Write a recursive program that finds out ifn is a Fibonacci number or not. The Fibonacci numbers are 0 1 1 2 3 5 13 21 Your program should have the following interface: Enter a number (enter a negative number to quit): 14 !!!!! Sorry14 is not a Fibonacci number Enter a number (enter a negative number to quit): 13 Yes, you got it, 13 is a Fibonacci number Enter a number (enter a negative number to quit): 22 !!!!! Sorry 22 is not a Fibonacci number Enter a number (enter a negative number to quit): 23 !!!!! Sorry 23 is not a Fibonacci number Enter a number (enter a negative number to quit): 21 Yes, you got it, 21 is a Fibonacci number Enter a number (enter a negative number to quit): -1 (*Thanks Have a good Day*)
I have the following code:
#include <iostream>
#include <cstdlib>
using namespace std;
//main method
int main()
{
//variable declaration
int num, num1 = 0, num2 = 1, temp;
// getting input from user
cout<<"Enter a number (enter a negative number to quit): ";
cin>>num;
//If the number entered by the user is negative then exit the
if (num<0)
{
//exit statement
exit(0);
}
// 0 and 1 are fibonacci numbers
if (num1==num || num2==num)
{
cout << num <<" is a fibonacci number\n";
return 0;
}
// checking whether a given number is Fibonacci or not
while (num2 <= num)
{
//assigning the value of the second number to a temp variable
temp = num2;
//assigning the sum value of num1 and num2 to num2
num2 = num1 + num2;
//assigning temp varible value to num1
num1 = temp;
//if num2 is equal to the number entered by the user
if (num2 == num)
{
break;
}
}
// printing the results
if (num1==num||num2==num)
{
//displaying a message to the user that entered number is a Fibonacci number
cout<<"Yes, you got it, " <<num<< " is a Fibonacci number";
}
else
{
//displaying a message to the user that the entered number is not a Fibonacci number
cout<<"!!!!! Sorry "<<num <<" is not a Fibonacci number";
}
return 0;
}
but I need it to output like exact attached image, where I can keep inputing numbers until it quits and can pleases how the recursive formula is determing FIB nums


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

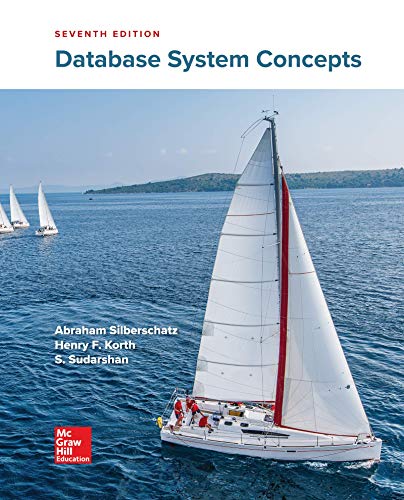
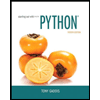
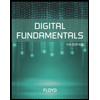
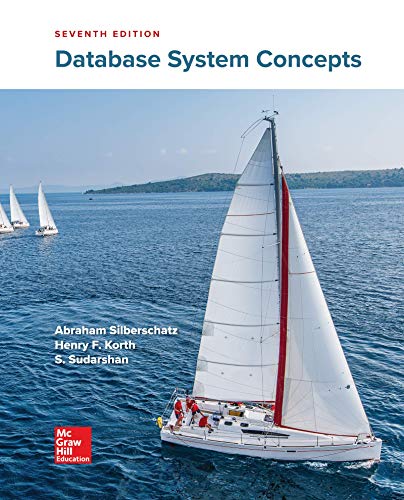
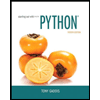
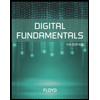
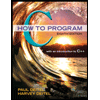
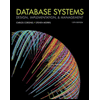
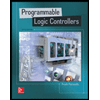