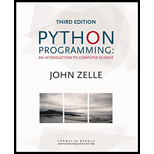
Face
Program Plan:
face.py
- Import the required packages.
- Definition of main “init” method.
- Assign the size value, eye size, eye off, mouth size, mouth off, window value to the corresponding variables.
- Definition of “getCenter” method.
- Return the center value.
- Definition of “move” method.
- Call the method “undrawn()”.
- Call the method getCenter().
- Get the position of “x” and “y”.
- Get the center position.
- Check the condition for the face to smile.
- Call the method “smile()”.
- Check the condition for the face to wink.
- Call the method “wink()”.
- Check the condition for the face to Grim Face.
- Call the method “GrimFace()”.
- Call the method “mediate()”.
- Check the condition for the face to wink.
- Call the method “smile()”.
- Definition “initializeGrimFace” method.
- Create a circle.
- Call the function to open the left eye and right eye.
- Create an array for teeth.
- Call the method to draw the mouth.
- Call the method to draw the face.
- Definition of method “lineMouth()”.
- Call the method “clone()”.
- Condition to set the mouth.
- Call the method “clone()”.
- Call the method “Line()”.
- Return the line.
- Definition of method “rectMouth()”
- Get the position to place the mouth.
- Return the position.
- Definition of method “undrawn()”.
- Remove the head, left eye, right eye, teeth and mouth.
- Definition of method “drawFace()”.
- Draw the circle to place the outline of the face.
- Set the head to the window.
- Set the left eye to the window.
- Set the left eye to the window.
- Set the mouth to the window.
- Definition of “leftEyeOpen()”.
- Set the left eye to the circle.
- Condition to open and close the left eyes.
- Definition of “rightEyeOpen()”.
- Set the right eye to the circle.
- Condition to open and close the right eyes.
- Definition of “leftEyeWink()” method.
- Place the position of the left eye.
- Get the values of “x” and “y”.
- Condition to get the “leftWink”.
- Set the value to “leftWink”.
- Definition of “rightEyeWink()” method.
- Place the position of the right eye.
- Get the values of “x” and “y”.
- Condition to get the “rightWink”.
- Set the value to “rightWink”.
- Definition of “wink()”.
- Call the method “unDraw()”.
- Call the method “lineMouth()”, leftEyeOpen(), “rightEyeWink()”, “drawFace()”.
- Definition of “smile()” method.
- Call the method “unDraw()”.
- Call the method “rectMouth()”.
- Get the “xint” value.
- Condition to append the mouth.
- Traverse the loop till “i” reaches “8”.
- Call the method “Line()” to get the two points.
- Condition to append the teeth.
- Check the condition to place the teeth.
- Draw the tooth for the face.
- Call the method “rectMouth()”., “leftEyeOpen()”, “rightEyeOpen()”, “drawFace()”.
- Definition of “mediate()” method.
- Call the respective method to un draw the face, draw mouth, right and left eye and face
- Definition of “mediate()” method.
Main.py:
- Import the required packages.
- Definition of method “makeButtons()”.
- Create a button “wink()”.
- Create a button “mediate()”.
- Create a button “Smile()”.
- Crate a button “Quit”.
- Activate all the buttons.
- Return the values.
- Definition of method “main()”.
- Creating the interface.
- Call the method “getMouse()”.
- Check whether the “endGame” is clicked or not.
- Check whether the “wink” is clicked.
- Call the method “wink”.
- Call the method “getMouse()”.
- Check whether the “smile” is clicked.
- Call the method “smile()”.
- Call the method “getMouse()”.
- Check whether the “mediate” is clicked.
- Call the method “mediate()”.
- Call the method “getMouse()”.
- Close the window.
- Call the method “main()”.
- Check whether the “wink” is clicked.

