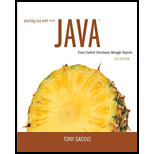
Concept explainers
Ship, CruiseShip, and CargoShip Classes
Design a Ship class that the following members:
- A field for the name of the ship (a string).
- A field for the year that the ship was built (a string).
- A constructor and appropriate accessors and mutators.
- A toString method that displays the ship’s name and the year it was built.
Design a CruiseShip class that extends the Ship class. The CruiseShip class should have the following members:
- A field for the maximum number of passengers (an int).
- A constructor and appropriate accessors and mutators.
- A toString method that overrides the toString method in the base class. The CruiseShip class’s toString method should display only the ship’s name and the maximum number of passengers.
Design a CargoShip class that extends the Ship class. The CargoShip class should have the following members:
- A field for the cargo capacity in tonnage (an int).
- A constructor and appropriate accessors and mutators.
- A toString method that overrides the toString method in the base class. The CargoShip class’s toString method should display only the ship’s name and the ship’s cargo capacity.
Demonstrate the classes in a

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Starting Out with Java: From Control Structures through Objects (6th Edition)
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Concepts Of Programming Languages
Database Concepts (8th Edition)
Modern Database Management
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Degarmo's Materials And Processes In Manufacturing
- Capsim Team PowerPoint Presentations - Slide Title: Key LearningsWhat were the key learnings that you discovered as a team through your Capsim simulation?arrow_forwardWrite the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.arrow_forwardDraw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.arrow_forward
- Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.arrow_forward2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forward
- hello please explain the architecture in the diagram below. thanks youarrow_forwardComplete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
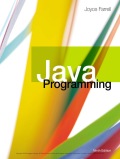
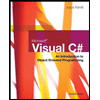
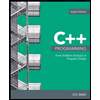
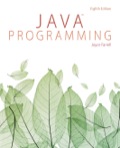
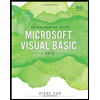