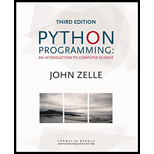
Three Button Monte
Program Plan:
- Import the required packages.
- Declare a main function. Inside the main function,
- Create the application window.
- Draw the interface widget by creating three buttons.
- Assign the text to the interface.
- Call the method “draw()”.
- Get the random number.
- Assign the values to “false”.
- Check the condition.
- Assign “point1” to “true”.
- Check the condition.
- Assign the “point2” to “true”.
- Otherwise, Assign the “point3” to “true”
- Activate all the three doors.
- Get the action mouse clicked.
- Check the condition using “while” loop.
- Check the condition for selecting the door 1 to be clicked.
- Check the condition for selecting the door 2 to be clicked.
- Check the condition for selecting the door 3 to be clicked.
- Call the “getMouse()” function
- Call the main function.

Explanation of Solution
Program:
Refer the program “button.py” given in the “Chapter 10” from the text book. Add the method “update()” along with the given code.
#Define the method update
def update(self, win, label):
#Call the method undraw()
self.label.undraw()
#Assign the position to centre
center = self.center
#Assign the label
self.label = Text(center, label)
#Set active to false
self.active = False
#Call the method draw()
self.label.draw(win)
Main.py:
#Import the required packages
from button import Button
from graphics import GraphWin, Point, Text
from random import random
#Definition of main method
def main():
#Creating the application window by setting title, cords and background
win = GraphWin("Three Button Monte", 500, 300)
win.setCoords(-12, -12, 12, 12)
win.setBackground("green3")
#Draw the interface widget by creating three button
door1 = Button(win, Point(-7.5, -3), 5, 6, "Door 1")
door2 = Button(win, Point(0, -3), 5, 6, "Door 2")
door3 = Button(win, Point(7.5, -3), 5, 6, "Door 3")
#Assign the text to the interface
direction = Text(Point(0, 10), "Pick a Door")
#Call the method draw
direction.draw(win)
#Get the random number
x = random() * 3
#Assign the values to false
point1 = point2 = point3 = False
#check the condition
if 0 <= x <1:
#Assign point1 to True
point1 = True
#Chcek the condition
elif 1 <= x < 2:
#Assign the point2 to True
point2 = True
#Otherwise
else:
#Assign the point3 to True
point3 = True
#Activate all the three doors
door1.activate()
door2.activate()
door3.activate()
#Get the action mouse click
click = win.getMouse()
#Check the condition using "while" loop
while door1.clicked(click) or door2.clicked(click) or door3.clicked(click):
#If door1 is clicked
if door1.clicked(click):
#Assign point1 to true
if point1 == True:
#Update the interface
door1.update(win,"Victory!")
else:
#If door1 is clicked
if door2.clicked(click):
#Update the interface
door2.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door3.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
#If door2 is clicked
elif door2.clicked(click):
#Assign point2 to true
if point2 == True:
#Update the interface
door2.update(win,"Victory!")
else:
#If door2 is clicked
if door3.clicked(click):
#Update the interface
door3.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door1.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
else:
#Assign point3 to true
if point3 == True:
#Update the interface
door3.update(win,"Victory!")
else:
#If door2 is clicked
if door2.clicked(click):
#Update the interface
door2.update(win,"Correct Door.")
#Get the action mouse click
click = win.getMouse()
else:
#Update the interface
door1.update(win,"Correct door.")
#Get the action mouse click
click = win.getMouse()
#Call the getMouse()
win.getMouse()
#Call the main function
main()
Output:
Screenshot of output
Screenshot of output
Want to see more full solutions like this?
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- This week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forwardIn completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward
- 1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward2. Consider the following pseudocode for partition: function partition (A,L,R) pivotkey = A [R] t = L for i L to R-1 inclusive: if A[i] A[i] t = t + 1 end if end for A [t] A[R] return t end function Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the indicated instances. Initial A After i=0 ends After 1 ends After i 2 ends After i = 3 ends After i = 4 ends After final swap 10 19 285 [12 pts]arrow_forward
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
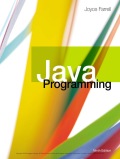
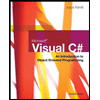
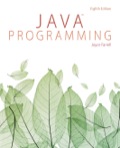
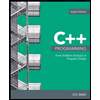
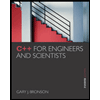