Write a C++ program that operationalizes the (awful) sort shown in the following flow chart
Write a C++ program that operationalizes the (awful) sort shown in the following flow
chart (2 images seen below, the first image to look at is the one that says start and then follow into the other image). You must use this sorting



Here, the task mentioned in the question is to write a code to implement the sorting flow chart provided. For the sake of understanding, this float chart can be converted to an algorithm as follows.
Algorithm:
1. Define three functions: Move, Findkay, and Sort.
Function Move(a, j, n):
1. Create a temporary variable 'temp' and store a[j + 1] in it.
2. Set a[j + 1] to a[j].
3. Set a[j] to 'temp'.
Function Findkay(a, j, n):
1. Initialize k to j and sw to 0.
2. While k is greater than 0 and sw is 0:
a. If a[k - 1] is greater than a[k], swap a[k - 1] and a[k], and set sw to 1.
b. Decrement k by 1.
3. Return k.
Function Sort(a, n):
1. Iterate over j from 0 to n - 2:
a. Call Findkay(a, j, n) and store the result in k.
b. If k is not equal to j, call Move(a, j, n).
2. In the main function:
1. Read the number of elements 'n' from the user.
2. Create an array 'a' of size 'n' and read the elements from the user.
3. Call Sort(a, n) to sort the array using the given algorithm.
4. Print the sorted array.
3. End of the algorithm.
Step by step
Solved in 4 steps with 2 images

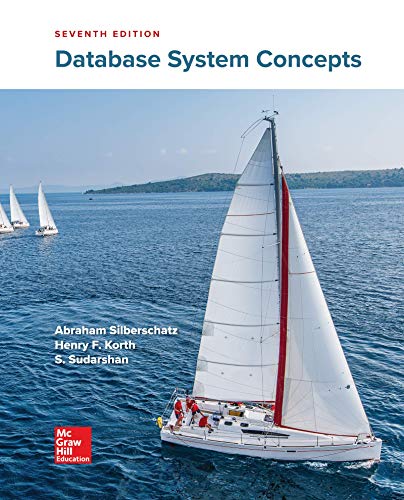
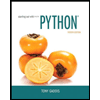
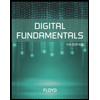
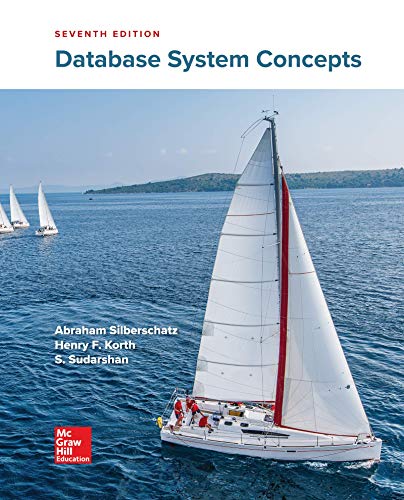
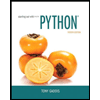
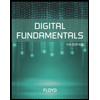
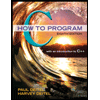
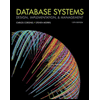
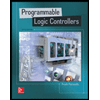