write a c++ program Write a class Date that represents a date consisting of a year, month, and day. A Date class should have the following methods: # Date(int year, int month, int day) Constructs a new Date object to represent the given date. # void add(int &days) Moves this Date object forward by the given number of days. hint:* you should decide on the basis of month and year that given month ends 30,31,28,29 days.
write a c++ program
Write a class Date that represents a date consisting of a year, month, and day. A Date class
should have the following methods:
# Date(int year, int month, int day)
Constructs a new Date object to represent the given date.
# void add(int &days)
Moves this Date object forward by the given number of days. hint:* you should decide
on the basis of month and year that given month ends 30,31,28,29 days.
# void add(int &month , int &days)
Moves this Date object forward by the given number of months and days. Months
should be within 1 to 12 and days in 1 to 31. For Example Date 2003/12/31and
add(1,29) =>Date will be 2004/02/29
# void add(Date & other)
Moves this Date object forward by the given Date.
# void addWeeks(int &weeks)
Moves this Date object forward by the given number of seven-day weeks.
# int daysTo(Date & other)
Returns the number of days that this Date must be adjusted to make it equal to the given
other Date.
# void subtract(int &days)
Moves this Date object backward by the given number of days. hint:* you should decide
on the basis of month and year that given month ends 30,31,28,29 days.
# void subtract(int &month , int &days)
Moves this Date object backward by the given number of months and days.
# void subtract (Date & other)
Moves this Date object backward by the given Date.
# int getDay()
Returns the day value of this date; for example, for the date 2006/07/22, returns 22.
# int getMonth()
Returns the month value of this date; for example, for the date
2006/07/22, returns 7.
# int getYear()
Returns the year value of this date; for example, for the date 2006/07/22, returns 2006.
# bool isLeapYear()
Returns true if the year of this date is a leap year. A leap year occurs every four years,
except for multiples of 100 that are not multiples of 400. For example, 1956, 1844,
1600, and 2000 are leap years, but 1983, 2002, 1700, and 1900 are not.
# String toString()
Returns a String representation of this date in year/month/day order, such as
"2006/07/22".

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

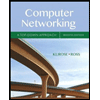
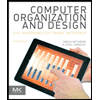
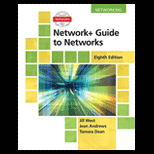
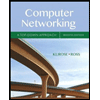
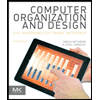
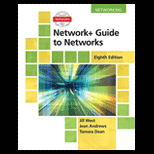
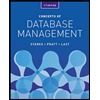
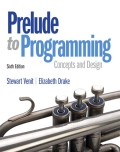
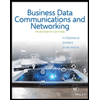