Write a program to allow a user to play the game, Hangman. DO NOT USE AN ARRAY The program will generate a random number (between 1 and 4581) to pick a word from the file - this is the word you then have to guess. Note: if the random number you generate is 42, you need the 42nd word - so loop 41 times picking up the word from the file and not doing anything with it, it is the 42nd you need. Here is how you will do this: String word: for (int k=0; k<41; k++) {word=scnr.nextLine(); }//end loop and now pick up the word you want word=scnr.nextLine() //this is the one you want The user will be allowed to guess a letter as many times as it takes - but 10 wrong guesses and they lose!! Eventually either they won or lost. In case they lost print out the answer. Java
Write a program to allow a user to play the game, Hangman. DO NOT USE AN ARRAY
The program will generate a random number (between 1 and 4581) to pick a word from the file - this is the word you then have to guess.
Note: if the random number you generate is 42, you need the 42nd word - so loop 41 times picking up the word from the file and not doing anything with it, it is the 42nd you need. Here is how you will do this:
String word:
for (int k=0; k<41; k++)
{word=scnr.nextLine();
}//end loop and now pick up the word you want
word=scnr.nextLine() //this is the one you want
The user will be allowed to guess a letter as many times as it takes - but 10 wrong guesses and they lose!!
Eventually either they won or lost. In case they lost print out the answer.
Java

Step by step
Solved in 2 steps with 5 images

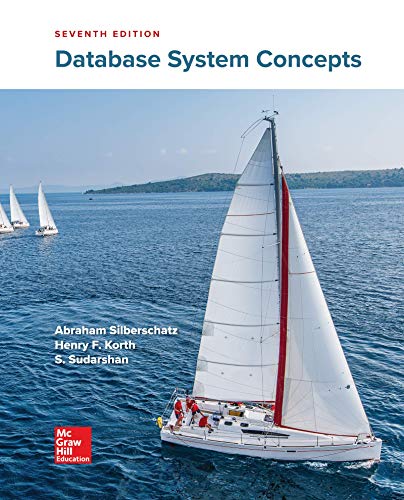
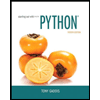
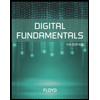
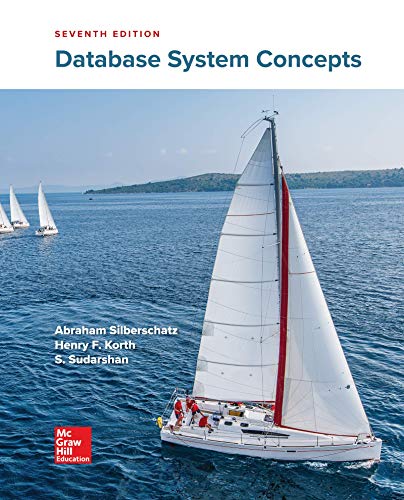
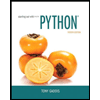
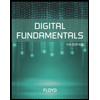
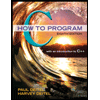
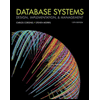
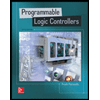