1) bagUnion: The union of two bags is a new bag containing the combined contents of the original two bags. Design and specify a method union for the ArrayBag that returns as a new bag the union of the bag receiving the call to the method and the bag that is the method's parameter. The method signature (which should appear in the .h file and be implemented in the .cpp file is: ArrayBag bagUnion(const ArrayBag &otherBag) const; This method would be called in main() with: ArrayBag bag1u2 = bag1.bagUnion(bag2); Note that the union of two bags might contain duplicate items. For example, if object x occurs five times in one bag and twice in another, the union of these bags contains x seven times. The union does not affect the contents of the original bags.
Please help me with this , I am stuck ! PLEASE WRITE IT IN C++
Thanks
1) bagUnion: The union of two bags is a new bag containing the combined contents of the original two bags. Design and specify a method union for the ArrayBag that returns as a new bag the union of the bag receiving the call to the method and the bag that is the method's parameter. The method signature (which should appear in the .h file and be implemented in the .cpp file is:
ArrayBag<T> bagUnion(const ArrayBag<T> &otherBag) const;
This method would be called in main() with:
ArrayBag<int> bag1u2 = bag1.bagUnion(bag2);
Note that the union of two bags might contain duplicate items. For example, if object x occurs five times in one bag and twice in another, the union of these bags contains x seven times. The union does not affect the contents of the original bags.
Here is the main file:
#include <cstdlib>
#include <iostream>
#include "ArrayBag.h"
using namespace std;
template <class T>
void
void displayBag(const ArrayBag<T>& bag);
/*
*
*/
int main() {
// Test using bags of integers
ArrayBag<int> bag1;
for (int i=1; i<=5; i++)
bag1.add(i);
ArrayBag<int> bag2;
bag2.add(20);
bag2.add(30);
bag2.add(40);
bag2.add(50);
bag2.add(1);
std::cout << "First bag: ";
displayBag(bag1);
std::cout << "Second bag: ";
displayBag(bag2);
// Test bagUnion, bagIntersection, bagDifference for bag1, bag2
ArrayBag<int> bag1u2 = bag1.bagUnion(bag2);
// Test using bags of strings
ArrayBag<std::string> bag3;
bag3.add("one");
bag3.add("two");
bag3.add("three");
bag3.add("four");
bag3.add("five");
ArrayBag<std::string> bag4;
bag4.add("twenty");
bag4.add("thirty");
bag4.add("fourty");
bag4.add("fifty");
bag4.add("one");
std::cout << "First bag: ";
displayBag(bag3);
std::cout << "Second bag: ";
displayBag(bag4);
// Test bagUnion, bagIntersection, bagDifference for bag3, bag4
return 0;
}
template <class T>
void displayBag(const ArrayBag<T>& bag) {
//must pass by reference
//pass by value calls copy constructor = shallow copy
cout << "The bag contains " << bag.getCurrentSize()
<< " items:" << endl;
bag.prtItems();
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

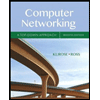
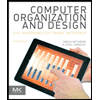
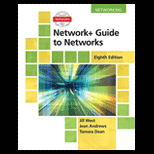
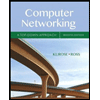
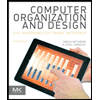
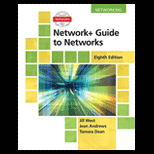
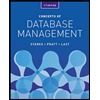
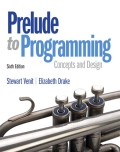
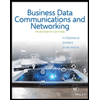