Explanation of Solution
Program:
face.py
#Import required packages
from graphics import *
#Definition of class Face
class Face:
#Definition of init method
def __init__(self, window, center, size):
#Assign the size value
self.size = size
#Calculate the eye size
self.eyeSize = 0.15 * size
#Condition to close the eye
self.eyeOff = size / 3.0
#Condition of mouth size
self.mouthSize = 0.8 * size
#Condition to close the mouth
self.mouthOff = size / 2.0
#Set the interface to centre
self.center = center
#Assign the window value
self.window = window
#Call the method initializeGrimFace
self.initializeGrimFace()
#Assign the rightEye
self.rightEye
#Definition of getCenter method
def getCenter(self):
#Return the centre value
return self.center
#Definition of method move
def move(self, dx, dy):
#Call the method undraw
self.unDraw()
#Call the method getCenter
center = self.getCenter()
#Get the position of x and y
x = center.getX()
y = center.getY()
#Get the centre position
self.center = Point(x + dx, y + dy)
#Check the condition for the face to simile
if dx < 0 and dy < 0:
#Call the smile method
self.smile()
#Check the condition for the face to wink
elif dx < 0 and dy > 1:
#Call the method wink
self.wink()
#Check the condition for GrimFace
elif dx > 0 and dy > 0:
#Call the method initializeGrimFace()
self.initializeGrimFace()
#Otherwise, call the method mediate()
else:
self.meditate()
#Definition of method initializeGrimFace
def initializeGrimFace(self):
#Create a circle
self.head = Circle(self.center, self.size)
#Call the function to open the left eye
self.leftEyeOpen()
#Call the function to open the right eye
self.rightEyeOpen()
#Create an array for teeth
self.teeth = []
#Call the method to draw the mouth
self.lineMouth()
#Call the method to draw the face
self.drawFace()
#Definition of method lineMouth
def lineMouth(self):
#Call the method clone()
p1 = self.center.clone()
#Condition to set the mouth
p1.move(-self.mouthSize/2, self.mouthOff)
#Call the method clone
p2 = self.center.clone()
#Condition to set the mouth
p2.move(self.mouthSize/2, self.mouthOff)
#Call the method Line()
self.mouth = Line(p1, p2)
#Return the line
return Line(p1, p2)
#Definition of method rectMouth()
def rectMouth(self):
#Get the position to place the mouth
p1, p2 = self.mouth.getP1(), self.mouth.getP2()
x1, x2, y1, y2 = p1.getX(), p2.getX(), p1.getY(), p2.getY()
offset = self.eyeSize / 2
self.mouth = Rectangle(Point(x1, y1 - offset), Point(x2, y2 + offset))
#Return the position
return x2, x1, y1, y2, offset
#Definition of method unDraw()
def unDraw(self):
#Remove the head, left eye, right eye, teeth and mouth
self.head.undraw()
self.leftEye.undraw()
self.rightEye.undraw()
self.mouth.undraw()
for tooth in self.teeth:
tooth.undraw()
#Definition of method drawFace()
def drawFace(self):
#Draw the circle to place the outline of the face
self.head = Circle(self.center, self.size)
#Set the head to the window
self.head.draw(self.window)
#Set the left eye to the window
self.leftEye.draw(self.window)
#Set the right eye to the window
self.rightEye.draw(self.window)
#Set the mouth to the window
self.mouth.draw(self.window)
#Definition of leftEyeOpen()
def leftEyeOpen(self):
#Set the left eye to the circle
self.leftEye = Circle(self.center, self.eyeSize)
#condition to open and close the left eyes
self.leftEye.move(-self.eyeOff, -self.eyeOff)
#Definition of rightEyeOpen()
def rightEyeOpen(self):
#Set the right eye to the circle
self.rightEye = Circle(self.center, self.eyeSize)
#Condition to open and close the right eyes
self.rightEye.move(self.eyeOff, -self.eyeOff)
#Definition of leftEyeWink()
def leftEyeWink(self):
#Place the position of the left eye
center = self.leftEye.getCenter()
#Get the x and y value
x = center.getX()
y = center.getY()
#Condition to get the leftWink
leftWink = Line(Point(x - self.eyeSize, y), Point(x + self.eyeSize, y))
#Set the value leftWink
self.leftEye = leftWink
#Definition of rightEyeWink()
