Functions and Prototypes - Converting Temperatures In this exercise you will create a program that will be used to convert Fahrenheit temperatures to Celsius and Kelvin temperatures through the use of two functions. For this program, assume that temperature will be represented as a double value. Begin by defining the function prototypes for the functions fahrenheit_to_celsius and fahrenheit_to_kelvin which are both passed a double value and return a double value. Now, at the bottom of the file, write the full definitions of both functions. The function fahrenheit_to_celsius is passed a Fahrenheit temperature and returns a rounded Celsius temperature. You may use the function round in order to round the return value. The formula to convert Fahrenheit to Celsius is (5.0/9.0) * (temperature - 32). The function fahrenheit_to_kelvin is passed a Fahrenheit temperature and returns a rounded Kelvin temperature. The formula to convert Fahrenheit to Kelvin is (5.0/9.0) * (temperature - 32) + 273. Remember the rules of PEMDAS when defining the body of your functions. Now, from the temperature_conversion function, declare and initialize the variables celsius_temperature and kelvin_temperature by calling the appropriate functions which you have just defined and passing the variable fahrenheit_temperature. You do not need to declare or initialize fahrenheit_temperature as it is already available to you as a parameter of the temperature_conversion function.
Functions and Prototypes - Converting Temperatures In this exercise you will create a program that will be used to convert Fahrenheit temperatures to Celsius and Kelvin temperatures through the use of two functions. For this program, assume that temperature will be represented as a double value. Begin by defining the function prototypes for the functions fahrenheit_to_celsius and fahrenheit_to_kelvin which are both passed a double value and return a double value. Now, at the bottom of the file, write the full definitions of both functions. The function fahrenheit_to_celsius is passed a Fahrenheit temperature and returns a rounded Celsius temperature. You may use the function round in order to round the return value. The formula to convert Fahrenheit to Celsius is (5.0/9.0) * (temperature - 32). The function fahrenheit_to_kelvin is passed a Fahrenheit temperature and returns a rounded Kelvin temperature. The formula to convert Fahrenheit to Kelvin is (5.0/9.0) * (temperature - 32) + 273. Remember the rules of PEMDAS when defining the body of your functions. Now, from the temperature_conversion function, declare and initialize the variables celsius_temperature and kelvin_temperature by calling the appropriate functions which you have just defined and passing the variable fahrenheit_temperature. You do not need to declare or initialize fahrenheit_temperature as it is already available to you as a parameter of the temperature_conversion function.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C++

Transcribed Image Text:### Transcription of C++ Template for Temperature Conversion Program
This is a code template for creating a simple C++ program that asks the user to enter a temperature in Fahrenheit. The temperature is then passed to a `temperature_conversion` function which computes its equivalent in Celsius and Kelvin using the `celsius_temperature` and `kelvin_temperature` functions.
```cpp
#include <iostream>
#include <cmath>
using namespace std;
//----DO NOT MODIFY THE CODE ABOVE THIS LINE----
//----WRITE YOUR FUNCTION PROTOTYPES BELOW THIS LINE----
//----WRITE YOUR FUNCTION PROTOTYPES ABOVE THIS LINE----
//----WRITE YOUR int main() BELOW THIS LINE----
//----WRITE YOUR int main() ABOVE THIS LINE----
//----DO NOT MODIFY THE CODE BELOW THIS LINE----
void temperature_conversion(double fahrenheit_temperature) {
//----DO NOT MODIFY THE CODE ABOVE THIS LINE----
//----WRITE YOUR FUNCTION CALLS BELOW THIS LINE----
//----WRITE YOUR FUNCTION CALLS ABOVE THIS LINE----
//----DO NOT MODIFY THE CODE BELOW THIS LINE----
cout << "The fahrenheit temperature " << fahrenheit_temperature
<< " degrees is equivalent to " << celsius_temperature
<< " degrees celsius and " << kelvin_temperature
<< " degrees kelvin.";
}
//----DO NOT MODIFY THE CODE ABOVE THIS LINE----
```
#### Explanation
- **Include Libraries**: The program starts by including the `<iostream>` and `<cmath>` libraries for input-output operations and mathematical functions respectively.
