Write an application that prompts a user for two integers and displays every integer between them. Display There are no integers between X and Y if there are no integers between the entered values. Make sure the program works regardless of which entered value is larger. An example of the program is shown below: Enter an integer >> 12 Enter another integer >> 20 The numbers between 12 and 20 include: 13 14 15 16 17 18 19
Java:


here is a Java program that does what you described:
import java.util.Scanner;
public class IntegerBetween {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer >> ");
int num1 = input.nextInt();
System.out.print("Enter another integer >> ");
int num2 = input.nextInt();
int start = Math.min(num1, num2);
int end = Math.max(num1, num2);
System.out.print("The numbers between " + start + " and " + end + " include: ");
for (int i = start + 1; i < end; i++) {
System.out.print(i + " ");
}
if (start + 1 == end) {
System.out.print("There are no integers between " + start + " and " + end);
}
input.close();
}
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

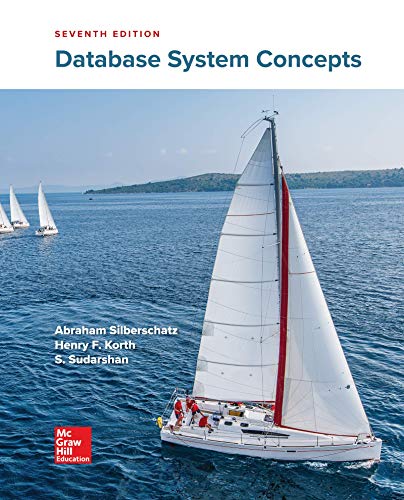
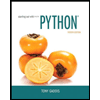
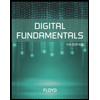
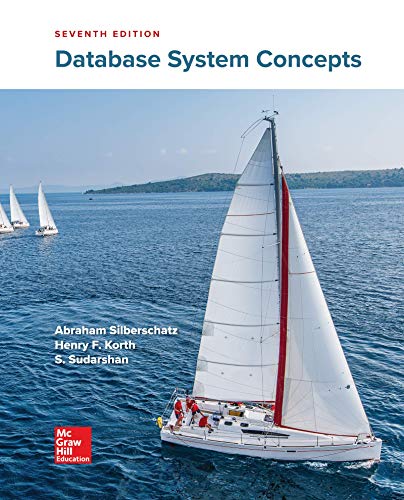
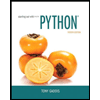
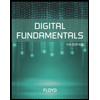
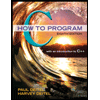
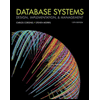
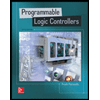