You will create a class that could be used to create a Text Adventure game. The class will be called "Player" that will represent a user-controlled character in the game. The Player Class The Player class will have the following 5 properties: A property to represent the player's name A property to represent the player's current x location. A property to represent the player's current y location. An array property that represents the list of items that the player is currently carrying. The length of the array should be 3, so the player will only be able to hold a maximum of 3 items at a time. A property that represents the number of items that the player is currently carrying. (This value should never be greater than 3.) (Note: Your Player class MUST have all of the properties listed above. You are allowed to create additional properties if needed, but please be judicious about it. In other words, you should only create additional properties if you have a legitimate reason for doing so.) The Player class will have exactly 5 methods: There will be a constructor that takes the player's name as a parameter. The player's initial location should be (0,0). The player should initially not be carrying any items. There will be a method called "move" that will take a Direction value as a parameter. You will create an enumeration called "Direction" with the values NORTH, SOUTH, EAST, and WEST. The "move" method will take one of these enumerated values as a parameter. If the player moves NORTH, its y location will be incremented. If it moves SOUTH, its y location will be decremented. If it moves EAST, its x location will be incremented. If it moves WEST, its x location will be decremented. There will be a method called "pickUpItem" that takes an Item as a parameter. The specified item should be added to the Player's array of items. (Note that the player will only be able to hold a maximum of 3 items, because of the capacity of the array. If a player tries to pick up an item while the array is full, then the item should NOT be picked up and an error message should be printed.) There will be a method called "putDownItem" that takes no parameters. The last item that was picked up by the player should be removed from the player's list of items. There will be a method called "print" that will print the Player's information to standard output. First, the name should be printed. Then, the player's current (x,y) location should be printed. Finally, the player's list of items should be printed.
You will create a class that could be used to create a Text Adventure game.
The class will be called "Player" that will represent a user-controlled character in the game.
The Player Class
The Player class will have the following 5 properties:
- A property to represent the player's name
- A property to represent the player's current x location.
- A property to represent the player's current y location.
- An array property that represents the list of items that the player is currently carrying. The length of the array should be 3, so the player will only be able to hold a maximum of 3 items at a time.
- A property that represents the number of items that the player is currently carrying. (This value should never be greater than 3.)
(Note: Your Player class MUST have all of the properties listed above. You are allowed to create additional properties if needed, but please be judicious about it. In other words, you should only create additional properties if you have a legitimate reason for doing so.)
The Player class will have exactly 5 methods:
- There will be a constructor that takes the player's name as a parameter. The player's initial location should be (0,0). The player should initially not be carrying any items.
- There will be a method called "move" that will take a Direction value as a parameter. You will create an enumeration called "Direction" with the values NORTH, SOUTH, EAST, and WEST. The "move" method will take one of these enumerated values as a parameter. If the player moves NORTH, its y location will be incremented. If it moves SOUTH, its y location will be decremented. If it moves EAST, its x location will be incremented. If it moves WEST, its x location will be decremented.
- There will be a method called "pickUpItem" that takes an Item as a parameter. The specified item should be added to the Player's array of items. (Note that the player will only be able to hold a maximum of 3 items, because of the capacity of the array. If a player tries to pick up an item while the array is full, then the item should NOT be picked up and an error message should be printed.)
- There will be a method called "putDownItem" that takes no parameters. The last item that was picked up by the player should be removed from the player's list of items.
- There will be a method called "print" that will print the Player's information to standard output. First, the name should be printed. Then, the player's current (x,y) location should be printed. Finally, the player's list of items should be printed.
PLEASE MAKE SURE ITS POSSIBLE TO COMPILE WITH THIS CODE. THANKS.
#include "Player.h"
#include <iostream>
#include <string> using namespace std;
int main()
{
Item sword("Sword of Destiny");
Item potion("Healing Potion");
Item key("Key of Wisdom");
string name;
cout << "Enter your name: ";
getline(cin, name);
Player user(name);
user.print();
cout << "USER PICKS UP SWORD:" << endl;
user.pickUpItem(sword);
user.print();
cout << "USER MOVES NORTH:" << endl;
user.move(NORTH);
user.print();
cout << "USER PICKS UP POTION:" << endl;
user.pickUpItem(potion);
user.print();
cout << "USER PUTS DOWN ITEM:" << endl;
user.putDownItem();
user.print();
cout << "USER MOVES WEST:" << endl;
user.move(WEST);
user.print();
cout << "USER PICKS UP KEY:" << endl;
user.pickUpItem(key);
user.print();
}

Step by step
Solved in 5 steps with 2 images

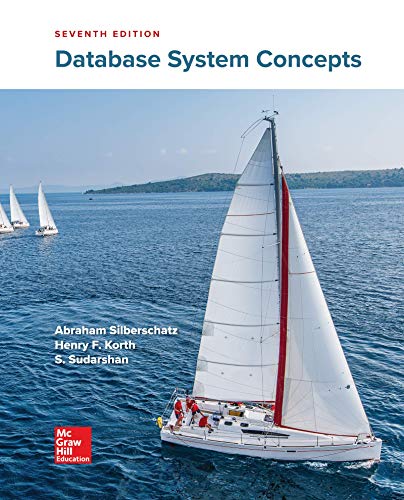
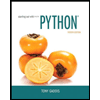
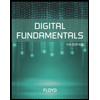
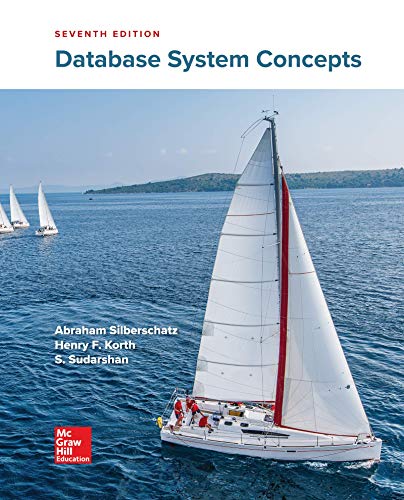
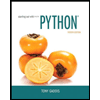
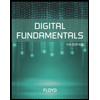
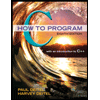
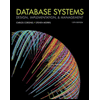
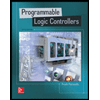