Write a Python program that takes two integers as input from the user and performs the following operations: a. Add the two integers together and store the result in a variable named sum. b. Subtract the second integer from the first integer and store the result in a variable named difference. c. Multiply the two integers together and store the result in a variable named product. d. Divide the first integer by the second integer and store the result in a variable named quotient. The output should be a floating point number. e. Calculate the remainder when the first integer is divided by the second integer and store the result in a variable named remainder. f. Print the values of sum, difference, product, quotient, and the remainder to the shell/console. Provide a label before each value to indicate what it represents (i.e. Sum: , Difference: , etc.). Each label/value pair must be on its own line. g. When your program is executed, the user should be able to enter their two integers, one at a time, and the result must match the screenshot below. However, the program should still work as expected regardless of what integers the user enters for input. Here is a screenshot of the expected behavior, testing all scenarios described above. Replicate this functionality in your script, including the formatting and outputs of all messages.
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
1. Write a Python
a. Add the two integers together and store the result in a variable named sum.
b. Subtract the second integer from the first integer and store the result in a variable named difference.
c. Multiply the two integers together and store the result in a variable named product.
d. Divide the first integer by the second integer and store the result in a variable named quotient. The output should be a floating point number.
e. Calculate the remainder when the first integer is divided by the second integer and store the result in a variable named remainder.
f. Print the values of sum, difference, product, quotient, and the remainder to the shell/console. Provide a label before each value to indicate what it represents (i.e. Sum: , Difference: , etc.). Each label/value pair must be on its own line.
g. When your program is executed, the user should be able to enter their two integers, one at a time, and the result must match the screenshot below. However, the program should still work as expected regardless of what integers the user enters for input. Here is a screenshot of the expected behavior, testing all scenarios described above. Replicate this functionality in your script, including the formatting and outputs of all messages.
.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6bd00569-71d6-4ece-b9f7-1b14421785b9%2Fbadfbcd6-6646-4fdd-a980-dc97aeab29d5%2Ftdb7xmi_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

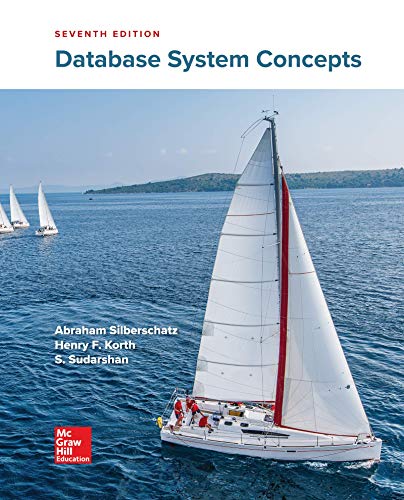
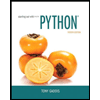
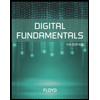
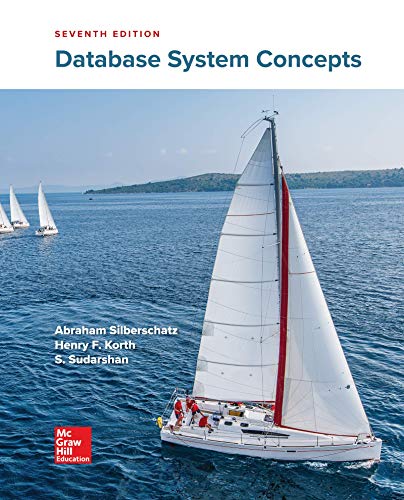
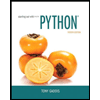
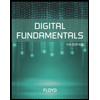
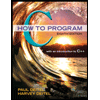
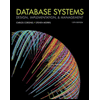
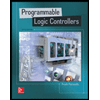