I am trying to create a lottery game application in Java. I want it to generate three random numbers between 0 and 9 (including 0 and 9). Then, the user will input their three guesses of what the winning numbers will be. Next, compare each of the user's guesses to the three random numbers and display a message that includes the user's guess, the randomly determined three digits, and the amount of money the user has won. I need to make certain that this application accommodates repeating digits. For example, if a user guesses 1, 2, and 3, and the randomly generated digits are 1, 1, and 1, do not give the user credit for three correct guesses-just one. (From this, it stands to reason that if the randomly generated digits include 2 repeated digits, that they also should be counted as-just one.) This is an example of the final output I am hoping to achieve with this program: Guess the 3 numbers I have chosen. Guess 1 right $10 Guess 2 right $100 Guess 3 right (any order) $1,000 Guess 3 right (exact order) $1,000,000 Enter the 1st number: 1 Enter the 2nd number: 4 Enter the 3rd number: 5 Computer picks: 1 2 3 You guessed: 1 4 5 1 Match, you win $10 I want to try and identify ALL test cases. A test case will include the input necessary to create an expected output. So far in my program, I have:
I am trying to create a lottery game application in Java. I want it to generate three random numbers between 0 and 9 (including 0 and 9). Then, the user will input their three guesses of what the winning numbers will be. Next, compare each of the user's guesses to the three random numbers and display a message that includes the user's guess, the randomly determined three digits, and the amount of money the user has won. I need to make certain that this application accommodates repeating digits. For example, if a user guesses 1, 2, and 3, and the randomly generated digits are 1, 1, and 1, do not give the user credit for three correct guesses-just one. (From this, it stands to reason that if the randomly generated digits include 2 repeated digits, that they also should be counted as-just one.)
This is an example of the final output I am hoping to achieve with this program:
Guess the 3 numbers I have chosen.
Guess 1 right $10
Guess 2 right $100
Guess 3 right (any order) $1,000
Guess 3 right (exact order) $1,000,000
Enter the 1st number: 1
Enter the 2nd number: 4
Enter the 3rd number: 5
Computer picks: 1 2 3 You guessed: 1 4 5
1 Match, you win $10
I want to try and identify ALL test cases. A test case will include the input necessary to create an expected output.
So far in my program, I have:
![D2L ZyBooks Chapter 3 PA/CA/Lab X
←
C
onlinegdb.com/#
SPONSOR Gitlab - Loved by developers. Trusted by enterprises. Start your free 30 day trial. X
Run
LotteryGameApplic... :
16
17
18
19
20
21
22
23
24
25
26
27
28
GDB online Debugger | Comp X D21 Homework Instructions - CSC × b Home | bartleby
Debug
Stop
Share
1
2
3
4- public class LotteryGameApplication{
5
6-
7
8
9
10
11
12
13
14
15
H Save
public static void main(String[] args) {
//User enters three guesses
System.out.println("Guess the three numbers I have chosen
System.out.println("Guess 1 right
System.out.println("Guess 2 right
System.out.println("Guess 3 right (any order)
System.out.println("Guess
3 right (exact order)
System.out.println("Enter the 1st number: ");
System.out.println("Enter the 2nd number: ");
System.out.println("Enter the 3rd number: ");
//Winnings
final double ZeroDollars
final double TenDollars
final double Hundred Dollars
1000;
final double Thousand Dollars
final double AMillion Dollars = 1000000;
input
{} Beautify
-
0;
10;
-
=
100;
//Reveals Winning Lottery Numbers
int min 0:
x +
between 0 and 9. ");
$10");
$100");
$1000");
$1,000,000");
stderr
Language Java
Ⓒ
GI
Ⓡ
PH
€0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc60c9d1f-b3f6-4480-9546-9a7e3a18aff9%2F20d8de06-e17c-43e7-89de-863a0b2c766c%2F592bon5_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

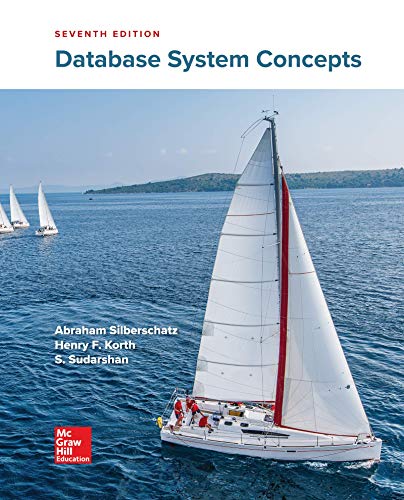
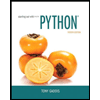
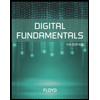
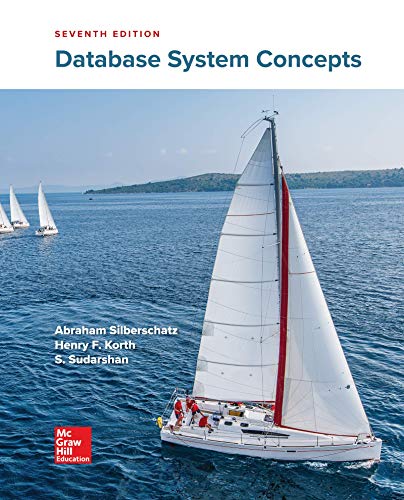
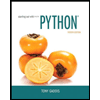
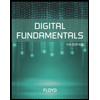
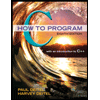
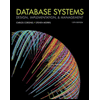
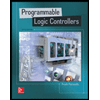