def rightEyeWink(self):
#Place the position of the right eye
center = self.rightEye.getCenter()
#Get the x and y value
x = center.getX()
y = center.getY()
#Condition to get the rightWink
rightWink = Line(Point(x - self.eyeSize, y), Point(x + self.eyeSize, y))
#Set the value rightEye
self.rightEye = rightWink
#Definition of wink()
def wink(self):
#Call the method unDraw()
self.unDraw()
#Call the method lineMouth(), leftEyeOpen(), rightEyeWink(), drawFace()
self.lineMouth()
self.leftEyeOpen()
self.rightEyeWink()
self.drawFace()
#Definition of method smile()
def smile(self):
#Call the method unDraw()
self.unDraw()
#Call the method rectMouth()
x2, x1, y1, y2, offset = self.rectMouth()
#Get xint value
xint = abs(x2 - x1) / 8
#Condition to append the mouth
self.teeth.append(self.lineMouth())
#Traverse the loop till i reaches 8
for i in range (8):
#Call the method Line() to get the two points
t2 = Line(Point(x1 + i * xint, y1 - offset), Point((x1 + i * xint), y2 + offset))
#Condition to append the teeth
self.teeth.append(t2)
#Check the condition to place the teeth
for tooth in self.teeth:
#Draw the tooth for the face
tooth.draw(self.window)
#Call the method reactMouth(), leftEyeOpen(), rightEyeOpen(), drawFace()
self.rectMouth()
self.leftEyeOpen()
self.rightEyeOpen()
self.drawFace()
#Definition of method mediate()
def meditate(self):
#Call the respective method to undraw the face, draw mouth, right and left eye and face
self.unDraw()
self.lineMouth()
self.leftEyeWink()
self.rightEyeWink()
self.drawFace()
Cbutton.py:
Refer the program “button.py” given in the “Chapter 10” from the text book. Add the method “update()” along with the given code.
#Define the method update
def update(self, win, label):
#Call the method undraw()
self.label.undraw()
#Assign the position to centre
center = self.center
#Assign the label
self.label = Text(center, label)
#Set active to false
self.active = False
#Call the method draw()
self.label.draw(win)
Main.py
#Import the required packages
from face import Face
from graphics import *
from cbutton import CButton
#Definition of method makeButton(0
def makeButtons(win):
#Create a button Wink
wink = CButton(win, Point(16, 17), 1, "Wink")
#Create a button Meditate
meditate = CButton(win, Point(12, 17), 1, "Meditate")
#Create a button Smile
smile = CButton(win, Point(8, 17), 1, "Smile")
#Create a button Quit
endGame = CButton(win, Point(4, 17), 1, "Quit")
#Activate all the buttons
wink.activate()
meditate.activate()
smile.activate()
endGame.activate()
#Return the values
return wink, meditate, smile, endGame
#Definition of method main()
def main():
#Creating the interface
win = GraphWin("Emoji Jawn", 600, 600)
win.setCoords(20, 20, 0, 0)
face = Face(win, Point(10,8), 7)
wink, meditate, smile, endGame = makeButtons(win)
#Call the method getMouse()
pt = win.getMouse()
#Check whether the endGame is clicked or not
while not endGame.clicked(pt):
#Check whther the Wink is clicked
if wink.clicked(pt):
#Call the method wink()
face.wink()
#Call the method getMouse()
pt = win.getMouse()
#Check whether the smile is clicked
elif smile.clicked(pt):
#Call the method smile()
face.smile()
#Call the getMouse() method
pt = win.getMouse()
#Check whether the meditate is clicked
elif meditate.clicked(pt):
#Call the method meditate()
face.meditate()
#Call the getMouse() method
pt = win.getMouse()
#Otherwise
else:
#Call the getMouse() method
pt = win.getMouse()
#close up shop
win.close()
#Call the method main()
main()
Output:
Screenshot of output
Clicking Wink button:
Screenshot of output
Clicking Mediate button:
Screenshot of output
Clicking Smile button:
Screenshot of output
Want to see more full solutions like this?
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
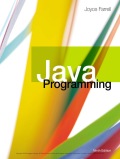
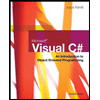
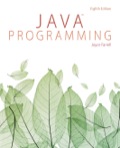
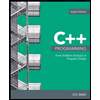
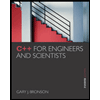