Write a loop that iterates over a String s and counts, then prints the total number of x's plus y's, plus z's combined. (i.e. if s = "xyzdddfgx" your code should print 4)
Write a loop that iterates over a String s and counts, then prints the total number of x's plus y's, plus z's combined. (i.e. if s = "xyzdddfgx" your code should print 4)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Attached is a question about a loop in my lab. We are using java and I am lost.
![### Loop to Count Specific Characters in a String
**Problem Statement:**
Write a loop that iterates over a `String` `s` and counts, then prints the total number of x’s plus y’s, plus z’s combined. *(i.e. if `s` = `"xyzdddfgx"` your code should print 4)*
**Detailed Explanation:**
1. **Objective:** The task is to write a loop in a programming language (such as Python, Java, etc.) that will go through each character in a string and keep a count of how many times the characters 'x', 'y', and 'z' appear.
2. **Example:**
- If the input string is `"xyzdddfgx"`, the output should be `4`, because the characters 'x', 'y', and 'z' appear a total of 4 times in the string.
#### Steps to Solve the Problem:
1. **Initialize a Counter:**
- Start by initializing a counter to zero. This counter will keep track of the total number of specific characters ('x', 'y', 'z') encountered in the string.
2. **Iterate Over the String:**
- Use a loop to go through each character in the string `s`.
3. **Check for Specific Characters:**
- During each iteration, check if the current character is either 'x', 'y', or 'z'.
- If it is, increment the counter by one.
4. **Print the Result:**
- After the loop has finished, print the value of the counter, which is the total number of 'x's, 'y's, and 'z's in the string.
#### Example Code in Python:
```python
# Example String
s = "xyzdddfgx"
# Initialize the counter
count = 0
# Loop through each character in the string
for char in s:
# Check if the character is 'x', 'y', or 'z'
if char in ['x', 'y', 'z']:
# Increment the counter
count += 1
# Print the result
print(count)
```
### Visual Aid
There are no graphs or diagrams associated with this problem. The explanation mainly involves understanding the logic of iteration and conditional counting within a string.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0309cbb4-3056-403b-8eb0-8f64136b6051%2F327ae3ae-64e2-4747-ab06-61da14fed2a5%2Fm504ggj_processed.png&w=3840&q=75)
Transcribed Image Text:### Loop to Count Specific Characters in a String
**Problem Statement:**
Write a loop that iterates over a `String` `s` and counts, then prints the total number of x’s plus y’s, plus z’s combined. *(i.e. if `s` = `"xyzdddfgx"` your code should print 4)*
**Detailed Explanation:**
1. **Objective:** The task is to write a loop in a programming language (such as Python, Java, etc.) that will go through each character in a string and keep a count of how many times the characters 'x', 'y', and 'z' appear.
2. **Example:**
- If the input string is `"xyzdddfgx"`, the output should be `4`, because the characters 'x', 'y', and 'z' appear a total of 4 times in the string.
#### Steps to Solve the Problem:
1. **Initialize a Counter:**
- Start by initializing a counter to zero. This counter will keep track of the total number of specific characters ('x', 'y', 'z') encountered in the string.
2. **Iterate Over the String:**
- Use a loop to go through each character in the string `s`.
3. **Check for Specific Characters:**
- During each iteration, check if the current character is either 'x', 'y', or 'z'.
- If it is, increment the counter by one.
4. **Print the Result:**
- After the loop has finished, print the value of the counter, which is the total number of 'x's, 'y's, and 'z's in the string.
#### Example Code in Python:
```python
# Example String
s = "xyzdddfgx"
# Initialize the counter
count = 0
# Loop through each character in the string
for char in s:
# Check if the character is 'x', 'y', or 'z'
if char in ['x', 'y', 'z']:
# Increment the counter
count += 1
# Print the result
print(count)
```
### Visual Aid
There are no graphs or diagrams associated with this problem. The explanation mainly involves understanding the logic of iteration and conditional counting within a string.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
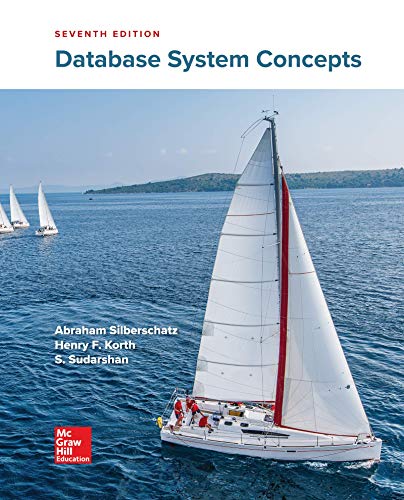
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
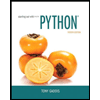
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
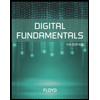
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
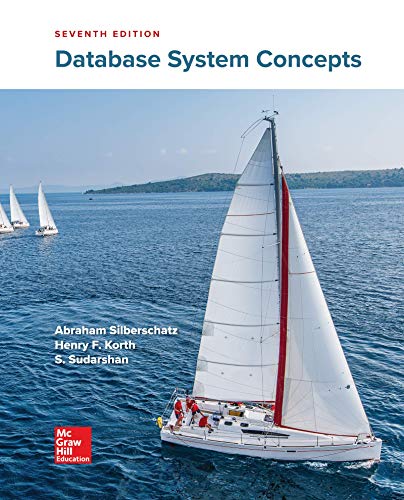
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
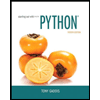
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
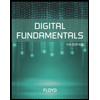
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
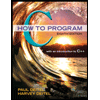
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
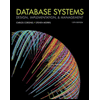
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
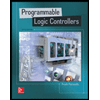
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education