- **Namespace**: The `std` namespace is used to simplify code writing and eliminate the need for `std::` prefix.
- **Function Prototypes**: You need to define the prototypes for the `celsius_temperature` and `kelvin_temperature` functions between the designated comment lines.
- **Main Function**: The `main()` function, where user input is handled, should be inserted between the provided placeholders.
- **Temperature Conversion**: The `temperature_conversion()` function computes and displays the Celsius and Kelvin equivalents of the Fahrenheit input, utilizing the `celsius_temperature` and `kelvin_temperature` converter functions. Implement these functions and insert their calls where indicated.
Ensure to complete the program by developing the necessary functions and adding appropriate logic within the `main()` to prompt user input.

Transcribed Image Text:# Functions and Prototypes - Converting Temperatures
In this exercise, you will create a program that will be used to convert Fahrenheit temperatures to Celsius and Kelvin temperatures through the use of two functions.
For this program, assume that **temperature will be represented as a double value**.
### Step-by-Step Instructions:
1. **Define Function Prototypes:**
- Begin by defining the function prototypes for the functions `fahrenheit_to_celsius` and `fahrenheit_to_kelvin` which are both **passed a double value** and **return a double value**.
2. **Function Definitions:**
- At the bottom of the file, write the full definitions of both functions.
3. **fahrenheit_to_celsius:**
- This function is **passed a Fahrenheit temperature** and **returns a rounded Celsius temperature**. You may use the `<cmath>` function `round` to round the return value.
- Formula: \( \frac{5.0}{9.0} \times (\text{temperature} - 32) \).
4. **fahrenheit_to_kelvin:**
- This function is **passed a Fahrenheit temperature** and **returns a rounded Kelvin temperature**.
- Formula: \( \frac{5.0}{9.0} \times (\text{temperature} - 32) + 273 \).
5. **PEMDAS Rules:**
- Remember the rules of **PEMDAS** (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction) when defining the body of your functions.
6. **Declare and Initialize Variables:**
- From the `temperature_conversion` function, declare and initialize the variables `celsius_temperature` and `kelvin_temperature` by calling the appropriate functions you have just defined, and passing the variable `fahrenheit_temperature`.
7. **Usage of Parameters:**
- You do not need to declare or initialize `fahrenheit_temperature` as it is already available to you as a parameter of the `temperature_conversion` function.
This guide provides the necessary steps to create functions that convert temperatures from Fahrenheit to Celsius and Kelvin, emphasizing proper function definition and parameter handling.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
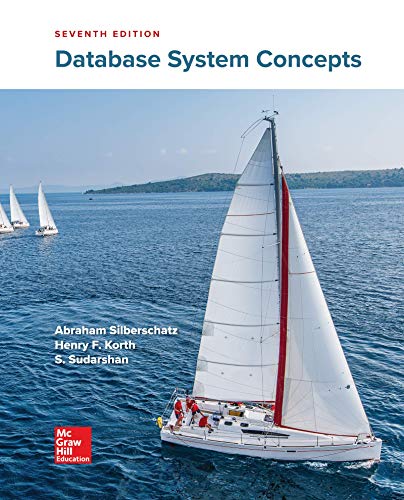
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
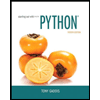
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
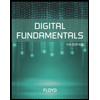
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
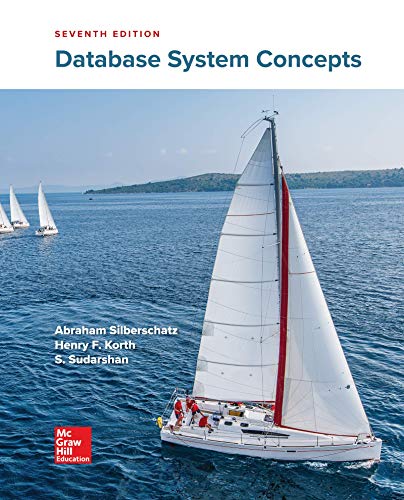
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
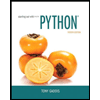
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
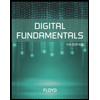
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
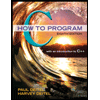
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
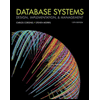
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
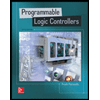
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education