Write a program, sonar.c, compiled with GCC on the RPi 3b/3b+, that performs the following steps using the provided sysfs_gpio files: a. Include the sysfs_gpio.h file b. Initializes the TRIGGER pin to be an output by calling gpioOutput() c. Initializes the ECHO pin to be an input by calling gpioInput() d. Sets Trig signal high (1) and then low (0) e. Zero a count variable f. Enter a while loop that waits until the Echo signal goes high There is no code in the while loop g. The program exits the first loop h. Enter another while loop that waits until the Echo signal goes low The while loop contains code that increments the count variable i. The program exits the second loop j. The count value is now proportional to the distance traveled k. Print the raw count value l. Convert the value to a distance (cm) by empirical means (the desk is about 4 feet or 122 cm in length) sysfs_gpio.c: #include //----------------------------------------------------------------------------- // Subroutines //----------------------------------------------------------------------------- void gpioOutput(int pin) { FILE* file; char str[35]; file = fopen("/sys/class/gpio/export", "w"); fprintf(file, "%d", pin); fclose(file); sprintf(str, "/sys/class/gpio/gpio%d/direction", pin); file = fopen(str, "w"); fprintf(file, "out"); fclose(file); } void gpioInput(int pin) { FILE* file; char str[35]; file = fopen("/sys/class/gpio/export", "w"); fprintf(file, "%d", pin); fclose(file); sprintf(str, "/sys/class/gpio/gpio%d/direction", pin); file = fopen(str, "w"); fprintf(file, "in"); fclose(file); } void gpioWrite(int pin, int value) { FILE* file; char str[35]; sprintf(str, "/sys/class/gpio/gpio%d/value", pin); file = fopen(str, "w"); fprintf(file, "%d", value); fclose(file); } int gpioRead(int pin) { FILE* file; int result; char str[30]; sprintf(str, "/sys/class/gpio/gpio%d/value", pin); file = fopen(str, "rw"); fscanf(file, "%d", &result); fclose(file); return result; } sysfs_gpio.h : #ifndef SYSFS_GPIO_H_ #define SYSFS_GPIO_H_ // Called to configure the pin as an input or output void gpioOutput(int pin); void gpioInput(int pin); // Called to set and output pin to a given value (1 or 0) void gpioWrite(int pin, int value); // Called to get the status (1 or 0) of a pin int gpioRead(int pin); #endif
Write a
using the provided sysfs_gpio files:
a. Include the sysfs_gpio.h file
b. Initializes the TRIGGER pin to be an output by calling gpioOutput()
c. Initializes the ECHO pin to be an input by calling gpioInput()
d. Sets Trig signal high (1) and then low (0)
e. Zero a count variable
f. Enter a while loop that waits until the Echo signal goes high
There is no code in the while loop
g. The program exits the first loop
h. Enter another while loop that waits until the Echo signal goes low
The while loop contains code that increments the count variable
i. The program exits the second loop
j. The count value is now proportional to the distance traveled
k. Print the raw count value
l. Convert the value to a distance (cm) by empirical means
(the desk is about 4 feet or 122 cm in length)
sysfs_gpio.c:
//-----------------------------------------------------------------------------
// Subroutines
//-----------------------------------------------------------------------------
void gpioOutput(int pin)
{
FILE* file;
char str[35];
file = fopen("/sys/class/gpio/export", "w");
fprintf(file, "%d", pin);
fclose(file);
sprintf(str, "/sys/class/gpio/gpio%d/direction", pin);
file = fopen(str, "w");
fprintf(file, "out");
fclose(file);
}
void gpioInput(int pin)
{
FILE* file;
char str[35];
file = fopen("/sys/class/gpio/export", "w");
fprintf(file, "%d", pin);
fclose(file);
sprintf(str, "/sys/class/gpio/gpio%d/direction", pin);
file = fopen(str, "w");
fprintf(file, "in");
fclose(file);
}
void gpioWrite(int pin, int value)
{
char str[35];
sprintf(str, "/sys/class/gpio/gpio%d/value", pin);
file = fopen(str, "w");
fprintf(file, "%d", value);
fclose(file);
}
int gpioRead(int pin)
{
FILE* file;
int result;
char str[30];
sprintf(str, "/sys/class/gpio/gpio%d/value", pin);
file = fopen(str, "rw");
fscanf(file, "%d", &result);
fclose(file);
return result;
}
#ifndef SYSFS_GPIO_H_
#define SYSFS_GPIO_H_
// Called to configure the pin as an input or output
void gpioOutput(int pin);
void gpioInput(int pin);
// Called to set and output pin to a given value (1 or 0)
void gpioWrite(int pin, int value);
// Called to get the status (1 or 0) of a pin
int gpioRead(int pin);
#endif

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

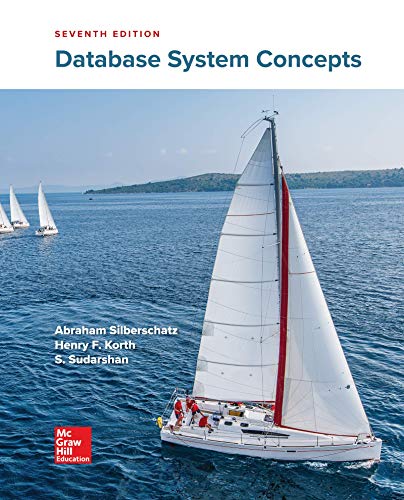
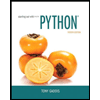
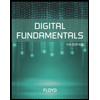
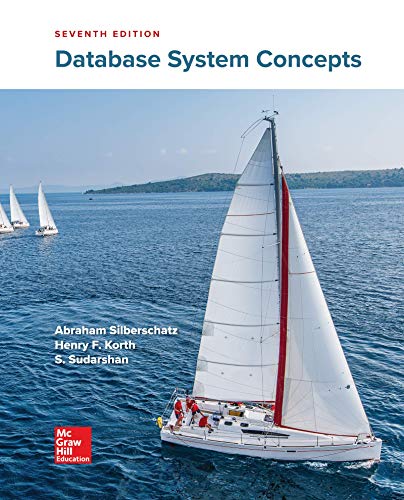
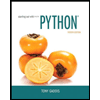
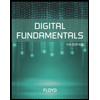
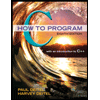
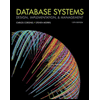
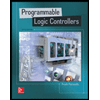