You are tasked with writing a function void removeOdds(). This function will remove all nodes that contain an odd value (any value not evenly divisible by 2). Make sure that you aren't leaking memory or leaving any dangling pointers! Example 1 list : 71 2 4 3 0 8 -3 list.removeOdds(); list : 2 4 0 8 Example 2 list : 581 -3 43 11 4 59 1 -81 -3 list.removeOdds() list : 4
You are tasked with writing a function void removeOdds(). This function will remove all nodes that contain an odd value (any value not evenly divisible by 2). Make sure that you aren't leaking memory or leaving any dangling pointers!
Example 1
list : 71 2 4 3 0 8 -3
list.removeOdds();
list : 2 4 0 8
Example 2
list : 581 -3 43 11 4 59 1 -81 -3
list.removeOdds()
list : 4
Main.CPP:
#include <iostream>
using namespace std;
#include "IntList.h"
int main() {
int testNum;
cout << "Enter test number: ";
cin >> testNum;
cout << endl;
// Test removeOdds function when first, middle, and last values odd
if (testNum == 1) {
IntList test1;
test1.push_front(-3);
test1.push_front(8);
test1.push_front(0);
test1.push_front(3);
test1.push_front(4);
test1.push_front(2);
test1.push_front(71);
cout << "Before: " << test1 << endl;
test1.removeOdds();
cout << "After : " << test1 << endl;
}
// Test removeOdds function when most values odd
if (testNum == 2) {
IntList test2;
test2.push_front(-3);
test2.push_front(-81);
test2.push_front(1);
test2.push_front(59);
test2.push_front(4);
test2.push_front(11);
test2.push_front(43);
test2.push_front(-3);
test2.push_front(581);
cout << "Before: " << test2 << endl;
test2.removeOdds();
cout << "After : " << test2 << endl;
}
return 0;
}
IntList.h:
#ifndef __INTLIST_H__
#define __INTLIST_H__
#include <ostream>
using namespace std;
struct IntNode {
int value;
IntNode *next;
IntNode(int val) : value(val), next(nullptr) {}
};
class IntList {
private:
IntNode *head;
public:
/* Initializes an empty list.
*/
IntList() : head(nullptr) {}
/* Inserts a data value to the front of the list.
*/
void push_front(int val) {
if (!head) {
head = new IntNode(val);
} else {
IntNode *temp = new IntNode(val);
temp->next = head;
head = temp;
}
}
/* Outputs to a single line all of the int values stored in the list, each separated by a space.
This function does NOT output a newline or space at the end.
*/
friend ostream & operator<<(ostream &out, const IntList &rhs) {
if (rhs.head) {
IntNode *curr = rhs.head;
out << curr->value;
for (curr = curr->next ; curr ; curr = curr->next) {
out << ' ' << curr->value;
}
}
return out;
}
/* Removes all nodes with an odd value. There is no return, the side effect is to modify the list.
*/
void removeOdds();
};
#endif
I need help filling in the IntList.cpp !
IntList.cpp:
#include "IntList.h"
void IntList::removeOdds() {
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

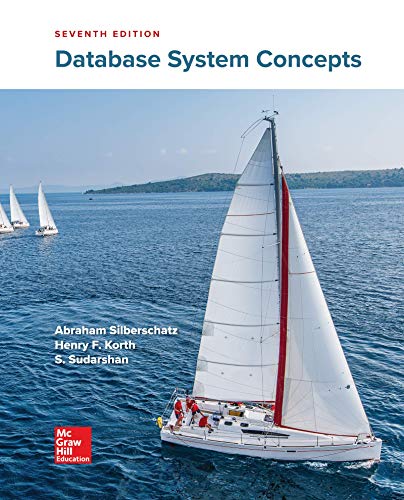
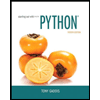
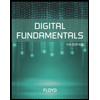
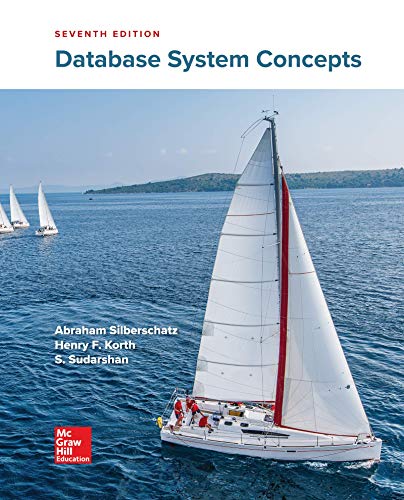
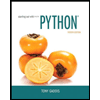
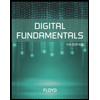
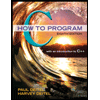
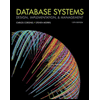
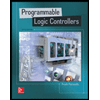