Write a struct Student that has member variables: (string) first name, (int) age and (double) fee. Write the functions as described in the class for the following purposes. 1. Write a c++ function to create a dynamic sorted (in ascending order according to the age)doubly linked list, where the data component of each node is an instance of the struct Student. 2. Write a function to insert the instances in the linked list. You also need to write a function to find the spot for insertion of the nodes. 3. Write a function to remove the node from the linked list. 4. Write a function to count the elements of the linked list. 5. Write a function to determine check whether an element belongs to the linked list. 6. Write a function to print the linked list (from head node) on the console. 7. Write a function to print the linked list (from tail node) on the console. Implement the above functions as follows. Initially, the list must have five nodes that are the instances of the struct whose member variable age are: 18, 21, 25, 27 and 33. Insert three nodes that are instances of the struct with age variable: (i) 17, (ii) 23 and (iii) 34. Print the list from head node (in ascending order). Remove three nodes that are instances of the struct with age variable 17, 25, and 34. Print the list from tail node (in descending order). Search the list to find whether an element that corresponds to the student with age 23. Insert a node that is an instance of struct with age variable 29. Print the number of elements in the list. Print the list from head (in ascending order) on the console
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Write a struct Student that has member variables: (string) first name, (int) age and (double) fee. Write the functions as described in the class for the following purposes.
1. Write a c++ function to create a dynamic sorted (in ascending order according to the age)doubly linked list, where the data component of each node is an instance of the struct
Student.
2. Write a function to insert the instances in the linked list. You also need to write a function to find the spot for insertion of the nodes.
3. Write a function to remove the node from the linked list.
4. Write a function to count the elements of the linked list.
5. Write a function to determine check whether an element belongs to the linked list.
6. Write a function to print the linked list (from head node) on the console.
7. Write a function to print the linked list (from tail node) on the console.
Implement the above functions as follows.
Initially, the list must have five nodes that are the instances of the struct whose member variable age are: 18, 21, 25, 27 and 33. Insert three nodes that are instances of the struct with age variable: (i) 17, (ii) 23 and (iii) 34. Print the list from head node (in ascending order). Remove three nodes
that are instances of the struct with age variable 17, 25, and 34. Print the list from tail node (in descending order). Search the list to find whether an element that corresponds to the student with age 23. Insert a node that is an instance of struct with age variable 29. Print the number of elements
in the list. Print the list from head (in ascending order) on the console

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

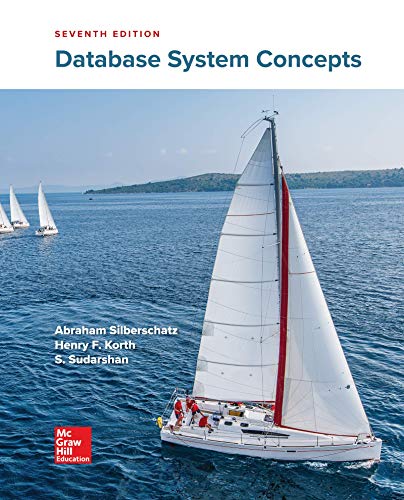
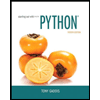
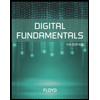
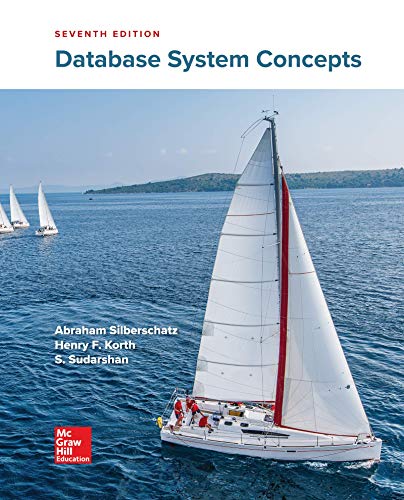
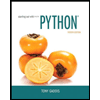
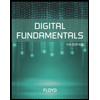
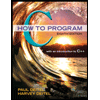
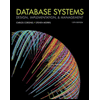
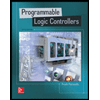