You should turn in FibonacciComparison.java and your plot (as a PNG). This can be a screenshot of the plot or the plot saved as a PNG file. Remember, your FibonacciComparison.java file should not be within a package other than src. This means the word "package" should not be at the top of the file signifying that the file is within a sub-directory of src. Your directory structure of your project should be: [project name] | src | | FibonacciComparison.java | | Pair.java There will be -15 points if one of the following happen: 1. Your code throws a compiler exception (meaning your code cannot be run) 2. You do not use FibonacciComparisonthe class name 3. Your FibonacciComparison.java file is within another package
You should turn in FibonacciComparison.java and your plot (as a PNG). This can be a screenshot of the plot or the plot saved as a PNG file.
Remember, your FibonacciComparison.java file should not be within a package other than src. This means the word "package" should not be at the top of the file signifying that the file is within a sub-directory of src. Your directory structure of your project should be:
[project name]
| src
| | FibonacciComparison.java
| | Pair.java
There will be -15 points if one of the following happen:
1. Your code throws a compiler exception (meaning your code cannot be run)
2. You do not use FibonacciComparisonthe class name
3. Your FibonacciComparison.java file is within another package
![import java.lang.System;
3.
public class FibonacciComparison {
4.
// Fibonacci Sequence: 0, 1, 1, 2, 3, 5, 8 ....
/*
7
input cases
8.
1) 0
9
2) 3
10
3) -1
11
4) 9
12
output cases
13
1) 0
14
2) 2
15
3) 0
16
4) 34
17
*/
// Note that you need to return 0 if the input is negative.
// Please pay close attention to the fact that the first index in our fib sequence is 0.
18
19
20
// Recursive Fibonacci
public static int fib(int n) {
// Code this func.
21
22
23
24
return -1;
25
26
// Iterative Fibonacci
27
28
public static int fiblinear(int n) {
// Code this func.
29
30
return -1;
31
32
33
public static void main(String[] args) {
34
// list of fibonacci sequence numbers
int[] nlist
w
{ 5,10, 15, 20, 25, 30, 35, 40, 45};
35
36
37
// Two arrays (one for fibLinear, other for fibRecursive) to store time for each run.
// There are a total of nlist.length inputs that we will test
double[] timingsEF = new double[nlist.Length];
double[] timingsLF = new double[nlist.length];
38
39
40
41
42
// Every number in n_list will be given as input 5 times to both fibonacci functions
43
// and an average will be taken to make the results more accurate.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc7f33394-5a52-4a6c-8272-5682354fd51c%2F5708b8a6-f4cf-4178-92ef-974599d5aba3%2Fyiwdcdf_processed.png&w=3840&q=75)
![44
int numTrials = 5;
45
46
//Iterating over number list
for ( int i = 0; i < nlist.length; i++ ) {
47
48
int n =
nlist[i];
49
50
//
FibRecursive
51
// Start recording time
52
<Code here>
53
// Run fibRecursive function 5 times
for ( int k = 0; k < numTrials; k++ )
54
55
fib(n);
// Stop recording time
56
57
<Code here>
wwww
58
// Taking average of the run time and store it in the array
59
timingsEF[i] = le-9*(stop-start) / numTrials;
60
61
//
Fiblinear
62
// Start recording time
63
<Code here>
ww
// Run Fiblinear 5 times
64
65
for ( int k = 0; k < numTrials; k++ )
fiblinear (n);
66
67
68
// Stop recording time
69
<Code here>
70
//Taking average and store it in the array
71
timingsLF[i] = le-9*(stop-start) / numTrials;
72
}
73
74
// Print out the runtimes for different fib functions.
75
System.out.println("Timings for Exponential Fibonacci");
for ( double time : timingsEF )
76
77
System.out.print(" "+time);
78
System.out.println();
79
System.out.println();
80
System.out.println();
81
System.out.println("Timings for Linear Fibonacci");
for ( double time : timingsLF )
82
83
System.out.print(" "+time);
84
System.out.println();
85
86](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc7f33394-5a52-4a6c-8272-5682354fd51c%2F5708b8a6-f4cf-4178-92ef-974599d5aba3%2F1tsod5y_processed.png&w=3840&q=75)

ANSWER:-
Step by step
Solved in 2 steps with 1 images

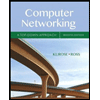
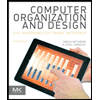
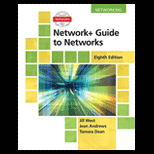
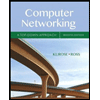
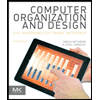
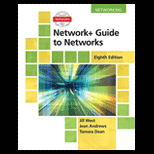
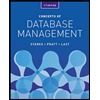
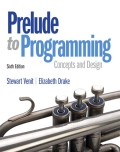
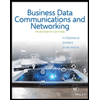