Your task in this question is to write a class called SkillTree which extends BasicMAryTree280 (an m-ary tree of Skill objects; a complete Skill.java is provided). A template for the SkillTree class is provided. It contains a constructor and a couple of useful methods. You will add additional methods to this class in the following steps, which you should complete in order: (a) Write a main() method in the SkillTree class in which you construct your own skill tree for your own hypothetical video game. Your tree must contain at least 10 skills. However, for the sanity of everyone involved, try to keep it under 15 skills. Be creative! There is no reason why any two students should hand in exactly the same (or even very similar) skill trees, nor should you just duplicate the skill tree shown in the sample output. Print your tree to the console using the toStringByLevel() method inherited from BasicMAryTree280. (b) Write a method in the SkillTree class called skillDependencies which takes a skill name as input and returns an instance of LinkedList280 which contains all the of the skills which are prerequisites for obtaining the input skill (including the input skill itself!). A RuntimeException exception should be thrown if the tree does not contain the given skill. A good implementation approach for this method is to use a recursive traversal of the tree to find the named skill, and then add skills to the output list as the recursion unwinds. Tutorial 3 includes some discussion of recursive traversal of m-ary trees. Add to your main() program a few tests of this method, and print out the lists that is returned (you can use the list's toString() method for this). Be sure to test the case where the named skill does not exist in the tree. (c) Write a method in the SkillTree class called skillTotalCost which takes a skill name as input and returns the total number of skill points that a player must invest to obtain the given skill. If the named skill is not in the skill tree, then the skillTotalCost method should throw a RuntimeException exception. Hint: this method is quite easy to implement if you make use of the previously implemented skillDependencies method. For example, in the above skill tree, if a character wants the Shield Ally skill they would need to spend 1 skill point to get Shield Proficiency, and then spend 3 skill points to get Shield Ally for an overall investment of 1 + 3 = 4 points, so for the above tree, skillTotalCost("Shield Ally") should return 4. Note that the Skill object contains the cost of the skill. Add to your main() program a few tests of skillTotalCost, and print out the total costs returned. Be sure to test the case where the named skill does not exist in the tree. (d) Run your main() program. Cut and paste the console output to a text file and submit it with your assignment. See the sample output below pictures are one of skilltree and the other is methods for skilltree
Your task in this question is to write a class called SkillTree which extends BasicMAryTree280 (an m-ary tree of Skill objects; a complete Skill.java is provided). A template for the SkillTree class is provided. It contains a constructor and a couple of useful methods. You will add additional methods to this class in the following steps, which you should complete in order: (a) Write a main() method in the SkillTree class in which you construct your own skill tree for your own hypothetical video game. Your tree must contain at least 10 skills. However, for the sanity of everyone involved, try to keep it under 15 skills. Be creative! There is no reason why any two students should hand in exactly the same (or even very similar) skill trees, nor should you just duplicate the skill tree shown in the sample output. Print your tree to the console using the toStringByLevel() method inherited from BasicMAryTree280. (b) Write a method in the SkillTree class called skillDependencies which takes a skill name as input and returns an instance of LinkedList280 which contains all the of the skills which are prerequisites for obtaining the input skill (including the input skill itself!). A RuntimeException exception should be thrown if the tree does not contain the given skill. A good implementation approach for this method is to use a recursive traversal of the tree to find the named skill, and then add skills to the output list as the recursion unwinds. Tutorial 3 includes some discussion of recursive traversal of m-ary trees. Add to your main() program a few tests of this method, and print out the lists that is returned (you can use the list's toString() method for this). Be sure to test the case where the named skill does not exist in the tree. (c) Write a method in the SkillTree class called skillTotalCost which takes a skill name as input and returns the total number of skill points that a player must invest to obtain the given skill. If the named skill is not in the skill tree, then the skillTotalCost method should throw a RuntimeException exception. Hint: this method is quite easy to implement if you make use of the previously implemented skillDependencies method. For example, in the above skill tree, if a character wants the Shield Ally skill they would need to spend 1 skill point to get Shield Proficiency, and then spend 3 skill points to get Shield Ally for an overall investment of 1 + 3 = 4 points, so for the above tree, skillTotalCost("Shield Ally") should return 4. Note that the Skill object contains the cost of the skill. Add to your main() program a few tests of skillTotalCost, and print out the total costs returned. Be sure to test the case where the named skill does not exist in the tree. (d) Run your main() program. Cut and paste the console output to a text file and submit it with your assignment. See the sample output below
pictures are one of skilltree and the other is methods for skilltree
![package lib280;
import lib280. tree. BasicMAryTree280;
2 usages
public class SkillTree extends BasicMAryTree280<Skill> {
0
}
/**
* Create lib280. tree with the specified root node and
* specified maximum arity of nodes.
* @timing 0(1)
* @param x item to set as the root node
* @param m number of children allowed for future nodes
*/
no usages
public Skill Tree (Skill x, int m) { super(x, m); }
/**
* A convenience method that avoids typecasts.
* Obtains a subtree of the root.
*
* @param i Index of the desired subtree of the root.
* @return the i-th subtree of the root.
*/
no usages
public Skill Tree rootSubTree (int i) {
}
return (Skill Tree) super.rootSubtree(i);
no usages
public static void main(String[] args) {
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc2b1624f-0c38-4be2-8827-46d361bea958%2F381a7c7e-9e57-4c38-9567-f4f4f3d84b7e%2F7tkoto_processed.png&w=3840&q=75)


Step by step
Solved in 3 steps

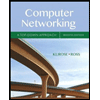
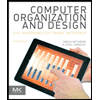
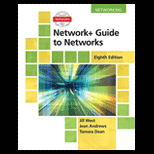
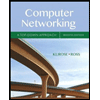
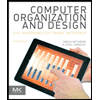
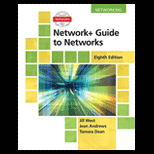
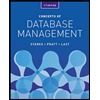
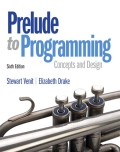
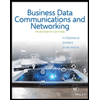