Write a function called tuneSimilarity that computes the similarity score as described above for two tunes of equal length. Function Specifications: • Name: tuneSimilarity() • Parameters (Your function should accept these parameters IN THIS ORDER): o tune1 (string): The first input tune o tune2 (string): The second input tune • Return Value: The similarity score (double) • The parameters should be two strings of equal length. If they are not equal in length, your function should return 0. • The function should not print anything. • You may assume that the input to tuneSimilarity will always be valid SPN, i.e. you do not have to account for arbitrary strings. --- Examples --- Sample function call tuneSimilarity ("G4E5D4", "G4F4D5") tuneSimilarity ("A0B0C0D0", "D1C1B1A1") tuneSimilarity ("E5E5G5A6G5D5", "E5G5A6G5D5D5") tuneSimilarity ("D5G2", "F7D1E4G4") Expected return value 0.666667 -4 0.333333 0 An example test case for the first sample function call would be: assert (doubles_equal(tuneSimilarity ("G4E5D4", "G4F4D5"), 0.666667)); Your file should be named tuneSimilarity.cpp and should also include a main function that tests your tuneSimilarity function. Once you have finished developing your solution in VSCode you should head over to the CodeRunner on Canvas and paste only your function tuneSimilarity into the answer box for question 4. You do not need to paste your main function into Coderunner. A main function has already been provided for you. You will need to include your main, tuneSimilarity and any other helper functions in the tuneSimilarity.cpp file that you submit to Canvas.
Use C++ to write this code
Write a function called tuneSimilarity that computes the similarity score as described above for two tunes of equal length.
Function Specifications:
- Name: tuneSimilarity()
- Parameters (Your function should accept these parameters IN THIS ORDER):
- tune1 (string): The first input tune
- tune2 (string): The second input tune
- Return Value: The similarity score (double)
- The parameters should be two strings of equal length. If they are not equal in length, your function should return 0.
- The function should not print anything.
- You may assume that the input to tuneSimilarity will always be valid SPN, i.e. you do not have to account for arbitrary strings.
--- Examples ---
Sample function call | Expected return value |
---|---|
tuneSimilarity("G4E5D4", "G4F4D5") | 0.666667 |
tuneSimilarity("A0B0C0D0", "D1C1B1A1") | -4 |
tuneSimilarity("E5E5G5A6G5D5", "E5G5A6G5D5D5") | 0.333333 |
tuneSimilarity("D5G2", "F7D1E4G4") | 0 |
An example test case for the first sample function call would be: assert(doubles_equal(tuneSimilarity("G4E5D4", "G4F4D5"), 0.666667));
Your file should be named tuneSimilarity.cpp and should also include a main function that tests your tuneSimilarity function. Once you have finished developing your solution in VSCode you should head over to the CodeRunner on Canvas and paste only your function tuneSimilarity into the answer box for question 4. You do not need to paste your main function into Coderunner. A main function has already been provided for you. You will need to include your main, tuneSimilarity and any other helper functions in the tuneSimilarity.cpp file that you submit to Canvas.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

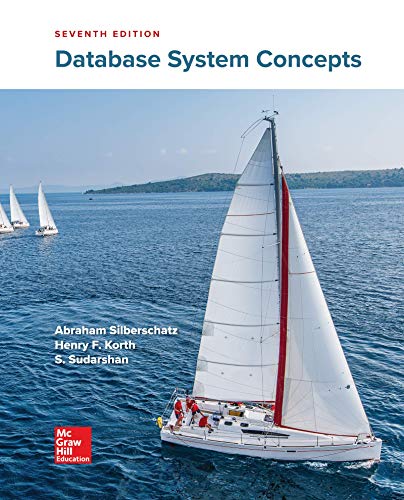
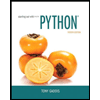
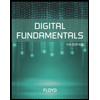
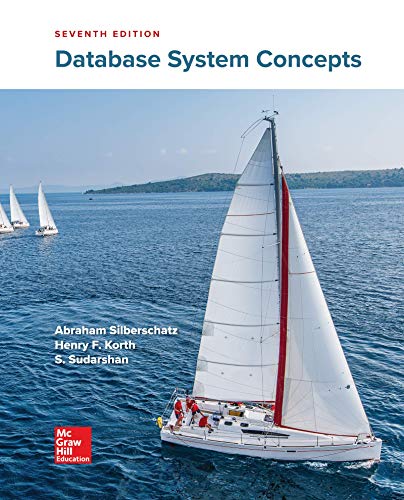
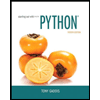
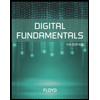
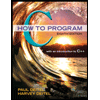
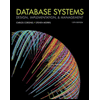
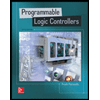