Write a RainFall class that stores the total rainfall for each of 12 months into an array of
import java.util.Scanner;
public class Rainfall {
double rain[];
public Rainfall() {
rain = new double[12];
}
public double averageRainfall() {
double number = getTotalRain() / 12.0;
double roundedNumber = Math.round(number * 10.0) / 10.0;
return roundedNumber;
}
public double getTotalRain() {
double total = 0.0;
for (int i = 0; i < 12; i++)
total += rain[i];
return total;
}
public int lowRainMonth() {
double min = rain[0];
int month = 0;
for (int i = 1; i < 12; i++) {
if (rain[i] < min) {
min = rain[i];
month = i;
}
}
return month;
}
public int highRainMonth() {
double max = rain[0];
int month = 0;
for (int i = 1; i < 12; i++) {
if (rain[i] > max) {
max = rain[i];
month = i;
}
}
return month;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Rainfall obj = new Rainfall();
for (int i = 0; i < 12; i++) {
System.out.println("Enter the rainfall amount for month " + (i + 1) + ":");
obj.rain[i] = sc.nextDouble();
}
System.out.println("Maximum rainfall: " + obj.rain[obj.highRainMonth()]);
System.out.println("Minimum rainfall: " + obj.rain[obj.lowRainMonth()]);
System.out.println("Total rainfall: " + obj.getTotalRain());
System.out.println("Average rainfall: " + obj.averageRainfall());
}
}
Test Case 1
1.2ENTER
Enter the rainfall amount for month 2:\n
2.3ENTER
Enter the rainfall amount for month 3:\n
3.4ENTER
Enter the rainfall amount for month 4:\n
5.1ENTER
Enter the rainfall amount for month 5:\n
1.7ENTER
Enter the rainfall amount for month 6:\n
6.5ENTER
Enter the rainfall amount for month 7:\n
2.5ENTER
Enter the rainfall amount for month 8:\n
3.3ENTER
Enter the rainfall amount for month 9:\n
1.1ENTER
Enter the rainfall amount for month 10:\n
5.5ENTER
Enter the rainfall amount for month 11:\n
6.6ENTER
Enter the rainfall amount for month 12:\n
6.0ENTER
Maximum rainfall: 6.6\n
Minimum rainfall: 1.1\n
Total rainfall: 45.2\n
Average rainfall: 3.8\n


In the given question, you are asked to create a Java class called `Rainfall` that can store monthly rainfall data for a year and perform the following tasks:
1. Calculate the total rainfall for the year.
2. Calculate the average monthly rainfall.
3. Identify the month with the highest amount of rainfall.
4. Identify the month with the lowest amount of rainfall.
You are also required to demonstrate the functionality of this class in a complete Java program.
Step by step
Solved in 4 steps with 3 images

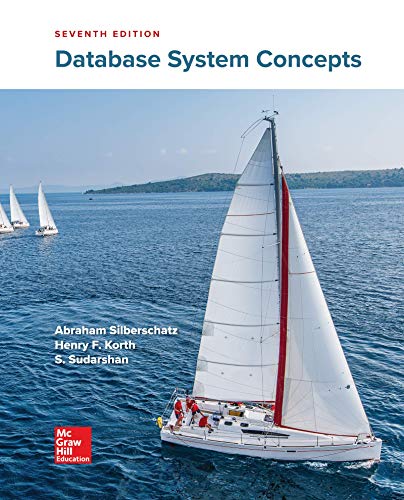
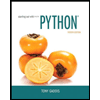
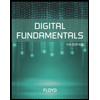
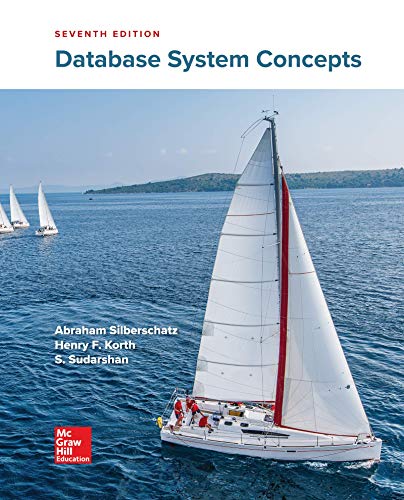
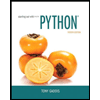
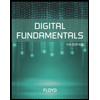
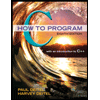
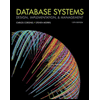
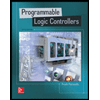