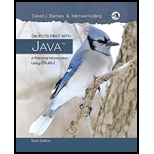
Add a second sun to the picture. To do this, pay attention to the field definitions close to the top of the class. You will find this code:
You need to add a line here for the second sun. For example:
Then write the appropriate code in two different places for creating the second sun and making it visible when the picture is drawn.

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Mechanics of Materials (10th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Starting Out with Python (4th Edition)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Create the VisualCounter class, which supports both increment and decrement operations. Take the constructor's two arguments N and max, where N indicates the maximum operation number and max specifies the counter's maximum absolute value. Make a plot that displays the value of the counter each time its tally changes as a byproduct.arrow_forwardIN JAVA LANGUAGE Write an ATM class with an ArrayList of Account objects as an attribute. In the constructor, add 3 Account objects to your ArrayList. They can all have a start balance of $100 and an annual interest rate of 0.12. Include two methods, menu and makeSelection as outlined below. Please note that since both methods get user input, create a Scanner attribute to use in both. menu method This method does not have any parameters and does not return a value. It should: Get the account number from the user. This corresponds to the index of the items in the ArrayList. Since there are 3 elements in your ArrayList, you are going to ask them for a number between 1 and 3, but keep in mind that the indices of the ArrayList are 0-2, so you'll have to adjust the value you get from the user accordingly. Present the user with a main menu as shown below: Get their menu selection Call the makeSelection method, passing it the account index obtained in step 1 and the menu selection…arrow_forwardIt's time for the opening quidditch match of the season! We represent the various positions for players with the QuidditchPlayer class and its subclasses. Every player begins with a base_energy level, but every position requires a different proportion of energy. Fill in the energy method for the Beater, Chaser, Seeker, and Keeper classes, according to their docstrings. In addition, fill in the init____ method for the Chaser class. class Beater (QuidditchPlayer): role="bludgers" def energy (self, time): ****** Returns the amount of energy left after playing for time minutes. After playing for time minutes, Beaters lose their base energy level divided by the number of minutes. If time is 0, catch the ZeroDivisionError and print "You can't divide by zero!" instead. >>> fred Beater ("Fred Weasley", 640) >>> fred.energy (40) 624.0 >>> fred.energy (0) You can't divide by zero! ***** "***** YOUR CODE HERE *****" Use OK to test your code: python3 ok -q Beater.energy class Chaser…arrow_forward
- Create two enumerations that hold colors and car modeltypes. You will use them as field types in a Car class and write ademonstration program that shows how the enumerations are used.arrow_forwardFor this lab task, you will work with classes and objects. Create a class named text that works similar to the built-in string class. You will need to define a constructor that can be used to initialize objects of this new class with some literal text. Next, define three methods: to_upper() that will convert all characters to uppercase, reverse() that will reverse the text and length() that will return the length of the text. After you have completed this part, copy the following mainfunction to your code and check if the implementation is correct. int main() { text sample = "This is a sample text"; cout << sample.to_upper(); // This should display "THIS IS A SAMPLE TEXT" cout << endl;cout << sample.reverse(); // This should display "txet elpmas a si sihT"cout << endl; cout << sample.length(); // This should display 21 }arrow_forwardFor this assignment, you are asked to create a ColorfulBouncingCircle class. ColorfulBouncingCircle should extend ColorfulCircle. Unlike the other Circle classes, it will include a velocity for the x and y axes. The constructor for ColorfulBouncingCircle includes these parameters:public ColorfulBouncingCircle(double radius, double centerX, double centerY, Color color, double xVelocity, double yVelocity) Note that we are treating x as increasing to the right and y as increasing down, as with Java’s graphics coordinates system. This was also seen with Circle’s draw method. ColorfulBouncingCircles will bounce around in a playing field which the tester will provide. The ColorfulBouncingCircle class should have a public static method to set the playing field width and height: public static void setPlayingFieldSize(double newWidth, double newHeight) ColorfulBouncingCircle will also include a method which should alter its centerX and centerY with each time tick (similar to a metronome’s…arrow_forward
- Write a program that asks the user for two points (x, y) and use them to create two Point2D objects. Then, use the methods in the Point2D class to determine and display the distance between the two points and the midpoint between the two points.arrow_forwardI think you forgot to apply encapsulation in classes where every data is editablearrow_forwardThe next sections will provide you with the definitions of the classes MonthCalender and DateTimerPicker respectively.arrow_forward
- Please help me create a cave class for a Hunt the Wumpus game (in java). You can read the rules in it's entirety of the Hunt the Wumpus game online to get a better idea of the specifications. It's an actual game. INFORMATION: The object of this game is to find and kill the Wumpus within a minimum number of moves, before you get exhausted or run out of arrows. There is only one way to win: you must discover which room the Wumpus is hiding in and kill it by shooting an arrow into that room from an adjacent room. The Cave The Wumpus lives in a cave of 30 rooms. The rooms are hexagonal. Each room has up to 3 tunnels, allowing access to 1, 2 or 3 (out of 6) adjacent rooms. The attached diagram shows the layout of rooms in the cave. The map wraps around such that rooms on the edges (white cells) have neighbors from the opposite edge (blue cells). E.g., the neighbors of room 1 are rooms 25, 26, 2, 7, 6, and 30, and you could choose to connect room 1 to any of these rooms. Observe how…arrow_forwardDesign a class named RoseBushes. A rose has fields for a color (for example, “red”), a price for athree gallon bush (for example, 17.99), and a field that indicates whether the rose bush hasbloomed (for example, “Yes”).Finish creating the class diagram below and then write the pseudocode that defines the class.Hint: You will need set and get methods for each field.UML Class Diagram:RoseBushes-color : string-price : num-bloomed : string Pseudocode:arrow_forwardEverything is in the attached picture! Thank you so much!arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
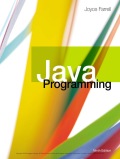