Java Write code statements to create an array based on user values and determine if that array contains at least one unique value. Code Specifications All of your code can go in the main method. Ask the user how many numbers they want to enter. Create an int[] using this size. Use a loop to read in the user's values and store them in the array. Determine if there is at least one unique value in the array. Hint: use a nested loop! Output the result to the user. What I have so far: import java.util.Scanner; public class UniqueNumbers { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("How many numbers do you want to enter?"); int numbers = Integer.parseInt(scan.nextLine()); int[]values = new int[numbers]; for(int i = 0; i
Java
Write code statements to create an array based on user values and determine if that array contains at least one unique value.
Code Specifications
All of your code can go in the main method.
- Ask the user how many numbers they want to enter.
- Create an int[] using this size.
- Use a loop to read in the user's values and store them in the array.
- Determine if there is at least one unique value in the array.
- Hint: use a nested loop!
- Output the result to the user.
What I have so far:
import java.util.Scanner;
public class UniqueNumbers {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.println("How many numbers do you want to enter?");
int numbers = Integer.parseInt(scan.nextLine());
int[]values = new int[numbers];
for(int i = 0; i<values.length; i++) {
System.out.println("Enter a number:");
values[i] = Integer.parseInt(scan.nextLine());
}
}
}
The picture is a table of test cases. I just need you to add the code that determines if there is a unique value
![Number
of
Numbers
5
5
4
6
3
4
4
5
Values in
the Array
{1, 2, 3, 4,
5}
{1, 2, 2, 1,
3}
(3, 5, 1, 1)
{6, 8, 2, 8,
6,5}
{4, 4, 4]
{7,7,7,7}
{1, 2, 1, 2}
{4, 4, 3, 4,
3}
Contains a
Unique
Number?
yes
yes
yes
yes
no
no
no
no](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7024c4db-e4c4-44c2-8fab-3045baf15ef1%2F197303ac-4cd1-4310-bcd3-fc78466bff69%2Fsnm2l_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

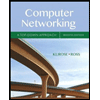
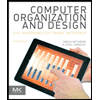
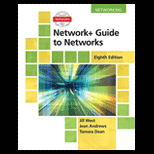
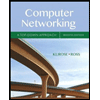
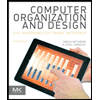
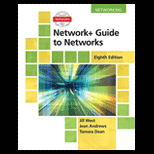
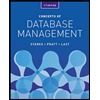
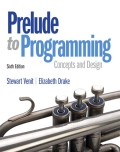
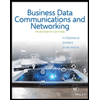