Write a class called Candidate to store details of a candidate in the election. The class must define fields to store the following details: • The name of the candidate. The value will be a String which will always have a length of at least 1 character. • The number of votes the candidate has received. This is an integer value. The value will always be greater-than or equal to zero. The class must have a single constructor that takes the name of a candidate. You do not need to check that the value passed to the constructor is valid. You may assume that it always will be. In other words, you may assume that the name String will be at least one character long. The initial value of votes must always be zero when the object is constructed and it is not passed as a parameter to the constructor. The class must define the following methods: • An accessor (get) method for each field. • A method called voteFor that adds 1 to the votes field. • A method called print to print the values of the fields on a single line in exactly the following format: Dodgy Drew has 5 votes. where "Dodgy Drew" is an example of a String in the name field and 5 is an example of the current value in the votes field. Each Candidate object will have its own particular values for these fields
Write a class called Candidate to store details of a candidate in the election.
The class must define fields to store the following details:
• The name of the candidate. The value will be a String which will
always have a length of at least 1 character.
• The number of votes the candidate has received. This is an integer
value. The value will always be greater-than or equal to zero.
The class must have a single constructor that takes the name of a candidate.
You do not need to check that the value passed to the constructor is valid.
You may assume that it always will be. In other words, you may assume that
the name String will be at least one character long. The initial value of votes
must always be zero when the object is constructed and it is not passed as a
parameter to the constructor.
The class must define the following methods:
• An accessor (get) method for each field.
• A method called voteFor that adds 1 to the votes field.
• A method called print to print the values of the fields on a single line
in exactly the following format:
Dodgy Drew has 5 votes.
where "Dodgy Drew" is an example of a String in the name field and 5
is an example of the current value in the votes field. Each Candidate
object will have its own particular values for these fields

Step by step
Solved in 2 steps

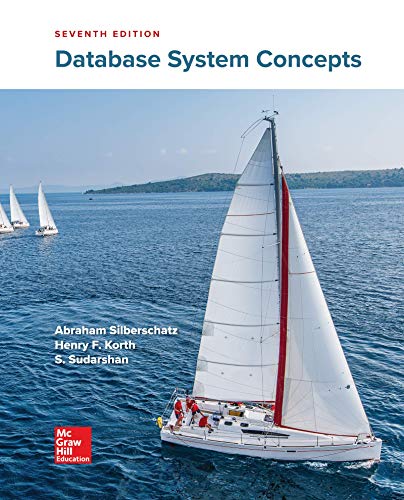
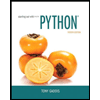
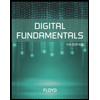
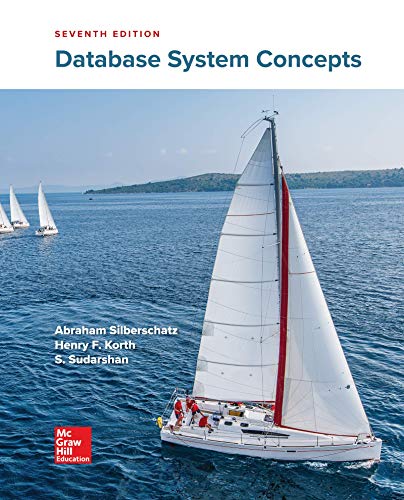
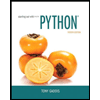
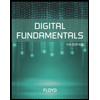
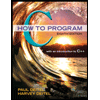
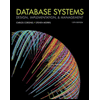
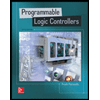