Write a program that generates a random number in the range of 1 through 1000, and asks the user to guess what the number is. If the user guess is higher than the random number, the program should display Too high, try again. If the users guess is lower than the random number, the program should display Too low, try again. If the user guesses the number, the application should congratulate the user and generate a new random number so the game can start over. If the user doesn't want to continue the game the user should type an appropriate stop symbol to terminate the program (such stop conditions were discussed in the class). For each game iteration, count the number of guesses made by the user and display them. The program should contain the following functions: check_guess(): this function checks if the user's guess is lower, higher or equal to the random number. random_gen(): function that generates one random number for one game iteration main(): the function where the program execution should begin and end. The number of function arguments and parameters are left for your own design choice.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Write a program that generates a random number in the range of 1 through 1000, and asks
the user to guess what the number is. If the user guess is higher than the random number,
the program should display Too high, try again. If the users guess is lower than the
random number, the program should display Too low, try again. If the user guesses the
number, the application should congratulate the user and generate a new random number
so the game can start over. If the user doesn't want to continue the game the user should type an appropriate stop symbol to terminate the program (such stop conditions were discussed in the class). For each game iteration, count the number of guesses made by the user and display them. The program should contain the following functions:
check_guess(): this function checks if the user's guess is lower, higher or equal to the random number.
random_gen(): function that generates one random number for one game iteration
main(): the function where the program execution should begin and end.
The number of function arguments and parameters are left for your own design choice.

Step by step
Solved in 4 steps with 2 images

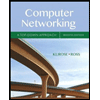
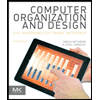
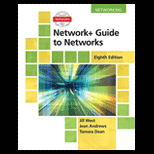
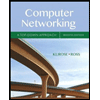
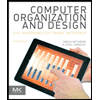
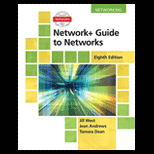
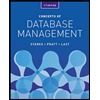
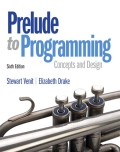
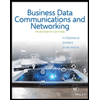