t principles to perform the conversion. Print the base-10 number when the loop ends... He sent me a diagram of the binary scale and i've never seen this scale before so I'm having issues with my program. I CAN'T use array, which is an issue I'm having right now. public static int getDecimal(int binary) { int decimal = 0;
The instructions my prof. wants is this: Write a program that prompts the user for a binary number (from 3 to 8 bits) as a String and converts it to base-10 decimal value. There are several ways to do this in Java but your program must use a for loop and first principles to perform the conversion. Print the base-10 number when the loop ends... He sent me a diagram of the binary scale and i've never seen this scale before so I'm having issues with my program. I CAN'T use array, which is an issue I'm having right now.
public static int getDecimal(int binary) {
int decimal = 0;
int n = 0;
while(true) {
if(binary == 0) {
break;
}else {
int temp = binary %10;
decimal += temp * Math.pow(2, n);
binary = binary / 10;
n++;
}
}
return decimal;
}
public static void main(String[] args) {
System.out.println("11 binary to decimal: " + getDecimal(11)); //3
System.out.println("100 binary to decimal: " + getDecimal(100)); //4
System.out.println("0101 binary to decimal: " + getDecimal(0101)); //5
System.out.println("0110 binary to decimal: " + getDecimal(0110)); //6
System.out.println("0111 binary to decimal: " + getDecimal(0111)); //7
System.out.println("1000 binary to decimal: " + getDecimal(1000)); //8
}
}

Step by step
Solved in 3 steps with 2 images

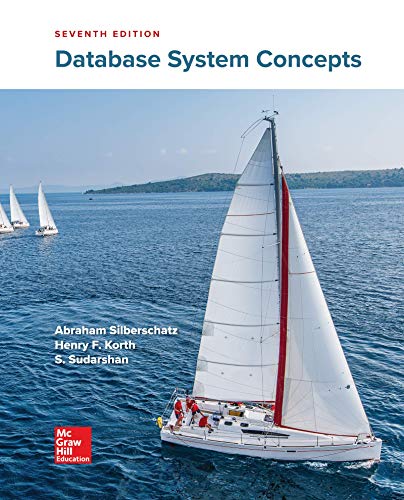
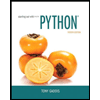
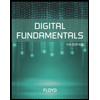
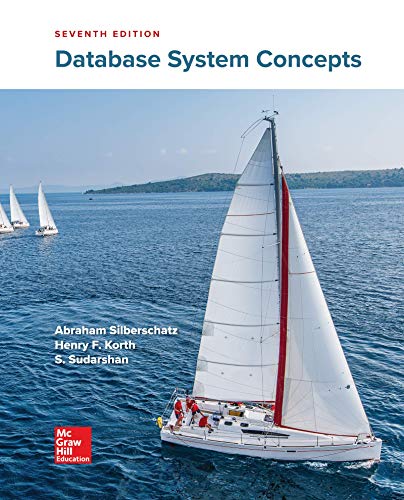
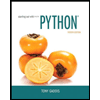
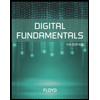
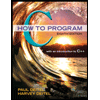
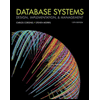
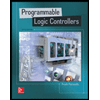