement set function that accepts a day as a parameter then verifies whether the day is valid and sets that day as the current day.
- Implement print function that accepts a day as an integer parameter and displays that day as a string.
- Implement getDay function that returns the current day as an integer.
- Implement next_day function that returns the next day to the current day as an integer.
- Implement prev_day function that returns the previous day to the current day as an integer.
- Implement a default constructor that sets 0 to the current day as Sun (Sunday).
- Implement parameterized constructor that sets the specified day to the current day.
Program Description: The purpose of the program is to implement the days of the week. It stores the day such as Sunday and it performs the specified operations.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Show all the work
Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.
Show all the work
Chapter 10 Solutions
C++ Programming: From Problem Analysis to Program Design
Ch. 10 - Mark the following statements as true or false....Ch. 10 - Find the syntax errors in the following class...Ch. 10 - Find the syntax errors in the following class...Ch. 10 -
Find the syntax errors in the following class...Ch. 10 -
Find the syntax errors in the following class...Ch. 10 - Prob. 6SACh. 10 - Assume the definition of class foodType as given...Ch. 10 -
Consider the definition of the following class:...Ch. 10 - Consider the definition of the class product Type...Ch. 10 -
Consider the definition of the class product Type...
Ch. 10 - Prob. 11SACh. 10 -
Assume the definition of class houseType as given...Ch. 10 - Chapter 9 defined the struct studentType to...Ch. 10 - Write a program that uses the class productType...Ch. 10 -
Write a program that uses the class houseType...Ch. 10 - Prob. 6PECh. 10 - Prob. 7PECh. 10 - Some of the characteristics of a book are the...Ch. 10 - Define the class bankAccount to implement the...
Knowledge Booster
Similar questions
- Show all the workarrow_forwardShow all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forward
- Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forward
- I need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forwardNeed help completing this algorithm here in coding! 2arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
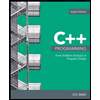
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
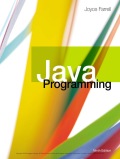
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
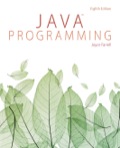
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
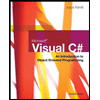
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
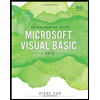
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage